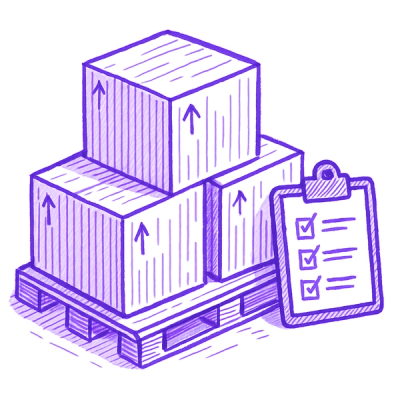
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
node.js bindings to the Genx XML generation library.
npm install genx
There are a few ways to build this project:
npm install
in the node-genx root directory should automatically trigger a build. You
should do this at least once regardless of which build method you wind up using since there are
some necessary dependencies npm must install before compilation.node-gyp configure build
mkdir -p build
cd build
cmake ..
make
command to be present:
npm run-script clean && npm run-script cmake
npm run-script clean && npm run-script cmake-debug
The following complete example uses Genx to reproduce the brief, single-entry Atom Feed Document in the Atom spec. The result is written to stdout.
var genx = require('genx');
// Passing "true" to the constructor indicates we want to nicely format the output
var w = new genx.Writer(true);
w.on('data', function(data) {
process.stdout.write(data);
});
// Declare the elements and attributes up-front
var ns = w.declareNamespace('http://www.w3.org/2005/Atom', '');
var feed = w.declareElement(ns, 'feed');
var title = w.declareElement(ns, 'title');
var link = w.declareElement(ns, 'link');
var updated = w.declareElement(ns, 'updated');
var author = w.declareElement(ns, 'author');
var name = w.declareElement(ns, 'name');
var id = w.declareElement(ns, 'id');
var entry = w.declareElement(ns, 'entry');
var summary = w.declareElement(ns, 'summary');
var href = w.declareAttribute('href');
// This is not a processing instruction and as such can't be generated by Genx
process.stdout.write("<?xml version=\"1.0\" encoding=\"utf-8\"?>\n");
w.startDocument()
.startElement(feed)
.startElement(title).addText("Example Feed").endElement()
.startElement(link).addAttribute(href, "http://example.org/").endElement()
.startElement(updated).addText("2003-12-13T18:30:02Z").endElement()
.startElement(author)
.startElement(name).addText("John Doe").endElement()
.endElement()
.startElement(id).addText("urn:uuid:60a76c80-d399-11d9-b93C-0003939e0af6").endElement()
.startElement(entry)
.startElement(title).addText("Atom-Powered Robots Run Amok").endElement()
.startElement(link).addAttribute(href, "http://example.org/2003/12/13/atom03").endElementInline()
.startElement(id).addText("urn:uuid:1225c695-cfb8-4ebb-aaaa-80da344efa6a").endElement()
.startElement(updated).addText("2003-12-13T18:30:02Z").endElement()
.startElement(summary).addText("Some text.").endElement()
.endElement()
.endElement()
.endDocument();
To run the above example:
node examples/atom.js
For more examples see, Generating XML With node.js.
The API pretty closely follows the underlying Genx library's API.
This module exports one object, Writer
, which you use to generate XML. Any
errors encountered are raised as exceptions.
Note: Each of the following examples assumes the module has been imported
and a Writer
created as follows:
var genx = require('genx');
var writer = new genx.Writer();
The Writer
emits data events with a single string argument containing an XML
fragment. You listen for data events in order to make use of the generated XML.
writer.on('data', function(data) {
// Do something with the data such as write it to a file
});
For more information on how to use streams, consult the Node Streams API documentation.
The Writer class provides the primary interface to Genx. Call writer methods to
generate XML. XML can be generated via literal nodes (elements, attributes) or
by reusing pre-declared nodes. The Genx documentation claims that using
predeclared nodes are more efficient. Where it makes sense the methods return
this
allowing calls to be chained. For example:
writer.startDocument().startElement(elem);
Constructs a new Writer object.
Arguments
false
\n
\t
Return Value
Returns the created Writer
Example
// No output formatting
var writer = new genx.Writer();
// PrettyPrint with the default \n newlines and \t to indent
var writer = new genx.Writer(true);
// PrettyPrint with \r\n newlines and four spaces to indent
var writer = new genx.Writer(true, "\r\n", " ");
Starts an XML document. Must be called before any elements can be added. This
method may be called on a Writer
multiple times after completing each
document with endDocument
in order to re-use a Writer
and generate multiple
documents.
Return Value
Returns the receiver.
Example
writer.startDocument();
Ends a document previously started with startDocument
. Must be called after
startDocument
and after any open elements have been closed.
Return Value
Returns the receiver.
Example
writer.startDocument().endDocument();
Declares a namespace for later use in declareElement
.
