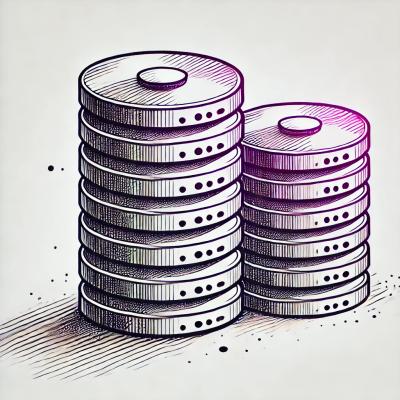
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
An intuitive data structure for graphs, implemented using ES6 data structures.
var Graph = require('graphs')
var graph = new Graph()
var a = {name: 'a'}
var b = {name: 'b'}
graph.add(a)
graph.add(b)
graph.link(a, b)
graph.traverse(function(from, to) {
console.log(from.name, 'linked to', to.name)
})
// => a linked to b
var graph = new Graph()
var a = {name: 'a'}
graph.add(a)
graph.has(a) // => true
graph.size // => 1
var b = {name: 'b'}
graph.has(b) // => false
graph.size // => 2
graph.add(b)
graph.has(b) // => true
graph.link(a, b)
Linking will also add nodes to the graph.
.to
and .from
return ES6 Sets of connected nodes.
graph.link(a, b)
graph.from(a) // Set of nodes connected from a
graph.from(a).size // => 1
graph.from(a).has(b) // => true
graph.to(b) // Set of nodes connected to b
graph.to(b).has(a) // => true
graph.from(b).size // => 0
graph.unlink(a, b)
graph.from(a).size // => 0
graph.delete(b)
.forEach
will even include entirely unlinked nodes.graph.forEach(function(node) {
console.log('node: %s', node.name)
})
graph.traverse
will traverse all links from the specified node.
from, to
from
and to
parameters.graph.traverse(startNode, function(from, to) {
console.log('from: %s', from)
console.log('to: %s', to)
})
graph.visit
will visit each node that can be reached from the specified node, once.
to, from
from
argument may not be set if visit
didn't follow a link to
the current node (e.g. on the first iteration).graph.visit(startNode, function(node, linkedFrom) {
console.log('node: %s', node)
console.log('linkedFrom: %s', linkedFrom)
})
Makes it easy to embed custom logic into your graph.
graph.before('add', function(a,b) {
// execute before add
})
graph.after('add', function(a,b) {
// execute after add
})
graph.guard('add', function(node) {
// prevent add from running if return falsey
})
See these libraries for usage information:
MIT
FAQs
Intuitive data structure for graphs
The npm package graphs receives a total of 15 weekly downloads. As such, graphs popularity was classified as not popular.
We found that graphs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.