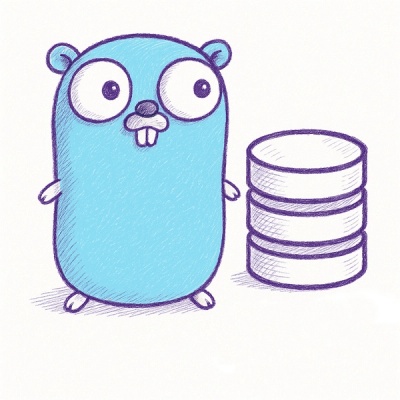
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
graphql-query-builder-v2
Advanced tools
Since graphql-query-builder seems to no longer be actively maintained, I have forked it. Nothing much has changed except the ability to use null
and undefined
values in the arguments. You are also allowed to not execute .find
API is unchanged.
let query =
new QueryBuilder(
"functionName",
{
name: null
}
)
Output
functionName( name: null )
let query =
new QueryBuilder(
"functionName",
{
name: null
}
)
query.find([
"_id",
"name"
])
Output
functionName( name: null ) {
_id,
name
}
let query =
new QueryBuilder(
"functionName",
{
status: QueryBuilder.Enum('draft')
}
)
Output
functionName( status: draft )
As I have recently started using graphql-upload, I am sharing how this package can also be used to create a valid FormData query.
const QueryBuilder = require("graphql-query-builder-v2");
// The wrapping "mutation"
let mutation = new QueryBuilder("mutation");
mutation.filter({
$image: QueryBuilder.Enum("Upload")
});
// Nested data within actual_query
const nested = {
data1: "stuff",
image: QueryBuilder.Enum("$image")
};
// The actual query we want to run
let actual_query = new QueryBuilder("imageUpload");
actual_query.filter({
_id: 1,
display_name: "example",
nested
});
// putting the actual query into the wrapping mutation
mutation.find([
actual_query
]);
let form_data = new FormData();
const operations = {
query: mutation_query.toString(),
variables: {
image: null
}
};
form_data.append("operations", JSON.stringify(operations));
const map = {
"0": ["variables.image"]
};
form_data.append("map", JSON.stringify(map));
form_data.append("0", image_file); // image_file is variable that is holding your File/Blob
Output
mutation output
mutation($image: Upload) {
imageUpload(
_id: 1,
display_name: "example",
nested: {
data1: "stuff",
image: $image
})
}
Use this instead of the install below.
npm install graphql-query-builder-v2
a simple but powerful graphQL query builder
info:
tests:
quality:
npm install graphql-query-builder
const Query = require('graphql-query-builder');
query/mutator you wish to use, and an alias or filter arguments.
Argument (one to two) | Description |
---|---|
String | the name of the query function |
* String / Object | (optional) This can be an alias or filter values |
let profilePicture = new Query("profilePicture",{size : 50});
set an alias for this result.
Argument | Description |
---|---|
String | The alias for this result |
profilePicture.setAlias("MyPic");
the parameters to run the query against.
Argument | Description |
---|---|
Object | An object mapping attribute to values |
profilePicture.filter({ height : 200, width : 200});
outlines the properties you wish to be returned from the query.
Argument (one to many) | Description |
---|---|
String or Object | representing each attribute you want Returned |
... | same as above |
profilePicture.find( { link : "uri"}, "width", "height");
return to the formatted query string
// A (ES6)
`${profilePicture}`;
// B
profilePicture+'';
// C
profilePicture.toString();
node example/simple.js
var Query = require('graphql-query-builder');
// example of nesting Querys
let profilePicture = new Query("profilePicture",{size : 50});
profilePicture.find( "uri", "width", "height");
let user = new Query("user",{id : 123});
user.find(["id", {"nickname":"name"}, "isViewerFriend", {"image":profilePicture}])
console.log(user)
/*
user( id:123 ) {
id,
nickname : name,
isViewerFriend,
image : profilePicture( size:50 ) {
uri,
width,
height
}
}
*/
// And another example
let MessageRequest = { type:"chat", message:"yoyo",
user:{
name:"bob",
screen:{
height:1080,
width:1920
}
},
friends:[
{id:1,name:"ann"},
{id:2,name:"tom"}
]
};
let MessageQuery = new Query("Message","myPost");
MessageQuery.filter(MessageRequest);
MessageQuery.find({ messageId : "id"}, {postedTime : "createTime" });
console.log(MessageQuery);
/*
myPost:Message( type:"chat",
message:"yoyo",
user:{name:"bob",screen:{height:1080,width:1920}},
friends:[{id:1,name:"ann"},{id:2,name:"tom"}])
{
messageId : id,
postedTime : createTime
}
*/
// Simple nesting
let user = new Query("user");
user.find([{"profilePicture":["uri", "width", "height"]}])
/*
user {
profilePicture {
uri,
width,
height
}
}
*/
// Simple nesting with rename
let user = new Query("user");
user.find([{"image":{"profilePicture":["uri", "width", "height"]}}])
/*
user {
image : profilePicture {
uri,
width,
height
}
}
*/
FAQs
a simple but powerful graphQL query builder
The npm package graphql-query-builder-v2 receives a total of 172 weekly downloads. As such, graphql-query-builder-v2 popularity was classified as not popular.
We found that graphql-query-builder-v2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.