google-auth-library
Advanced tools
Comparing version
@@ -409,5 +409,5 @@ "use strict"; | ||
'Content-Type': 'application/x-www-form-urlencoded', | ||
Authorization: `Bearer ${accessToken}`, | ||
}, | ||
url: OAuth2Client.GOOGLE_TOKEN_INFO_URL, | ||
data: querystring.stringify({ access_token: accessToken }), | ||
}); | ||
@@ -414,0 +414,0 @@ const info = Object.assign({ |
@@ -7,2 +7,9 @@ # Changelog | ||
### [6.1.4](https://www.github.com/googleapis/google-auth-library-nodejs/compare/v6.1.3...v6.1.4) (2020-12-22) | ||
### Bug Fixes | ||
* move accessToken to headers instead of parameter ([#1108](https://www.github.com/googleapis/google-auth-library-nodejs/issues/1108)) ([67b0cc3](https://www.github.com/googleapis/google-auth-library-nodejs/commit/67b0cc3077860a1583bcf18ce50aeff58bbb5496)) | ||
### [6.1.3](https://www.github.com/googleapis/google-auth-library-nodejs/compare/v6.1.2...v6.1.3) (2020-10-22) | ||
@@ -9,0 +16,0 @@ |
{ | ||
"name": "google-auth-library", | ||
"version": "6.1.3", | ||
"version": "6.1.4", | ||
"author": "Google Inc.", | ||
@@ -46,3 +46,3 @@ "description": "Google APIs Authentication Client Library for Node.js", | ||
"codecov": "^3.0.2", | ||
"execa": "^4.0.0", | ||
"execa": "^5.0.0", | ||
"gts": "^2.0.0", | ||
@@ -49,0 +49,0 @@ "is-docker": "^2.0.0", |
189
README.md
@@ -1,17 +0,47 @@ | ||
<img src="https://avatars0.githubusercontent.com/u/1342004?v=3&s=96" alt="Google Inc. logo" title="Google" align="right" height="96" width="96"/> | ||
[//]: # "This README.md file is auto-generated, all changes to this file will be lost." | ||
[//]: # "To regenerate it, use `python -m synthtool`." | ||
<img src="https://avatars2.githubusercontent.com/u/2810941?v=3&s=96" alt="Google Cloud Platform logo" title="Google Cloud Platform" align="right" height="96" width="96"/> | ||
# Google Auth Library | ||
# [Google Auth Library: Node.js Client](https://github.com/googleapis/google-auth-library-nodejs) | ||
[![npm version][npmimg]][npm] | ||
[![codecov][codecov-image]][codecov-url] | ||
[![Dependencies][david-dm-img]][david-dm] | ||
[![Known Vulnerabilities][snyk-image]][snyk-url] | ||
[](https://cloud.google.com/terms/launch-stages) | ||
[](https://www.npmjs.org/package/google-auth-library) | ||
[](https://codecov.io/gh/googleapis/google-auth-library-nodejs) | ||
This is Google's officially supported [node.js][node] client library for using OAuth 2.0 authorization and authentication with Google APIs. | ||
## Installation | ||
This library is distributed on `npm`. To add it as a dependency, run the following command: | ||
``` sh | ||
$ npm install google-auth-library | ||
This is Google's officially supported [node.js](http://nodejs.org/) client library for using OAuth 2.0 authorization and authentication with Google APIs. | ||
A comprehensive list of changes in each version may be found in | ||
[the CHANGELOG](https://github.com/googleapis/google-auth-library-nodejs/blob/master/CHANGELOG.md). | ||
* [Google Auth Library Node.js Client API Reference][client-docs] | ||
* [Google Auth Library Documentation][product-docs] | ||
* [github.com/googleapis/google-auth-library-nodejs](https://github.com/googleapis/google-auth-library-nodejs) | ||
Read more about the client libraries for Cloud APIs, including the older | ||
Google APIs Client Libraries, in [Client Libraries Explained][explained]. | ||
[explained]: https://cloud.google.com/apis/docs/client-libraries-explained | ||
**Table of contents:** | ||
* [Quickstart](#quickstart) | ||
* [Installing the client library](#installing-the-client-library) | ||
* [Samples](#samples) | ||
* [Versioning](#versioning) | ||
* [Contributing](#contributing) | ||
* [License](#license) | ||
## Quickstart | ||
### Installing the client library | ||
```bash | ||
npm install google-auth-library | ||
``` | ||
@@ -27,3 +57,3 @@ | ||
## Application Default Credentials | ||
This library provides an implementation of [Application Default Credentials][] for Node.js. The [Application Default Credentials][] provide a simple way to get authorization credentials for use in calling Google APIs. | ||
This library provides an implementation of [Application Default Credentials](https://cloud.google.com/docs/authentication/getting-started)for Node.js. The [Application Default Credentials](https://cloud.google.com/docs/authentication/getting-started) provide a simple way to get authorization credentials for use in calling Google APIs. | ||
