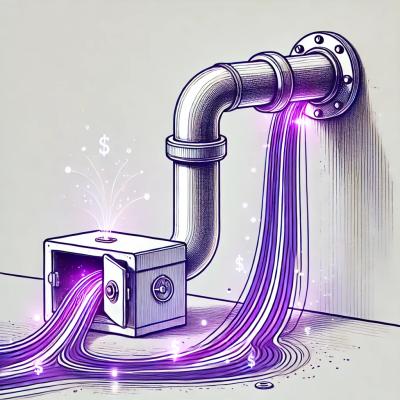
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
find-requires
Advanced tools
Find all require() calls. Fast and solid implementation backed with direct scanner and esprima AST parser
The find-requires npm package is a tool for analyzing JavaScript code to find and list all the require statements. It is useful for understanding dependencies in a codebase, refactoring, or simply auditing the use of modules.
Find all require statements in a file
This feature allows you to find all the require statements in a given JavaScript file. The code reads the content of a file and uses the find-requires package to extract all the require statements.
const findRequires = require('find-requires');
const fs = require('fs');
const fileContent = fs.readFileSync('path/to/your/file.js', 'utf8');
const requires = findRequires(fileContent);
console.log(requires);
Find require statements with options
This feature allows you to find require statements with additional options. For example, setting the 'raw' option to true will return the raw AST nodes instead of just the module names.
const findRequires = require('find-requires');
const fs = require('fs');
const fileContent = fs.readFileSync('path/to/your/file.js', 'utf8');
const options = { raw: true };
const requires = findRequires(fileContent, options);
console.log(requires);
The detective package is a similar tool that parses JavaScript code to find all the require statements. It is highly configurable and can be used with various parsers. Compared to find-requires, detective offers more flexibility in terms of parser options and supports ES6 import statements.
Precinct is another package that can find dependencies in JavaScript code, including both require and import statements. It supports multiple file types and can be extended with custom parsers. Precinct provides a more comprehensive solution for dependency detection compared to find-requires.
Made for modules-webmake. Fast esniff based implementation of require calls parser.
foo.js:
var one = require("one");
var two = require("two");
var slp = require("some/long" + "/path");
var wrong = require(cannotTakeThat);
program.js:
var fs = require("fs");
var findRequires = require("find-requires");
var src = fs.readFileSync("foo.js", "utf-8");
console.log(findRequires(src)); // => ['one', 'two', 'some/long/path'];
// or we can get more detailed data with `raw` option:
console.log(findRequires(src, { raw: true })); /* => [
{ value: 'one', raw: '\'one\'', point: 19, line: 1, column: 19 },
{ value: 'two', raw: '\'two\'', point: 45, line: 2, column: 19 },
{ value: 'some/long/path', raw: '\'some/long\' +\n\t\t\t\t\t\t\'/path\'',
point: 71, line: 3, column: 19 },
{ raw: 'cannotTakeThat', point: 121, line: 5, column: 21 }
] */
// We can also ensure some specific cases of dynamic requires code with some setup code injection
console.log(
findRequires("require(__dirname + '/foo.js')", {
setupCode: `const __dirname = ${ JSON.stringify(__dirname) }`
})
);
> npm install -g find-requires
Find all requires in a file:
> find-requires file1.js
test1.js:3:LIB + '/test2'
test1.js:4:fs
Find all places the fs module is required: find-requires -m fs $(find . -name '*.js')
$ npm test
FAQs
Find all require() calls. Fast and solid implementation backed with direct scanner and esprima AST parser
The npm package find-requires receives a total of 689,152 weekly downloads. As such, find-requires popularity was classified as popular.
We found that find-requires demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.