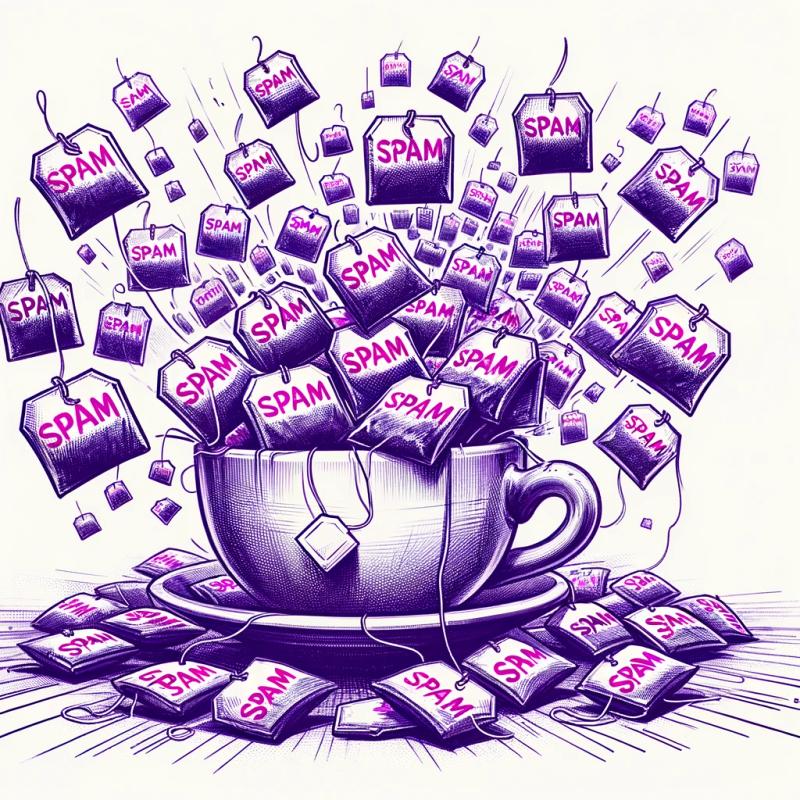
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
ghrr
Advanced tools
Readme
Receive all Github events in realtime with socket.io from the Github Realtime Relay. This is probably the simplest way to create a realtime application on top of Github.
Install the socket.io-client from npm.
npm install socket.io-client
To receive all events you can hook onto the /events
namespace
and subscribe to a specific Github Event. Please use lower case for subscribing to the event types.
var url = 'http://ghrr.lukasmartinelli.ch:80/events';
var socket = require('socket.io-client')(url);
socket.on('pushevent', function(event){
console.log('Push: ' + event.repository.full_name);
});
There is also a /statistics
namespace used by the GHRR web interface that
sends usage statistics for the Event Types.
var url = 'http://ghrr.lukasmartinelli.ch:80';
var io = require('socket.io-client')(url);
io('/statistics').on('types', function(typeCounts) {
console.log('PushEvents: ' + typeCounts.pushevent);
}
You need to add the socket.io-client to your web application.
<script src="//cdn.jsdelivr.net/socket.io-client/1.2.0/socket.io.js"></script>
You can now connect directly to the public websocket. We support CORS for all domains so you should not encounter any problems.
var url = 'ghrr.lukasmartinelli.ch:80/events';
var socket = io(url);
socket.on('pushevent', function (event) {
console.log('Push: ' + event.repository.full_name);
});
Install with npm.
npm install ghrr
In order to poll all events you need an OAUTH access token.
Run the github realtime relay with a poll rate of 1000
and on port 80
.
npm install ghrr
npm run start "YOUR GITHUB ACCESS TOKEN" 1000 80
Now you can visit the status page displaying live statistics.
FAQs
Github Realtime Relay
The npm package ghrr receives a total of 2 weekly downloads. As such, ghrr popularity was classified as not popular.
We found that ghrr demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.