Arguments
""
, then this namespace will be set as the default
namespace.Return Value
Returns a Namespace
object for later use with declareElement
.
Examples
// Namespace with prefix
var ns = writer.declareNamespace('http://www.w3.org/2005/Atom', "atom");
// Default namespace
var ns = writer.declareNamespace('http://www.w3.org/2005/Atom', "");
// Generated prefix
var ns = writer.declareNamespace('http://www.w3.org/2005/Atom');
Declares an element with name name
in namespace namespace
. If
no namespace is supplied then the element is in no namespace.
Arguments
Namespace
object returned by declareNamespace
.Return Value
Returns an Element
object for later use with startElement
.
Examples
// Element without a namespace
var elem = writer.declareElement('test');
// Namespaced element
var ns = writer.declareNamespace('http://www.w3.org/2005/Atom', "");
var elem = writer.declareElement(ns, 'feed');
Declares an attribute with name name
in namespace namespace
. If no
namespace is supplied then the attribute is in no namespace.
Arguments
Namespace
object returned by declareNamespace
.addAttribute
.Return Value
Returns an Attribute
object for later use with addAttribute
.
Examples
// Attribute without a namespace
var elem = writer.declareElement('type');
// Namespaced attribute
var ns = writer.declareAttribute('http://www.w3.org/2005/Atom', "");
var elem = writer.declareAttribute(ns, 'type');
Opens the element element
.
Arguments
Element
object previously declared via declareElement
.Return Value
Returns the receiver.
Example
var elem = writer.declareElement('feed');
writer.startDocument()
.startElement(elem)
.endElement()
.endDocument()
Opens an element with name, name
in namespace namespace
(a URI) without
pre-declaring it. The Genx documentation claims that pre-declaring is more
efficient, especially if the element is emitted multiple times.
Arguments
declareNamespace
then that prefix will be used, otherwise Genx will
generate one of the form described in declareNamespace
.Return Value
Returns the receiver.
Examples
// Without a namespace
writer.startDocument()
.startElementLiteral('feed')
.endElement()
.endDocument()
// With a namespace
writer.startDocument()
.startElementLiteral('http://www.w3.org/2005/Atom', 'feed')
.endElement()
.endDocument()
Adds a text node to the document.
Arguments
Return Value
Returns the receiver.
Example
writer.startDocument()
.startElementLiteral('feed')
.addText("Some text")
.endElement()
.endDocument()
Arguments
Return Value
Returns the receiver.
Example
writer.startDocument()
.addComment("Generated " + (new Date()).toString())
.startElementLiteral('feed')
.endElement()
.endDocument();
Arguments
Attribute
object previously declared via declareAttribute
.Return Value
Returns the receiver.
Example
var ns = writer.declareNamespace('http://www.w3.org/2005/Atom', '');
var feed = writer.declareElement(ns, 'feed');
var title = writer.declareElement(ns, 'title');
var type = writer.declareAttribute('type');
writer.startDocument()
.startElement(feed)
.startElement(title)
.addAttribute(type, 'text')
.addText("Test Title")
.endElement()
.endElement()
.endDocument();
Arguments
declareNamespace
then that prefix will be used, otherwise Genx will
generate one of the form described in declareNamespace
.Return Value
Returns the receiver.
Example
var ns = writer.declareNamespace('http://www.w3.org/2005/Atom', '');
var feed = writer.declareElement(ns, 'feed');
var title = writer.declareElement(ns, 'title');
writer.startDocument()
.startElement(feed)
.startElement(title)
.addAttributeLiteral('type', 'text')
.addText("Test Title")
.endElement()
.endElement()
.endDocument();
Return Value
Returns the receiver.
Example
writer.startDocument()
.startElementLiteral('feed')
.endElement()
.endDocument()
Ends an element as an inline / "self-closing" tag. The element must only contain attributes, or an exception is thrown.
Return Value
Returns the receiver.
Example
writer.startDocument()
.startElementLiteral('feed')
.addAttributeLiteral('an-attribute', 'attribute-value')
.endElementInline()
.endDocument()
This project has a test suite in the test
directory. It utilises the
Mocha test framework. To run the suite you need to have the
mocha
and should
modules installed:
npm install -g mocha
npm install should
The suite is run by running mocha
in the project root:
% mocha
...................................
42 passing (23ms)
There is also a Guardfile present that enables automatically rebuilding
the module and running the tests when one of the source files
change. To use this you need the guard
and guard-shell
Ruby gems
installed. This can be done as follows:
gem install guard guard-shell
Then run guard
in the project root.
FAQs
Evented XML generation using the Genx C library
The npm package genx receives a total of 2,020 weekly downloads. As such, genx popularity was classified as popular.
We found that genx demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.