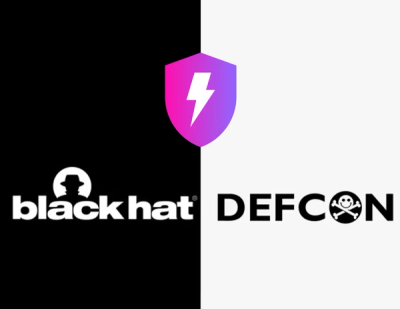
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
google-login-vue3
Advanced tools
A lightweight and customizable Vue 3 component for Google OAuth 2.0 authentication, supporting both code-based and JWT-based authentication modes.
A lightweight and customizable Vue 3 component for Google OAuth 2.0 authentication supporting both JWT-based and Authorization Code-based flows.
npm install google-login-vue3
<template>
<GoogleLogin
:clientId="YOUR_GOOGLE_CLIENT_ID"
mode="JWT"
:oneTapLogin="true"
@success="handleSuccess"
@error="handleError"
/>
<!-- Optional: mode="JWT" (default) or "code" -->
<!-- Optional: Enables Google One Tap (:oneTapLogin="true") in JWT mode -->
</template>
<script setup>
import GoogleLogin from "google-login-vue3";
const handleSuccess = (response) => {
console.log("Success response:", response);
if (response.credential) {
console.log("JWT Token:", response.credential);
// Send the token to your backend for verification
} else if (response.code) {
console.log("Authorization Code:", response.code);
// Send the code to your backend for access token exchange
}
};
const handleError = (error) => {
console.error("Google login error:", error);
};
</script>
Replace
YOUR_GOOGLE_CLIENT_ID
with your actual client ID from Google Developer Console.
oneTapLogin
prop.Prop | Type | Default | Description |
---|---|---|---|
clientId | String | ā | Required. Your Google OAuth 2.0 client ID. |
mode | String | "JWT" | "JWT" or "code" depending on the flow you want to use. |
oneTapLogin | Boolean | true | Enables Google One Tap login (only valid in JWT mode). |
code
mode, you can customize the login button using the default slot.Example:
<GoogleLogin mode="code" :clientId="clientId">
<template #default>
<button class="my-custom-btn">Sign in with Google</button>
</template>
</GoogleLogin>
mode="code"
)google-auth-library
google-auth
success
and error
events properly.Contributions are welcome! Feel free to:
Shiv Baran Singh
š§ spyshiv@gmail.com
š GitHub
FAQs
A lightweight and customizable Vue 3 component for Google OAuth 2.0 authentication, supporting both code-based and JWT-based authentication modes.
The npm package google-login-vue3 receives a total of 3 weekly downloads. As such, google-login-vue3 popularity was classified as not popular.
We found that google-login-vue3 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Ā It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600Ć faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.