@@ -34,3 +64,3 @@ They are best suited for cases when the call needs to have the same identity and authorization level for the application independent of the user. This is the recommended approach to authorize calls to Cloud APIs, particularly when you're building an application that uses Google Cloud Platform. | ||
To use Application Default Credentials, You first need to download a set of JSON credentials for your project. Go to **APIs & Auth** > **Credentials** in the [Google Developers Console][devconsole] and select **Service account** from the **Add credentials** dropdown. | ||
To use Application Default Credentials, You first need to download a set of JSON credentials for your project. Go to **APIs & Auth** > **Credentials** in the [Google Developers Console](https://console.cloud.google.com/) and select **Service account** from the **Add credentials** dropdown. | ||
@@ -44,3 +74,3 @@ > This file is your *only copy* of these credentials. It should never be | ||
Before making your API call, you must be sure the API you're calling has been enabled. Go to **APIs & Auth** > **APIs** in the [Google Developers Console][devconsole] and enable the APIs you'd like to call. For the example below, you must enable the `DNS API`. | ||
Before making your API call, you must be sure the API you're calling has been enabled. Go to **APIs & Auth** > **APIs** in the [Google Developers Console](https://console.cloud.google.com/) and enable the APIs you'd like to call. For the example below, you must enable the `DNS API`. | ||
@@ -60,5 +90,5 @@ | ||
/** | ||
* Instead of specifying the type of client you'd like to use (JWT, OAuth2, etc) | ||
* this library will automatically choose the right client based on the environment. | ||
*/ | ||
* Instead of specifying the type of client you'd like to use (JWT, OAuth2, etc) | ||
* this library will automatically choose the right client based on the environment. | ||
*/ | ||
async function main() { | ||
@@ -80,7 +110,7 @@ const auth = new GoogleAuth({ | ||
This library comes with an [OAuth2][oauth] client that allows you to retrieve an access token and refreshes the token and retry the request seamlessly if you also provide an `expiry_date` and the token is expired. The basics of Google's OAuth2 implementation is explained on [Google Authorization and Authentication documentation][authdocs]. | ||
This library comes with an [OAuth2](https://developers.google.com/identity/protocols/OAuth2) client that allows you to retrieve an access token and refreshes the token and retry the request seamlessly if you also provide an `expiry_date` and the token is expired. The basics of Google's OAuth2 implementation is explained on [Google Authorization and Authentication documentation](https://developers.google.com/accounts/docs/OAuth2Login). | ||
In the following examples, you may need a `CLIENT_ID`, `CLIENT_SECRET` and `REDIRECT_URL`. You can find these pieces of information by going to the [Developer Console][devconsole], clicking your project > APIs & auth > credentials. | ||
In the following examples, you may need a `CLIENT_ID`, `CLIENT_SECRET` and `REDIRECT_URL`. You can find these pieces of information by going to the [Developer Console](https://console.cloud.google.com/), clicking your project > APIs & auth > credentials. | ||
For more information about OAuth2 and how it works, [see here][oauth]. | ||
For more information about OAuth2 and how it works, [see here](https://developers.google.com/identity/protocols/OAuth2). | ||
@@ -102,4 +132,4 @@ #### A complete OAuth2 example | ||
/** | ||
* Start by acquiring a pre-authenticated oAuth2 client. | ||
*/ | ||
* Start by acquiring a pre-authenticated oAuth2 client. | ||
*/ | ||
async function main() { | ||
@@ -122,5 +152,5 @@ const oAuth2Client = await getAuthenticatedClient(); | ||
/** | ||
* Create a new OAuth2Client, and go through the OAuth2 content | ||
* workflow. Return the full client to the callback. | ||
*/ | ||
* Create a new OAuth2Client, and go through the OAuth2 content | ||
* workflow. Return the full client to the callback. | ||
*/ | ||
function getAuthenticatedClient() { | ||
