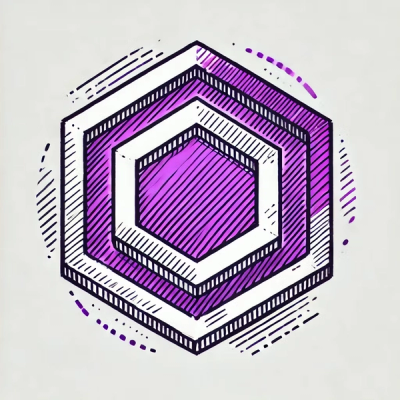
Security News
ESLint Adds Official Support for Linting HTML
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.
Note: The gdax
package is deprecated and might have to be removed from NPM.
Please migrate to the coinbase-pro
package to ensure future compatibility.
The official Node.js library for Coinbase's Pro API.
npm install coinbase-pro
You can learn about the API responses of each endpoint by reading our documentation.
The Coinbase Pro API has both public and private endpoints. If you're only interested in
the public endpoints, you should use a PublicClient
.
const CoinbasePro = require('coinbase-pro');
const publicClient = new CoinbasePro.PublicClient();
All methods, unless otherwise specified, can be used with either a promise or callback API.
publicClient
.getProducts()
.then(data => {
// work with data
})
.catch(error => {
// handle the error
});
The promise API can be used as expected in async
functions in ES2017+
environments:
async function yourFunction() {
try {
const products = await publicClient.getProducts();
} catch (error) {
/* ... */
}
}
Your callback should accept three arguments:
error
: contains an error message (string
), or null
if no error was
encounteredresponse
: a generic HTTP response abstraction created by the request
librarydata
: contains data returned by the Coinbase Pro API, or undefined
if an error was
encounteredpublicClient.getProducts((error, response, data) => {
if (error) {
// handle the error
} else {
// work with data
}
});
NOTE: if you supply a callback, no promise will be returned. This is to
prevent potential UnhandledPromiseRejectionWarning
s, which will cause future
versions of Node to terminate.
const myCallback = (err, response, data) => {
/* ... */
};
const result = publicClient.getProducts(myCallback);
result.then(() => {
/* ... */
}); // TypeError: Cannot read property 'then' of undefined
Some methods accept optional parameters, e.g.
publicClient.getProductOrderBook('BTC-USD', { level: 3 }).then(book => {
/* ... */
});
To use optional parameters with callbacks, supply the options as the first parameter(s) and the callback as the last parameter:
publicClient.getProductOrderBook(
'ETH-USD',
{ level: 3 },
(error, response, book) => {
/* ... */
}
);
const publicClient = new CoinbasePro.PublicClient(endpoint);
productID
optional - defaults to 'BTC-USD' if not specified.endpoint
optional - defaults to 'https://api.pro.coinbase.com' if not specified.publicClient.getProducts(callback);
// Get the order book at the default level of detail.
publicClient.getProductOrderBook('BTC-USD', callback);
// Get the order book at a specific level of detail.
publicClient.getProductOrderBook('LTC-USD', { level: 3 }, callback);
publicClient.getProductTicker('ETH-USD', callback);
publicClient.getProductTrades('BTC-USD', callback);
// To make paginated requests, include page parameters
publicClient.getProductTrades('BTC-USD', { after: 1000 }, callback);
Wraps around getProductTrades
, fetches all trades with IDs >= tradesFrom
and
<= tradesTo
. Handles pagination and rate limits.
const trades = publicClient.getProductTradeStream('BTC-USD', 8408000, 8409000);
// tradesTo can also be a function
const trades = publicClient.getProductTradeStream(
'BTC-USD',
8408000,
trade => Date.parse(trade.time) >= 1463068e6
);
publicClient.getProductHistoricRates('BTC-USD', callback);
// To include extra parameters:
publicClient.getProductHistoricRates(
'BTC-USD',
{ granularity: 3600 },
callback
);
publicClient.getProduct24HrStats('BTC-USD', callback);
publicClient.getCurrencies(callback);
publicClient.getTime(callback);
The private exchange API endpoints require you
to authenticate with a Coinbase Pro API key. You can create a new API key in your
exchange account's settings. You can also specify
the API URI (defaults to https://api.pro.coinbase.com
).
const key = 'your_api_key';
const secret = 'your_b64_secret';
const passphrase = 'your_passphrase';
const apiURI = 'https://api.pro.coinbase.com';
const sandboxURI = 'https://api-public.sandbox.pro.coinbase.com';
const authedClient = new CoinbasePro.AuthenticatedClient(
key,
secret,
passphrase,
apiURI
);
Like PublicClient
, all API methods can be used with either callbacks or will
return promises.
