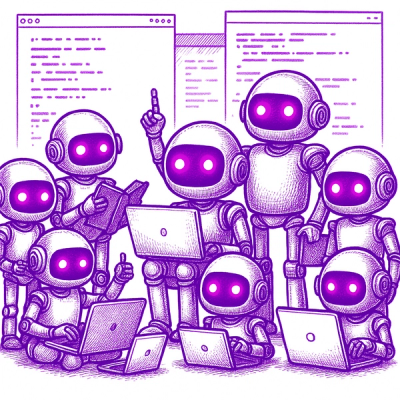
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
graphql-fields
Advanced tools
The graphql-fields npm package is a utility for extracting the fields requested in a GraphQL query. It helps in resolving only the necessary fields, optimizing the performance of your GraphQL server by avoiding over-fetching data.
Extracting Fields from a GraphQL Query
This feature allows you to extract the fields requested in a GraphQL query. By using the `graphqlFields` function and passing the `info` object from the resolver, you can get an object representing the fields requested. This can be used to optimize data fetching by only querying the necessary fields from your data source.
const graphqlFields = require('graphql-fields');
const resolvers = {
Query: {
user: (parent, args, context, info) => {
const fields = graphqlFields(info);
console.log(fields); // Logs the fields requested in the query
// Fetch and return the user data based on the requested fields
}
}
};
Handling Nested Fields
This feature allows you to handle nested fields in a GraphQL query. The `graphqlFields` function will return an object that includes nested fields, enabling you to understand the full structure of the query and fetch data accordingly.
const graphqlFields = require('graphql-fields');
const resolvers = {
Query: {
user: (parent, args, context, info) => {
const fields = graphqlFields(info);
console.log(fields); // Logs nested fields as well
// Fetch and return the user data based on the requested fields
}
}
};
The graphql-parse-resolve-info package provides similar functionality by parsing the resolve info to extract the fields requested in a GraphQL query. It offers more detailed information about the query, including arguments and fragments, making it a more comprehensive solution compared to graphql-fields.
Turns GraphQLResolveInfo into a map of the requested fields. Flattens all fragments and duplicated fields into a neat object to easily see which fields were requested at any level. Takes into account any @include
or @skip
directives, excluding fields/fragments which are @include(if: $false)
or @skip(if: $true)
.
Schema Type definition
const graphqlFields = require('graphql-fields');
const graphql = require('graphql')
const UserType = new graphql.GraphQLObjectType({
name: 'User',
fields: {
profile: {type: new graphql.GraphQLObjectType({
name: 'Profile',
fields: {
firstName: {type: graphql.GraphQLString},
lastName: {type: graphql.GraphQLString},
middleName: {type: graphql.GraphQLString},
nickName: {type: graphql.GraphQLString},
maidenName: {type: graphql.GraphQLString}
}
}),
email: {type: graphql.GraphQLString},
id: {type: graphql.GraphQLID}
}
});
module.exports = new GraphQLSchema({
query: new GraphQLObjectType({
name: 'Query',
fields: () =>
Object.assign({
user: {
type: UserType,
resolve(root, args, context, info) {
console.log(
JSON.stringify(graphqlFields(info), null, 2);
);
...
}
}
})
})
})
Query
{
user {
...A
profile {
...B
firstName
}
}
}
fragment A on User {
...C
id,
profile {
lastName
}
}
Fragment B on Profile {
firstName
nickName @skip(if: true)
}
Fragment C on User {
email,
profile {
middleName
maidenName @include(if: false)
}
}
will log
{
"profile": {
"firstName": {},
"lastName": {},
"middleName": {}
},
"email": {},
"id": {}
}
To enable subfields arguments parsing, you'll have to provide an option object to the function. This feature is disable by default.
const graphqlFields = require('graphql-fields');
const fieldsWithSubFieldsArgs = graphqlFields(info, {}, { processArguments: true });
For each subfield w/ arguments, a __arguments
property will be created.
It will be an array with the following format:
[
{
arg1Name: {
kind: ARG1_KIND,
value: ARG1_VALUE,
},
},
{
arg2Name: {
kind: ARG2_KIND,
value: ARG2_VALUE,
}
}
]
The kind property is here to help differentiate value cast to strings by javascript clients, such as enum values.
Most of the time we don't need __typename
to be sent to backend/rest api, we can exclude __typename
using this:
const graphqlFields = require('graphql-fields');
const fieldsWithoutTypeName = graphqlFields(info, {}, { excludedFields: ['__typename'] });
An underlying REST api may only return fields based on query params.
{
user {
profile {
firstName
},
id
}
}
should request /api/user?fields=profile,id
while
{
user {
email
}
}
should request /api/user?fields=email
Implement your resolve method like so:
resolve(root, args, context, info) {
const topLevelFields = Object.keys(graphqlFields(info));
return fetch(`/api/user?fields=${topLevelFields.join(',')}`);
}
npm test
2.0.3 (2019-03-02)
FAQs
Turns GraphQLResolveInfo into a map of the requested fields
The npm package graphql-fields receives a total of 132,919 weekly downloads. As such, graphql-fields popularity was classified as popular.
We found that graphql-fields demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.