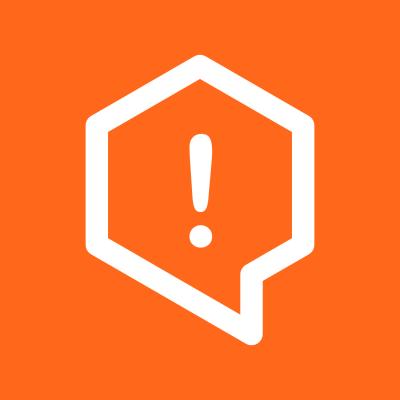
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
google-closure-library
Advanced tools
The google-closure-library is a comprehensive, well-tested, modular, and cross-browser JavaScript library. It provides a wide range of utilities for building complex web applications, including data structures, UI components, and language utilities.
Data Structures
The google-closure-library provides various data structures like Map, Set, and PriorityQueue. These structures are optimized for performance and provide additional methods compared to native JavaScript objects.
const goog = require('google-closure-library');
require('google-closure-library/closure/goog/structs/map');
const map = new goog.structs.Map();
map.set('key1', 'value1');
map.set('key2', 'value2');
console.log(map.get('key1')); // Output: 'value1'
UI Components
The library includes a set of UI components that can be used to build interactive web applications. These components are highly customizable and support various themes and styles.
const goog = require('google-closure-library');
require('google-closure-library/closure/goog/ui/button');
const button = new goog.ui.Button('Click Me');
button.render(document.body);
Localization and Internationalization
The library offers extensive support for localization and internationalization, including date and time formatting, number formatting, and message translations.
const goog = require('google-closure-library');
require('google-closure-library/closure/goog/i18n/datetimesymbols');
const symbols = goog.i18n.DateTimeSymbols;
console.log(symbols.WEEKDAYS); // Output: Array of weekday names
Lodash is a modern JavaScript utility library delivering modularity, performance, and extras. It provides utility functions for common programming tasks using a functional programming paradigm. While it doesn't offer UI components or localization features like google-closure-library, it excels in data manipulation and functional programming utilities.
React is a JavaScript library for building user interfaces, particularly single-page applications where data changes over time. Unlike google-closure-library, React focuses on the UI layer and component-based architecture, making it more suitable for building modern web applications with dynamic views.
Moment.js is a library for parsing, validating, manipulating, and displaying dates and times in JavaScript. It provides similar date and time functionalities as google-closure-library but is more focused and specialized in handling date and time operations.
Closure Library is a powerful, low-level JavaScript library designed for building complex and scalable web applications. It is used by many Google web applications, such as Google Search, Gmail, Google Docs, Google+, Google Maps, and others.
For more information, visit the Google Developers or GitHub sites.
Download the latest stable version on our releases page.
Developers, please see the Generated API Documentation.
See also the goog.ui Demos
Install the official package from npm.
npm install google-closure-library
Require the package and use goog.require normally.
require("google-closure-library");
goog.require("goog.crypt.Sha1");
var sha1 = new goog.crypt.Sha1();
sha1.update("foobar");
var hash = sha1.digest();
Please read the CONTRIBUTING for details on how to contribute to this project.
FAQs
Google's common JavaScript library
The npm package google-closure-library receives a total of 93,776 weekly downloads. As such, google-closure-library popularity was classified as popular.
We found that google-closure-library demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.