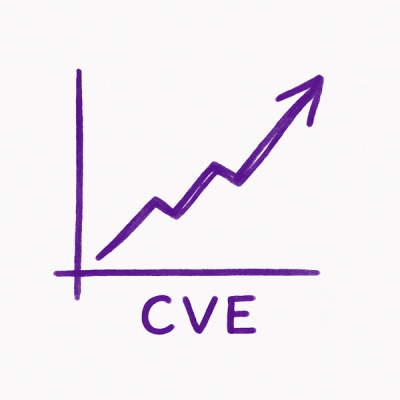
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
An approach to aspect-oriented programming (AOP) in javascript for both node.js and browser.
There are different use cases where AOP comes in handy, cases like logging, caching, testing, debugging, before/after filters and so on. filter.js
provides an elegant approach to AOP in javascript.
npm install filter.js
Filter = require('filter.js');
// Every call to object.method will be wrapped by our filter
// Multiple filters can be applied to a single method
var f = new Filter(object, 'method', function(args, next) {
// do something with the args
var result = next(args);
// do something with the result
return result;
});
// Temporary disable filter
f.off();
// Activate a disabled filter
f.on();
// Premanently remove filter
f.remove();
Here is an example usage of filter.js to log and cache mysql queries:
var Filter = require('filter.js');
var mysql = require('mysql');
var connection = mysql.createConnection({
host : 'localhost',
user : 'user',
password : 'secret',
database : 'mydb'
});
var cache = {};
//Log queries
new Filter(connection, 'query', function(args, next) {
var query = args[0];
var callback = args[1];
var start = Date.now();
args[1] = function(err, rows) {
console.log(query);
console.log('Took ' + (Date.now() - start) + ' ms');
callback(err, rows);
};
return next(args);
});
// Cache SELECT queries
new Filter(connection, 'query', function(args, next) {
var query = args[0];
var callback = args[1];
// Cache only SELECT queries
if (!query.match(/^SELECT/i)) {
return next(args);
}
//Check if we already have cached result in memory
if (cache[query]) {
// skip executing the query
callback(null, cache[query]);
return;
}
args[1] = function(err, rows) {
cache[query] = rows;
callback(err, rows);
};
return next(args);
});
connection.query('SELECT * FROM posts', function(err, rows) {
console.log(rows);
});
filter.js is released under the MIT license.
The idea is inspired by li3 filter system.
FAQs
An approach to aspect-oriented programming (AOP) in javascript
The npm package filter.js receives a total of 5 weekly downloads. As such, filter.js popularity was classified as not popular.
We found that filter.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.