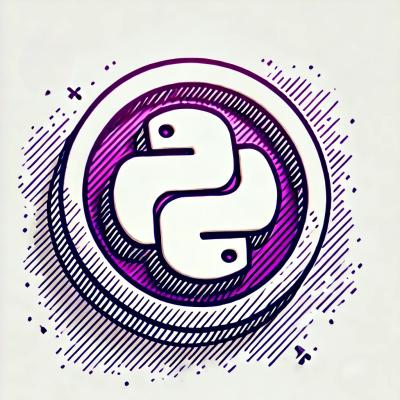
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
eval
, don't parse untrusted JSON!Implementation of reviver and replacer functions for JSON parser to stringify, detect and parse methods as strings. Works in browser and node, no dependencies.
In a browser just load a script:
<script src="https://cdn.jsdelivr.net/npm/funjson/browser/funJSON.min.js"></script>
Using npm:
npm i --save funjson
const funJSON = require('funjson');
or
import { stringify, stringifyToScript, parse } from 'funjson';
var obj = {
a:{
a1:'a1',
fa:function(name){
//some comment here
return 'fa Hello ' + name;
}
},
b:{
b1:'b1'
},
f:(name) => {
//some comment here
return 'f Hello ' + name;
}
};
var str = funJSON.stringify(obj,null,2);// same syntax as for JSON.stringify
console.log('JSON:',str);
var obj2 = funJSON.parse(str);// same syntax as for JSON.parse
obj2.f('obj'); // --> 'f Hello obj'
obj2.a.fa('obj.a'); // --> 'fa Hello obj.a'
str = funJSON.stringifyToScript(obj,null,2);// generate JavaScript string.
//Useful to let user edit it in some code editor.
console.log('JS:',str);
eval('obj2 = '+str);
obj2.f("obj"); // --> 'f Hello obj'
obj2.a.fa("obj.a"); // --> 'fa Hello obj.a'
Any feedback is welcome.
FAQs
JSON with functions (methods)
The npm package funjson receives a total of 0 weekly downloads. As such, funjson popularity was classified as not popular.
We found that funjson demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.