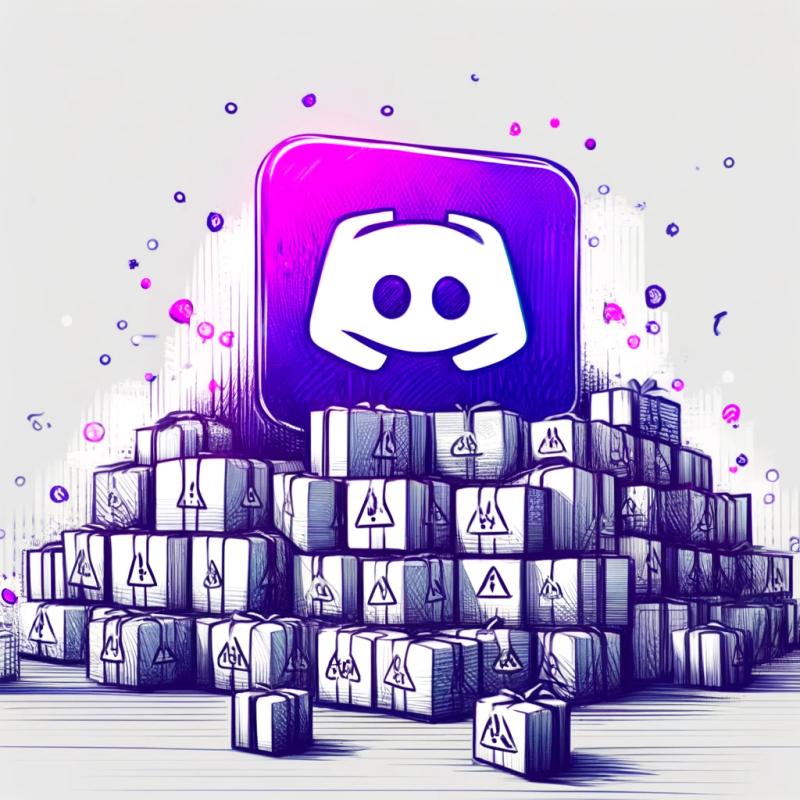
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
gulp-babel
Advanced tools
Changelog
8.0.0-beta.1 (2018-01-26)
gulp-util
(#137)CHANGELOG.md
, update .gitignore
, license year, update dependencies,
add lock files, add npm badge, mention gulp-babel@next
.Readme
This readme is for gulp-babel v8 + Babel v7 Check the 7.x branch for docs with Babel v6 usage
Use next generation JavaScript, today, with Babel
Issues with the output should be reported on the Babel issue tracker.
Install gulp-babel
if you want to get the pre-release of the next version of gulp-babel
.
# Babel 7
$ npm install --save-dev gulp-babel @babel/core @babel/preset-env
# Babel 6
$ npm install --save-dev gulp-babel@7 babel-core babel-preset-env
const gulp = require('gulp');
const babel = require('gulp-babel');
gulp.task('default', () =>
gulp.src('src/app.js')
.pipe(babel({
presets: ['@babel/env']
}))
.pipe(gulp.dest('dist'))
);
See the Babel options, except for sourceMap
and filename
which is handled for you.
Use gulp-sourcemaps like this:
const gulp = require('gulp');
const sourcemaps = require('gulp-sourcemaps');
const babel = require('gulp-babel');
const concat = require('gulp-concat');
gulp.task('default', () =>
gulp.src('src/**/*.js')
.pipe(sourcemaps.init())
.pipe(babel({
presets: ['@babel/env']
}))
.pipe(concat('all.js'))
.pipe(sourcemaps.write('.'))
.pipe(gulp.dest('dist'))
);
Files in the stream are annotated with a babel
property, which contains the metadata from babel.transform()
.
const gulp = require('gulp');
const babel = require('gulp-babel');
const through = require('through2');
function logBabelMetadata() {
return through.obj((file, enc, cb) => {
console.log(file.babel.test); // 'metadata'
cb(null, file);
});
}
gulp.task('default', () =>
gulp.src('src/**/*.js')
.pipe(babel({
// plugin that sets some metadata
plugins: [{
post(file) {
file.metadata.test = 'metadata';
}
}]
}))
.pipe(logBabelMetadata())
)
If you're attempting to use features such as generators, you'll need to add transform-runtime
as a plugin, to include the Babel runtime. Otherwise, you'll receive the error: regeneratorRuntime is not defined
.
Install the runtime:
$ npm install --save-dev @babel/plugin-transform-runtime
$ npm install --save @babel/runtime
Use it as plugin:
const gulp = require('gulp');
const babel = require('gulp-babel');
gulp.task('default', () =>
gulp.src('src/app.js')
.pipe(babel({
plugins: ['@babel/transform-runtime']
}))
.pipe(gulp.dest('dist'))
);
MIT © Sindre Sorhus
FAQs
Use next generation JavaScript, today
The npm package gulp-babel receives a total of 142,578 weekly downloads. As such, gulp-babel popularity was classified as popular.
We found that gulp-babel demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.