graphql-modules
Advanced tools
Comparing version
@@ -1,2 +0,3 @@ | ||
import { DocumentNode, ExecutionResult, GraphQLSchema } from 'graphql'; | ||
import { DocumentNode, GraphQLSchema } from 'graphql'; | ||
import { ExecutionContextBuilder } from './context'; | ||
import { Application } from './types'; | ||
@@ -14,18 +15,9 @@ export interface ApolloRequestContext { | ||
} | ||
export interface ApolloGatewayLoadResult { | ||
executor: ApolloExecutor; | ||
} | ||
export type ApolloExecutor = (requestContext: ApolloRequestContext) => Promise<ExecutionResult>; | ||
export interface ApolloGatewayInterface { | ||
onSchemaLoadOrUpdate(callback: (schemaContext: { | ||
apiSchema: GraphQLSchema; | ||
coreSupergraphSdl: string; | ||
}) => void): () => void; | ||
load(): Promise<ApolloGatewayLoadResult>; | ||
stop(): Promise<void>; | ||
} | ||
export declare function apolloGatewayCreator({ schema, typeDefs, createExecution, }: { | ||
schema: Application['schema']; | ||
typeDefs: Application['typeDefs']; | ||
export declare function apolloExecutorCreator({ createExecution, }: { | ||
createExecution: Application['createExecution']; | ||
}): Application['createApolloGateway']; | ||
}): Application['createApolloExecutor']; | ||
export declare function apolloSchemaCreator({ createSubscription, contextBuilder, schema, }: { | ||
createSubscription: Application['createSubscription']; | ||
contextBuilder: ExecutionContextBuilder; | ||
schema: GraphQLSchema; | ||
}): () => GraphQLSchema; |
@@ -6,4 +6,4 @@ import { execute } from 'graphql'; | ||
}): (options?: { | ||
execute?: typeof execute | undefined; | ||
controller?: import("./types").OperationController | undefined; | ||
} | undefined) => typeof execute; | ||
execute?: typeof execute; | ||
controller?: import("./types").OperationController; | ||
}) => typeof execute; |
@@ -6,4 +6,4 @@ import { subscribe } from 'graphql'; | ||
}): (options?: { | ||
subscribe?: typeof subscribe | undefined; | ||
controller?: import("./types").OperationController | undefined; | ||
} | undefined) => typeof subscribe; | ||
subscribe?: typeof subscribe; | ||
controller?: import("./types").OperationController; | ||
}) => typeof subscribe; |
@@ -5,3 +5,3 @@ import { execute, subscribe, DocumentNode, GraphQLSchema, ExecutionResult } from 'graphql'; | ||
import type { MiddlewareMap } from '../shared/middleware'; | ||
import type { ApolloGatewayInterface, ApolloRequestContext } from './apollo'; | ||
import type { ApolloRequestContext } from './apollo'; | ||
import type { Single } from '../shared/types'; | ||
@@ -60,5 +60,12 @@ import type { InternalAppContext } from './application'; | ||
}): Execution; | ||
createApolloGateway(options?: { | ||
/** | ||
* @deprecated Use `createApolloExecutor`, `createExecution` and `createSubscription` methods instead. | ||
*/ | ||
createSchemaForApollo(): GraphQLSchema; | ||
/** | ||
* Experimental | ||
*/ | ||
createApolloExecutor(options?: { | ||
controller?: OperationController; | ||
}): ApolloGatewayInterface; | ||
}): ApolloExecutor; | ||
/** | ||
@@ -65,0 +72,0 @@ * @internal |
@@ -1,2 +0,2 @@ | ||
import { Type, InjectionToken, Provider } from './providers'; | ||
import { Type, InjectionToken, Provider, AbstractType } from './providers'; | ||
import { ResolvedProvider, GlobalProviderMap } from './resolution'; | ||
@@ -7,3 +7,3 @@ import { Key } from './registry'; | ||
export declare abstract class Injector { | ||
abstract get<T>(token: Type<T> | InjectionToken<T>, notFoundValue?: any): T; | ||
