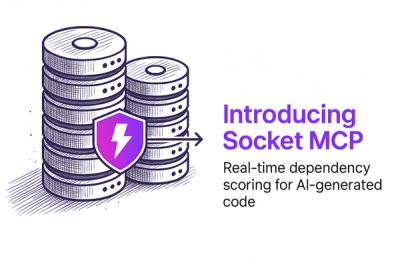
Product
Secure Your AI-Generated Code with Socket MCP
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
fritz-callmonitor
Advanced tools
Provides a TypeScript Node.js wrapper for the call monitor api of the AVM Fritz!Box.
Provides a Node.js wrapper for the call monitor api of the AVM Fritz!Box. Written in TypeScript.
npm install fritz-callmonitor
You need TypeScript 2. Just install the NPM package and you're ready to go!
The network API is disabled by default. To use this, call #96*5*
on a phone which is managed by the FRITZ!Box.
"use strict";
import { CallMonitor, EventKind } from "fritz-callmonitor";
const cm = new CallMonitor("192.168.178.1", 1012);
cm.on("ring", rr => {
console.dir(rr);
console.log(`${rr.caller} calling...`);
});
cm.on("call", rr => console.dir(rr));
cm.on("pickup", rr => console.dir(rr));
cm.on("hangup", rr => console.dir(rr));
cm.on("phone", evt => {
// gets called on every phone event
switch(evt.kind) {
case EventKind.Ring:
case EventKind.Call:
console.log(`${evt.caller} -> ${evt.callee}`);
break;
}
});
cm.on("close", () => console.log("Connection closed."));
cm.on("connect", () => console.log("Connected to device."));
cm.on("error", err => console.dir(err));
cm.connect();
FAQs
Provides a TypeScript Node.js wrapper for the call monitor api of the AVM Fritz!Box.
The npm package fritz-callmonitor receives a total of 7 weekly downloads. As such, fritz-callmonitor popularity was classified as not popular.
We found that fritz-callmonitor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
Security News
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
Research
Security News
Socket’s Threat Research Team has uncovered 60 npm packages using post-install scripts to silently exfiltrate hostnames, IP addresses, DNS servers, and user directories to a Discord-controlled endpoint.