AuthenticatedClient
inherits all of the API methods from
PublicClient
, so if you're hitting both public and private API endpoints you
only need to create a single client.
authedClient.getCoinbaseAccounts(callback);
authedClient.getPaymentMethods(callback);
authedClient.getAccounts(callback);
const accountID = '7d0f7d8e-dd34-4d9c-a846-06f431c381ba';
authedClient.getAccount(accountID, callback);
const accountID = '7d0f7d8e-dd34-4d9c-a846-06f431c381ba';
authedClient.getAccountHistory(accountID, callback);
// For pagination, you can include extra page arguments
authedClient.getAccountHistory(accountID, { before: 3000 }, callback);
const accountID = '7d0f7d8e-dd34-4d9c-a846-06f431c381ba';
authedClient.getAccountTransfers(accountID, callback);
// For pagination, you can include extra page arguments
authedClient.getAccountTransfers(accountID, { before: 3000 }, callback);
const accountID = '7d0f7d8e-dd34-4d9c-a846-06f431c381ba';
authedClient.getAccountHolds(accountID, callback);
// For pagination, you can include extra page arguments
authedClient.getAccountHolds(accountID, { before: 3000 }, callback);
// Buy 1 BTC @ 100 USD
const buyParams = {
price: '100.00', // USD
size: '1', // BTC
product_id: 'BTC-USD',
};
authedClient.buy(buyParams, callback);
// Sell 1 BTC @ 110 USD
const sellParams = {
price: '110.00', // USD
size: '1', // BTC
product_id: 'BTC-USD',
};
authedClient.sell(sellParams, callback);
// Buy 1 LTC @ 75 USD
const params = {
side: 'buy',
price: '75.00', // USD
size: '1', // LTC
product_id: 'LTC-USD',
};
authedClient.placeOrder(params, callback);
const orderID = 'd50ec984-77a8-460a-b958-66f114b0de9b';
authedClient.cancelOrder(orderID, callback);
// Cancels "open" orders
authedClient.cancelOrders(callback);
// `cancelOrders` may require you to make the request multiple times until
// all of the "open" orders are deleted.
// `cancelAllOrders` will handle making these requests for you asynchronously.
// Also, you can add a `product_id` param to only delete orders of that product.
// The data will be an array of the order IDs of all orders which were cancelled
authedClient.cancelAllOrders({ product_id: 'BTC-USD' }, callback);
authedClient.getOrders(callback);
// For pagination, you can include extra page arguments
// Get all orders of 'open' status
authedClient.getOrders({ after: 3000, status: 'open' }, callback);
const orderID = 'd50ec984-77a8-460a-b958-66f114b0de9b';
authedClient.getOrder(orderID, callback);
const params = {
product_id: 'LTC-USD',
};
authedClient.getFills(params, callback);
// For pagination, you can include extra page arguments
authedClient.getFills({ before: 3000 }, callback);
authedClient.getFundings({}, callback);
const params = {
amount: '2000.00',
currency: 'USD',
};
authedClient.repay(params, callback);
const params =
'margin_profile_id': '45fa9e3b-00ba-4631-b907-8a98cbdf21be',
'type': 'deposit',
'currency': 'USD',
'amount': 2
};
authedClient.marginTransfer(params, callback);
const params = {
repay_only: false,
};
authedClient.closePosition(params, callback);
const params = {
from: 'USD',
to: 'USDC',
amount: '100',
};
authedClient.convert(params, callback);
// Deposit to your Exchange USD account from your Coinbase USD account.
const depositParamsUSD = {
amount: '100.00',
currency: 'USD',
coinbase_account_id: '60680c98bfe96c2601f27e9c', // USD Coinbase Account ID
};
authedClient.deposit(depositParamsUSD, callback);
// Withdraw from your Exchange USD account to your Coinbase USD account.