abstract get<T>(token: Type<T> | InjectionToken<T> | AbstractType<T>, notFoundValue?: any): T; | ||
} | ||
@@ -10,0 +10,0 @@ export declare class ReflectiveInjector implements Injector { |
@@ -27,3 +27,3 @@ export declare const Type: FunctionConstructor; | ||
export interface BaseProvider<T> extends ProviderOptions { | ||
provide: Type<T> | InjectionToken<T>; | ||
provide: Type<T> | InjectionToken<T> | AbstractType<T>; | ||
} | ||
@@ -30,0 +30,0 @@ export interface TypeProvider<T> extends Type<T> { |
@@ -7,3 +7,2 @@ import { DocumentNode } from 'graphql'; | ||
import { MiddlewareMap } from '../shared/middleware'; | ||
import { ResolvedProvider } from '../di/resolution'; | ||
export type TypeDefs = Plural<DocumentNode>; | ||
@@ -43,8 +42,5 @@ export type Resolvers = Plural<Record<string, any>>; | ||
id: ID; | ||
providers?: Provider[]; | ||
typeDefs: DocumentNode[]; | ||
metadata: ModuleMetadata; | ||
factory: ModuleFactory; | ||
operationProviders: ResolvedProvider[]; | ||
singletonProviders: ResolvedProvider[]; | ||
config: ModuleConfig; | ||
@@ -51,0 +47,0 @@ } |
{ | ||
"name": "graphql-modules", | ||
"version": "3.0.0-alpha-20231106133212-0b04b56e", | ||
"version": "3.0.0-alpha-20250219090434-d6cc8940d97c7ef3dba3f59c56376d7cbab77c83", | ||
"description": "Create reusable, maintainable, testable and extendable GraphQL modules", | ||
@@ -11,4 +11,5 @@ "sideEffects": false, | ||
"@graphql-tools/schema": "^10.0.0", | ||
"@graphql-tools/wrap": "^10.0.0", | ||
"@graphql-typed-document-node/core": "^3.1.0", | ||
"ramda": "^0.29.0" | ||
"ramda": "^0.30.0" | ||
}, | ||
@@ -15,0 +16,0 @@ "keywords": [ |
@@ -1,3 +0,6 @@ | ||
[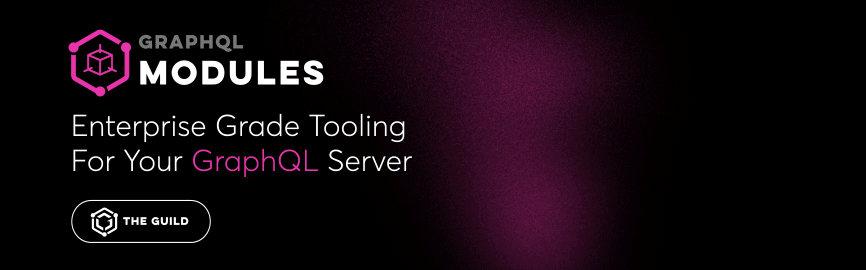](https://graphql-modules.com/) | ||
[](https://graphql.org/conf/2024/?utm_source=github&utm_medium=graphql_modules&utm_campaign=readme) | ||
<!-- Uncomment when we remove GraphQL Conf banner --> | ||
<!-- [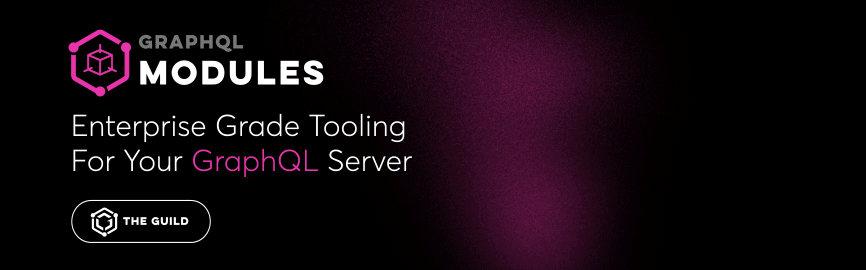](https://graphql-modules.com/) --> | ||
[](https://www.npmjs.com/package/graphql-modules) | ||
@@ -4,0 +7,0 @@  |
@@ -19,4 +19,4 @@ import { GraphQLResolveInfo } from 'graphql'; | ||
}; | ||
export declare function createMiddleware(path: string[], middlewareMap?: MiddlewareMap): (context: MiddlewareContext, next: Next<any>) => Promise<any>; | ||
export declare function createMiddleware(path: string[], middlewareMap?: MiddlewareMap): (context: MiddlewareContext, next: Next) => Promise<any>; | ||
export declare function mergeMiddlewareMaps(app: MiddlewareMap, mod: MiddlewareMap): MiddlewareMap; | ||
export declare function validateMiddlewareMap(middlewareMap: MiddlewareMap, metadata: ModuleMetadata): void; |
@@ -7,3 +7,3 @@ export declare function flatten<T>(arr: T[]): T extends (infer A)[] ? A[] : T[]; | ||
export declare function isAsyncIterable(obj: any): obj is AsyncIterableIterator<any>; | ||
export declare function tapAsyncIterator<T>(iterable: AsyncIterable<T>, doneCallback: () => void): AsyncGenerator<T>; | ||
export declare function tapAsyncIterator<T, TAsyncIterableIterator extends AsyncIterableIterator<T>>(iterable: TAsyncIterableIterator, doneCallback: () => void): TAsyncIterableIterator; | ||
export declare function once(cb: () => void): () => void; | ||
@@ -10,0 +10,0 @@ export declare function share<T, A>(factory: (arg?: A) => T): (arg?: A) => T; |
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is not supported yet
201677
2.78%5339
2.52%93
3.33%5
25%+ Added
+ Added
+ Added
+ Added
+ Added
+ Added
+ Added
+ Added
+ Added
- Removed
Updated