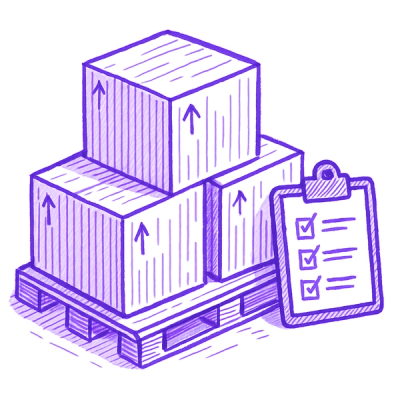
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
The 'getenv' npm package is a utility for safely and easily accessing environment variables in Node.js applications. It provides a straightforward API to retrieve environment variables with type safety and default values.
Get a string environment variable
This feature allows you to retrieve a string environment variable. If the variable is not set, it will throw an error.
const getenv = require('getenv');
const dbHost = getenv('DB_HOST');
Get a string environment variable with a default value
This feature allows you to retrieve a string environment variable and provide a default value in case the variable is not set.
const getenv = require('getenv');
const dbHost = getenv('DB_HOST', 'localhost');
Get an integer environment variable
This feature allows you to retrieve an integer environment variable. If the variable is not set or cannot be converted to an integer, it will throw an error.
const getenv = require('getenv');
const port = getenv.int('PORT');
Get an integer environment variable with a default value
This feature allows you to retrieve an integer environment variable and provide a default value in case the variable is not set or cannot be converted to an integer.
const getenv = require('getenv');
const port = getenv.int('PORT', 3000);
Get a boolean environment variable
This feature allows you to retrieve a boolean environment variable. If the variable is not set or cannot be converted to a boolean, it will throw an error.
const getenv = require('getenv');
const isProduction = getenv.bool('IS_PRODUCTION');
Get a boolean environment variable with a default value
This feature allows you to retrieve a boolean environment variable and provide a default value in case the variable is not set or cannot be converted to a boolean.
const getenv = require('getenv');
const isProduction = getenv.bool('IS_PRODUCTION', false);
The 'dotenv' package loads environment variables from a .env file into process.env. It is widely used for managing environment variables in Node.js applications. Unlike 'getenv', 'dotenv' does not provide type safety or default values.
The 'env-var' package provides a similar functionality to 'getenv' with additional features like type validation, default values, and custom transformations. It offers a more comprehensive API for handling environment variables.
The 'config' package allows you to manage configuration files for your Node.js application. It supports environment-specific configurations and provides a more structured approach to managing environment variables compared to 'getenv'.
Helper to get and typecast environment variables. This is especially useful if you are building Twelve-Factor-Apps where all configuration is stored in the environment.
npm install getenv
TypeScript types are available from the @types/getenv
module.
Set environment variables:
export HTTP_HOST="localhost"
export HTTP_PORT=8080
export HTTP_START=true
export AB_TEST_RATIO=0.5
export KEYWORDS="sports,business"
export PRIMES="2,3,5,7"
Get and use them:
const getenv = require('getenv');
const host = getenv('HTTP_HOST'); // same as getenv.string('HTTP_HOST');
const port = getenv.int('HTTP_PORT');
const start = getenv.bool('HTTP_START');
if (start === true) {
// const server = http.createServer();
// server.listen(port, host);
}
const abTestRatio = getenv.float('AB_TEST_RATIO');
if (Math.random() < abTestRatio) {
// test A
} else {
// test B
}
const keywords = getenv.array('KEYWORDS');
keywords.forEach(function (keyword) {
// console.log(keyword);
});
const primes = getenv.array('PRIMES', 'int');
primes.forEach(function (prime) {
// console.log(prime, typeof prime);
});
All methods accept a fallback value that will be returned if the requested environment variable is not set. If the fallback value is omitted and if the requested environment variable does not exist, an exception is thrown.
Alias for env.string(name, [fallback])
.
Return as string.
Return as integer number.
Return as float number.
Return as boolean. Only allows true/false as valid values.
Return as boolean. Allows true/false/1/0 as valid values.
Split value of the environment variable at each comma (default) and return the resulting array where each value has been typecast according to the type
parameter. An array can be provided as fallback
and a regular expression can be provided as separator
in case commas don't fit.
Return a list of environment variables based on a spec
:
const config = getenv.multi({
foo: 'FOO', // throws if FOO doesn't exist
bar: ['BAR', 'defaultval'], // set a default value
baz: ['BAZ', 'defaultval', 'string'], // parse into type
quux: ['QUUX', undefined, 'int'], // parse & throw
});
Return a parsed URL as per Node's require("url").parse
. N.B url
doesn't validate URLs, so be sure it includes a protocol or you'll get deeply weird results.
const serviceUrl = getenv.url('SERVICE_URL');
serviceUrl.port; // parsed port number
Disallows fallbacks in environments where you don't want to rely on brittle development defaults (e.g production, integration testing). For example, to disable fallbacks if we indicate production via NODE_ENV
:
if (process.env.NODE_ENV === 'production') {
getenv.disableFallbacks();
}
getenv
won't throw any error. If a fallback value is provided, that will be returned, else undefined
is returned.
getenv.disableErrors();
console.log(getenv('RANDOM'));
// undefined
Revert the effect of disableErrors()
.
getenv.disableErrors();
console.log(getenv('RANDOM'));
// undefined
getenv.enableErrors();
console.log(getenv('RANDOM'));
// Error: GetEnv.Nonexistent: RANDOM does not exist and no fallback value provided.
env.bool()
(#22)env.array()
(#19)getenv.multi()
support.disableFallbacks()
, url()
disableErrors()
, enableErrors()
This module is licensed under the MIT license.
FAQs
Get and typecast environment variables.
The npm package getenv receives a total of 1,843,362 weekly downloads. As such, getenv popularity was classified as popular.
We found that getenv demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.