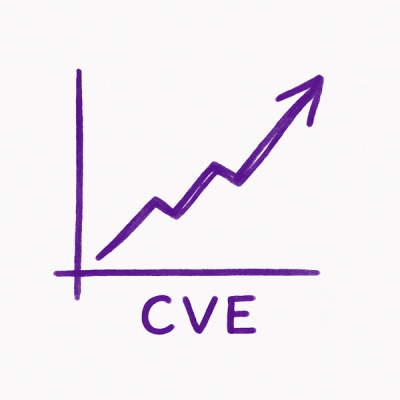
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
get-executable-bin-path
Advanced tools
Get the current package's binary path and ensure it's executable.
Get the current package's binary path and ensure it's executable.
Useful for making sure your CLIs are configured correctly.
npm install --save-dev get-executable-bin-path
yarn add --dev get-executable-bin-path
import anyTest, { type TestFn } from "ava";
import { execa } from "execa";
import { getExecutableBinPath } from "get-executable-bin-path";
const test = anyTest as TestFn<{
binPath: string;
}>;
test.before("setup context", async t => {
t.context.binPath = await getExecutableBinPath();
});
test("main", async t => {
const { stdout } = await execa(t.context.binPath);
t.is(stdout, /* … */);
});
Promise<string>
string
Type: object
Type: string
Default: package.json
name
field
Name of the binary. See get-bin-path
for more details.
Type: string
Default: process.cwd()
Override the current directory. Used when retrieving the package.json
.
Type: (binPath: string) => string
An optional mapping that resolves to a binary's path.
This can be used to get the path of the source binary, for example.
const binPath = await getExecutableBinPath();
//=> "…/dist/cli.js"
const mappedBinPath = await getExecutableBinPath({
map: binPath => binPath.replace("dist", "src").replace(".js", ".ts"),
});
//=> "…/src/cli.ts"
The error thrown when a given binary cannot be resolved.
The error thrown when a given binary is not executable or resolvable.
FAQs
Get the current package's binary path and ensure it's executable.
The npm package get-executable-bin-path receives a total of 6 weekly downloads. As such, get-executable-bin-path popularity was classified as not popular.
We found that get-executable-bin-path demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.