@@ -240,5 +270,5 @@ return new Promise((resolve, reject) => { | ||
#### OAuth2 with Installed Apps (Electron) | ||
If you're authenticating with OAuth2 from an installed application (like Electron), you may not want to embed your `client_secret` inside of the application sources. To work around this restriction, you can choose the `iOS` application type when creating your OAuth2 credentials in the [Google Developers console][devconsole]: | ||
If you're authenticating with OAuth2 from an installed application (like Electron), you may not want to embed your `client_secret` inside of the application sources. To work around this restriction, you can choose the `iOS` application type when creating your OAuth2 credentials in the [Google Developers console](https://console.cloud.google.com/): | ||
![application type][apptype] | ||
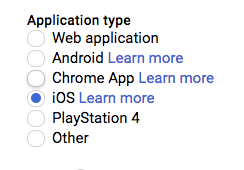 | ||
@@ -423,32 +453,91 @@ If using the `iOS` type, when creating the OAuth2 client you won't need to pass a `client_secret` into the constructor: | ||
## Questions/problems? | ||
* Ask your development related questions on [Stack Overflow][stackoverflow]. | ||
* If you've found an bug/issue, please [file it on GitHub][bugs]. | ||
## Samples | ||
Samples are in the [`samples/`](https://github.com/googleapis/google-auth-library-nodejs/tree/master/samples) directory. Each sample's `README.md` has instructions for running its sample. | ||
| Sample | Source Code | Try it | | ||
| --------------------------- | --------------------------------- | ------ | | ||
| Adc | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/adc.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/adc.js,samples/README.md) | | ||
| Compute | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/compute.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/compute.js,samples/README.md) | | ||
| Credentials | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/credentials.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/credentials.js,samples/README.md) | | ||
| Headers | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/headers.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/headers.js,samples/README.md) | | ||
| ID Tokens for Identity-Aware Proxy (IAP) | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/idtokens-iap.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/idtokens-iap.js,samples/README.md) | | ||
| ID Tokens for Serverless | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/idtokens-serverless.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/idtokens-serverless.js,samples/README.md) | | ||
| Jwt | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/jwt.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/jwt.js,samples/README.md) | | ||
| Keepalive | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/keepalive.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/keepalive.js,samples/README.md) | | ||
| Keyfile | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/keyfile.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/keyfile.js,samples/README.md) | | ||
| Oauth2-code Verifier | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/oauth2-codeVerifier.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/oauth2-codeVerifier.js,samples/README.md) | | ||
| Oauth2 | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/oauth2.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/oauth2.js,samples/README.md) | | ||
| Sign Blob | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/signBlob.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/signBlob.js,samples/README.md) | | ||
| Verifying ID Tokens from Identity-Aware Proxy (IAP) | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/verifyIdToken-iap.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/verifyIdToken-iap.js,samples/README.md) | | ||
| Verify Id Token | [source code](https://github.com/googleapis/google-auth-library-nodejs/blob/master/samples/verifyIdToken.js) | [![Open in Cloud Shell][shell_img]](https://console.cloud.google.com/cloudshell/open?git_repo=https://github.com/googleapis/google-auth-library-nodejs&page=editor&open_in_editor=samples/verifyIdToken.js,samples/README.md) | | ||
The [Google Auth Library Node.js Client API Reference][client-docs] documentation | ||
