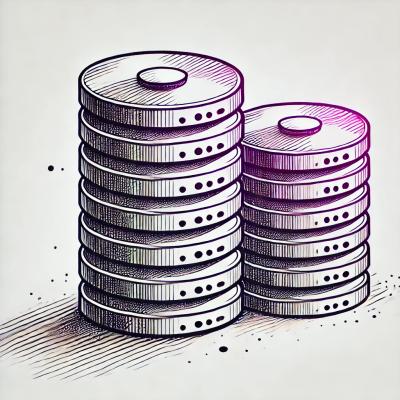
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
get-package-type
Advanced tools
The `get-package-type` npm package is designed to determine the type of a Node.js package. It can identify if a package is a CommonJS or an ECMAScript module by analyzing the package's configuration and structure. This is particularly useful in environments where understanding the module type is necessary for compatibility or transformation purposes.
Determine package type
This feature allows you to determine whether a given package is a CommonJS or ECMAScript module. The function `getPackageType` takes the path to the package as an argument and returns a promise that resolves with the type of the package.
const getPackageType = require('get-package-type');
async function checkPackageType() {
const type = await getPackageType('/path/to/package');
console.log(`Package type: ${type}`); // 'commonjs' or 'module'
}
checkPackageType();
The `read-pkg` package reads the `package.json` file of a Node.js project and parses it into a JavaScript object. While it doesn't directly determine the module type like `get-package-type`, it can be used to manually check the `type` field in the `package.json` to infer if a package is a CommonJS or ECMAScript module.
The `pkg-dir` package finds the root directory of a Node.js project or npm package. This can be a complementary tool to `get-package-type` by first finding the root directory of a package and then using `get-package-type` to determine the package type. However, `pkg-dir` itself does not provide functionality to identify the module type.
Determine the package.json#type
which applies to a location.
const getPackageType = require('get-package-type');
(async () => {
console.log(await getPackageType('file.js'));
console.log(getPackageType.sync('file.js'));
})();
This function does not validate the value found in package.json#type
. Any truthy value
found will be returned. Non-truthy values will be reported as commonjs
.
The argument must be a filename.
// This never looks at `dir1/`, first attempts to load `./package.json`.
const type1 = await getPackageType('dir1/');
// This attempts to load `dir1/package.json`.
const type2 = await getPackageType('dir1/index.cjs');
The extension of the filename does not effect the result. The primary use case for this
module is to determine if myapp.config.js
should be loaded with require
or import
.
FAQs
Determine the `package.json#type` which applies to a location
The npm package get-package-type receives a total of 21,963,845 weekly downloads. As such, get-package-type popularity was classified as popular.
We found that get-package-type demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.