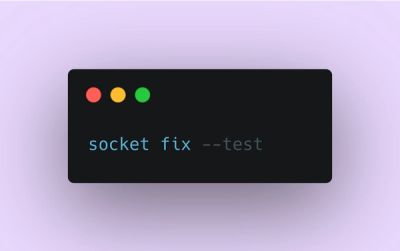
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
fortysixelks-node
Advanced tools
A node.js wrapper of the 46elks API (http://46elks.com). Currently supports sending sms/text messages, flash sms, allocate numbers, deallocate/inactivet numbers, create subaccounts.
npm install fortysixelks-node
Everything hangs off a Client object:
var client = require('fortysixelks-node')('<username>', '<password>');
Using promises
var client = require('fortysixelks-node')('<username>', '<password>');
client.sendSms('Test ' + new Date(), '+461234567', '+461234567')
.then(function(res){
console.log(res);
});
Using callbacks
var client = require('fortysixelks-node')('<username>', '<password>');
client.sendSms('Test' + new Date(), '+46123456', '+46123456', function(err, res){
if(err) console.log(':(');
console.log(res);
});
Response format (JSON)
{
direction: 'outgoing',
from: '+46123456',
created: '2015-11-11T14:10:38.496470',
to: '+467123345',
cost: 3500,
message: 'Test from fortysixelks-node Wed Nov 11 2015 15:10:38 GMT+0100 (CET)',
id: 's1bd965cc067fcaba9b09c128a555c815'
}
Using Promises
var client = require('fortysixelks-node')('<username>', '<password>');
client.listSms().then(function(res) { console.log(res); });
Using Callbacks
var client = require('fortysixelks-node')('<username>', '<password>');
client.listSms(function(err, res){
console.log(res);
})
Response format
{ data:
[ { status: 'delivered',
delivered: '2015-11-11T14:10:40.854000',
direction: 'outgoing',
from: '+46123456',
created: '2015-11-11T14:10:38.496000',
to: '+46123456',
cost: 3500,
message: 'NONON Wed Nov 11 2015 15:10:38 GMT+0100 (CET)',
id: 's1bd965cc067fcaba9b09c128a555c801' },
{ status: 'delivered',
delivered: '2015-11-09T12:05:31.454000',
direction: 'outgoing',
from: '+46123456',
created: '2015-11-09T12:05:28.128000',
to: '+46123456',
cost: 3500,
message: 'NONON Mon Nov 09 2015 13:05:28 GMT+0100 (CET)',
id: 'sbb8f7da6dd8830b2ee0db4694fd27fe8' },
{ status: 'delivered',
delivered: '2015-11-09T11:29:35.322000',
direction: 'outgoing',
from: 'tk',
created: '2015-11-09T11:29:33.042000',
to: '+46123456',
cost: 3500,
message: 'Hoho',
id: 'sd334f449791a00f1352df8d22519b52a' },
{ status: 'delivered',
delivered: '2015-11-09T06:48:02.040000',
direction: 'outgoing',
from: '+46123456',
created: '2015-11-09T06:47:58.990000',
to: '+46123456',
cost: 3500,
message: 'testing...',
id: 'sf0a416264bbd36f507d047c877849c04'
} ]
}
Using Promises
var client = require('fortysixelks-node')('<username>', '<password>');
client.listSmsFrom('2015-11-09T06:47:58.990000').then(function(res) { console.log(res); });
Using Callbacks
var client = require('fortysixelks-node')('<username>', '<password>');
client.listSmsFrom('2015-11-09T06:47:58.990000', function(err, res){
console.log(res);
})
Using Promises
var client = require('fortysixelks-node')('<username>', '<password>');
client.sendFlashSms('PIN. 123456', '+46123456', '+46123456').then(function(res){ console.log(res);});
Callback
var client = require('fortysixelks-node')('<username>', '<password>');
client.sendFlashSms('PIN: 123456', '+46123456', '+46123456', function(err, res){
if(err) console.error(':( Error! ' + err.message);
console.log(res);
});
Response
{
direction: 'outgoing',
from: '+46123456',
created: '2015-11-11T20:06:18.251418',
flashsms: 'yes',