also contains samples. | ||
## Supported Node.js Versions | ||
Our client libraries follow the [Node.js release schedule](https://nodejs.org/en/about/releases/). | ||
Libraries are compatible with all current _active_ and _maintenance_ versions of | ||
Node.js. | ||
Client libraries targeting some end-of-life versions of Node.js are available, and | ||
can be installed via npm [dist-tags](https://docs.npmjs.com/cli/dist-tag). | ||
The dist-tags follow the naming convention `legacy-(version)`. | ||
_Legacy Node.js versions are supported as a best effort:_ | ||
* Legacy versions will not be tested in continuous integration. | ||
* Some security patches may not be able to be backported. | ||
* Dependencies will not be kept up-to-date, and features will not be backported. | ||
#### Legacy tags available | ||
* `legacy-8`: install client libraries from this dist-tag for versions | ||
compatible with Node.js 8. | ||
## Versioning | ||
This library follows [Semantic Versioning](http://semver.org/). | ||
This library is considered to be **General Availability (GA)**. This means it | ||
is stable; the code surface will not change in backwards-incompatible ways | ||
unless absolutely necessary (e.g. because of critical security issues) or with | ||
an extensive deprecation period. Issues and requests against **GA** libraries | ||
are addressed with the highest priority. | ||
More Information: [Google Cloud Platform Launch Stages][launch_stages] | ||
[launch_stages]: https://cloud.google.com/terms/launch-stages | ||
## Contributing | ||
See [CONTRIBUTING][contributing]. | ||
Contributions welcome! See the [Contributing Guide](https://github.com/googleapis/google-auth-library-nodejs/blob/master/CONTRIBUTING.md). | ||
Please note that this `README.md`, the `samples/README.md`, | ||
and a variety of configuration files in this repository (including `.nycrc` and `tsconfig.json`) | ||
are generated from a central template. To edit one of these files, make an edit | ||
to its template in this | ||
[directory](https://github.com/googleapis/synthtool/tree/master/synthtool/gcp/templates/node_library). | ||
## License | ||
This library is licensed under Apache 2.0. Full license text is available in [LICENSE][copying]. | ||
Apache Version 2.0 | ||
[Application Default Credentials]: https://cloud.google.com/docs/authentication/getting-started | ||
[apptype]: https://user-images.githubusercontent.com/534619/36553844-3f9a863c-17b2-11e8-904a-29f6cd5f807a.png | ||
[authdocs]: https://developers.google.com/accounts/docs/OAuth2Login | ||
[bugs]: https://github.com/googleapis/google-auth-library-nodejs/issues | ||
[codecov-image]: https://codecov.io/gh/googleapis/google-auth-library-nodejs/branch/master/graph/badge.svg | ||
[codecov-url]: https://codecov.io/gh/googleapis/google-auth-library-nodejs | ||
[contributing]: https://github.com/googleapis/google-auth-library-nodejs/blob/master/CONTRIBUTING.md | ||
[copying]: https://github.com/googleapis/google-auth-library-nodejs/tree/master/LICENSE | ||
[david-dm-img]: https://david-dm.org/googleapis/google-auth-library-nodejs/status.svg | ||
[david-dm]: https://david-dm.org/googleapis/google-auth-library-nodejs | ||
[node]: http://nodejs.org/ | ||
[npmimg]: https://img.shields.io/npm/v/google-auth-library.svg | ||
[npm]: https://www.npmjs.org/package/google-auth-library | ||
[oauth]: https://developers.google.com/identity/protocols/OAuth2 | ||
[snyk-image]: https://snyk.io/test/github/googleapis/google-auth-library-nodejs/badge.svg | ||
[snyk-url]: https://snyk.io/test/github/googleapis/google-auth-library-nodejs | ||
[stackoverflow]: http://stackoverflow.com/questions/tagged/google-auth-library-nodejs | ||
[devconsole]: https://console.cloud.google.com/ | ||
See [LICENSE](https://github.com/googleapis/google-auth-library-nodejs/blob/master/LICENSE) | ||
[client-docs]: https://googleapis.dev/nodejs/google-auth-library/latest | ||
[product-docs]: https://cloud.google.com/docs/authentication/ | ||
[shell_img]: https://gstatic.com/cloudssh/images/open-btn.png | ||
[projects]: https://console.cloud.google.com/project | ||
[billing]: https://support.google.com/cloud/answer/6293499#enable-billing | ||
[auth]: https://cloud.google.com/docs/authentication/getting-started |
242066
3.53%535
19.96%