const withdrawParamsUSD = {
amount: '100.00',
currency: 'USD',
coinbase_account_id: '60680c98bfe96c2601f27e9c', // USD Coinbase Account ID
};
authedClient.withdraw(withdrawParamsUSD, callback);
// Deposit to your Exchange BTC account from your Coinbase BTC account.
const depositParamsBTC = {
amount: '2.0',
currency: 'BTC',
coinbase_account_id: '536a541fa9393bb3c7000023', // BTC Coinbase Account ID
};
authedClient.deposit(depositParamsBTC, callback);
// Withdraw from your Exchange BTC account to your Coinbase BTC account.
const withdrawParamsBTC = {
amount: '2.0',
currency: 'BTC',
coinbase_account_id: '536a541fa9393bb3c7000023', // BTC Coinbase Account ID
};
authedClient.withdraw(withdrawParamsBTC, callback);
// Fetch a deposit address from your Exchange BTC account.
const depositAddressParams = {
currency: 'BTC',
};
authedClient.depositCrypto(depositAddressParams, callback);
// Withdraw from your Exchange BTC account to another BTC address.
const withdrawAddressParams = {
amount: 10.0,
currency: 'BTC',
crypto_address: '15USXR6S4DhSWVHUxXRCuTkD1SA6qAdy',
};
authedClient.withdrawCrypto(withdrawAddressParams, callback);
// Schedule Deposit to your Exchange USD account from a configured payment method.
const depositPaymentParamsUSD = {
amount: '100.00',
currency: 'USD',
payment_method_id: 'bc6d7162-d984-5ffa-963c-a493b1c1370b', // ach_bank_account
};
authedClient.depositPayment(depositPaymentParamsUSD, callback);
// Withdraw from your Exchange USD account to a configured payment method.
const withdrawPaymentParamsUSD = {
amount: '100.00',
currency: 'USD',
payment_method_id: 'bc6d7162-d984-5ffa-963c-a493b1c1370b', // ach_bank_account
};
authedClient.withdrawPayment(withdrawPaymentParamsUSD, callback);
// Get your 30 day trailing volumes
authedClient.getTrailingVolume(callback);
The WebsocketClient
allows you to connect and listen to the exchange
websocket messages.
const websocket = new CoinbasePro.WebsocketClient(['BTC-USD', 'ETH-USD']);
websocket.on('message', data => {
/* work with data */
});
websocket.on('error', err => {
/* handle error */
});
websocket.on('close', () => {
/* ... */
});
The client will automatically subscribe to the heartbeat
channel. By
default, the full
channel will be subscribed to unless other channels are
requested.
const websocket = new CoinbasePro.WebsocketClient(
['BTC-USD', 'ETH-USD'],
'wss://ws-feed-public.sandbox.pro.coinbase.com',
{
key: 'suchkey',
secret: 'suchsecret',
passphrase: 'muchpassphrase',
},
{ channels: ['full', 'level2'] }
);
Optionally, change subscriptions at runtime:
websocket.unsubscribe({ channels: ['full'] });
websocket.subscribe({ product_ids: ['LTC-USD'], channels: ['ticker', 'user'] });
websocket.subscribe({
channels: [
{
name: 'user',
product_ids: ['ETH-USD'],
},
],
});
websocket.unsubscribe({
channels: [
{
name: 'user',
product_ids: ['LTC-USD'],
},
{
name: 'user',
product_ids: ['ETH-USD'],
},
],
});
The following events can be emitted from the WebsocketClient
:
open
message
close
error
Orderbook
is a data structure that can be used to store a local copy of the
orderbook.
const orderbook = new CoinbasePro.Orderbook();
The orderbook has the following methods:
state(book)
get(orderId)
add(order)
remove(orderId)
match(match)
change(change)
OrderbookSync
creates a local mirror of the orderbook on Coinbase Pro using
Orderbook
and WebsocketClient
as described
here.
const orderbookSync = new CoinbasePro.OrderbookSync(['BTC-USD', 'ETH-USD']);
console.log(orderbookSync.books['ETH-USD'].state());
npm test
# test for known vulnerabilities in packages
npm install -g nsp
nsp check --output summary
FAQs
Client for the GDAX API
The npm package gdax receives a total of 77 weekly downloads. As such, gdax popularity was classified as not popular.
We found that gdax demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.