to: '+467123456',
cost: 3500,
message: 'PIN: 123456',
id: 's68c992f33df7903ffbfd721926fe7207'
}
The url that gets called when 46elks gets a incoming sms to your allocated number. Using Promises
var client = require('fortysixelks-node')('<username>', '<password>');
client.setSmsUrl('nd51066f1746006c917aebb9sdfdsde58', 'http://hardcoded.se/incoming_sms')
.then(function(res) {console.dir(res);});
Callback
var client = require('fortysixelks-node')('<username>', '<password>');
client.setSmsUrl('nd51066f1746006c917aebb9sdfdsde58', 'http://hardcoded.se/incoming_sms', function(err, res) {
if(err) console.error(':( Error! ' + err.message);
console.log(res);
});
Response
{
category: 'mobile',
country: 'se',
expires: '2015-12-12T14:56:07',
number: '+46123456',
capabilities: [ 'sms', 'voice' ],
active: 'yes',
sms_url: 'http://hardcoded.se/incoming_sms',
id: 'nd51066f1746006c917aebb9sdfdsde58'
}
I've added functions for MMS but they are not tested, I did not have a MMS enabled number and could not find in the docs how-to send an MMS.
var client = require('fortysixelks-node')('<username>', '<password>');
client.listNumbers().then(function(res) {
console.log(res);
});
Response
{
"data": [
{
"category": "mobile",
"country": "se",
"expires": "2015-12-12T14:56:07",
"number": "+46123456",
"capabilities": [
"sms",
"voice"
],
"active": "yes",
"sms_url": "http://hardcoded.se/sms_hook",
"id": "nd510a4a4a446006c917aebb91dc506e58"
},
{
"category": "mobile",
"country": "se",
"number": "+46123456",
"capabilities": [
"sms",
"voice"
],
"active": "no",
"sms_url": "http://hardcoded.se/sms_hook",
"id": "n420baa4a40e29c48282ec3415bdacaa5"
}
]
}
var client = require('fortysixelks-node')('<username>', '<password>');
client.getNumberDetail('nd510a4a4a446006c917aebb91dc506e58').then(function(res) {
console.log(res);
});
Response
{
"category": "mobile",
"country": "se",
"expires": "2015-12-12T14:56:07",
"number": "+46123456",
"capabilities": [
"sms",
"voice"
],
"active": "yes",
"sms_url": "http://hardcoded.se/sms_hook",
"id": "nd510a4a4a446006c917aebb91dc506e58"
}
var client = require('fortysixelks-node')('<username>', '<password>');
client.allocateNewNumber('country (se)', 'sms url', 'mms url', 'voice start url').then(function(res) {
console.log(res);
});
Only country is mandatory!
Response
{
"category": "mobile",
"country": "se",
"expires": "2015-12-12T14:56:07",
"number": "+46123456",
"capabilities": [
"sms",
"voice"
],
"active": "yes",
"sms_url": "http://hardcoded.se/sms_hook",
"id": "nd510a4a4a446006c917aebb91dc506e58"
}
Reminder: You cannot get a deactivated number back.
var client = require('fortysixelks-node')('<username>', '<password>');
client.deallocateNumber('nd510a4a4a446006c917aeb1vvdc506e58').then(function(res) {
console.log(res);
});
Only country is mandatory!
Response
{
"category": "mobile",
"country": "se",
"expires": "2015-12-12T14:56:07",
"number": "+46123456",
"capabilities": [
"sms",
"voice"
],
"active": "no",
"sms_url": "http://hardcoded.se/sms_hook",
"id": "nd510a4a4a446006c917aeb1vvdc506e58"
}
Coming...
These names end you up in the same place.
Method name | Other method name |
---|---|
sendSms | sendMessage |
listSms | listMessages |
FAQs
A node.js wrapper of the 46elks API (http://46elks.com)
We found that fortysixelks-node demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.