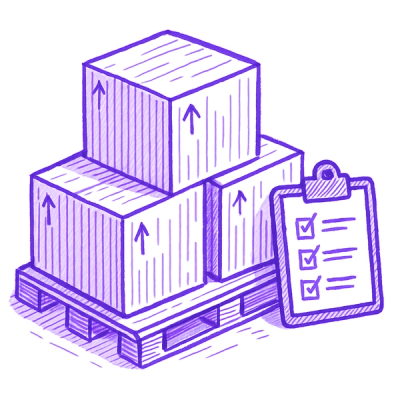
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
hadoop-streaming-utils
Advanced tools
A set of functions to allow you write hadoop jobs easily.
// mapper.js (count word example)
var hadoopUtils = require('hadoop-streaming-utils');
hadoopUtils.iterateJsonLines(function(line) {
var words = line.split(/\s+/);
words.forEach(function(word) {
// using emitJson instead of emit allows to preserve variable type
hadoopUtils.emitJson(word, 1);
});
});
// reducer.js
var hadoopUtils = require('hadoop-streaming-utils');
hadoopUtils.iterateKeysWithGroupedJsonValues(function(word, counts) {
var totalCount = 0;
counts.forEach(function(cnt) {
// no need to parseInt because in reducer we use "emitJson"
totalCount += cnt;
});
hadoopUtils.emitJson(word, totalCount);
});
// Run (emulate hadoop-streaming behaviour)
cat file | node mapper.js | sort -k1,1 | node reducer.js
See more examples in "examples" folder.
This modules contains a set of utils to read and process data line by line. So, next line will be read only after finishing processing the previous one. It is easy when your callback is synchronous. When your callback is asynchronous you should return a promise from it. Moreover, every iterating function returns a promise which will be resolved after finishing processing all lines.
iterateJsonLines
Will read input line by line and will apply JSON.parse to each line.
hadoopUtils.iterateJsonLines(function(data) { });
iterateKeysWithJsonValues
hadoopUtils.iterateKeysWithJsonValues(function(key, value) { });
iterateKeysWithGroupedJsonValues
hadoopUtils.iterateKeysWithGroupedJsonValues(function(key, values) { });
emitJson
Serializes data to JSON and emits it.
hadoopUtils.emitJson(key, data);
iterateLines
Will read and process input line by line.
hadoopUtils.iterateLines(function(data) { });
iterateKeysWithValues
hadoopUtils.iterateKeysWithValues(function(key, value) { });
iterateKeysWithGroupedValues
hadoopUtils.iterateKeysWithGroupedValues(function(key, values) { });
emit
Emits key and value.
hadoopUtils.emitJson(key, value);
incrementCounter
Updates hadoop counter.
hadoopUtils.incrementCounter(group, counter, amount);
When your callback is async you should return promise from it. So, iterating function will wait until promise is resolved. Moreover, every iterating function returns a promise which will be resolved when all lines were processed.
Usage example
var Promise = require('bluebird');
var streamingUtils = require('hadoop-streaming-utils');
streamingUtils.iterateLines(function(line) {
return new Promise(function(resolve, reject) {
asyncSplit(line, function(err, words) {
resolve(words);
});
}).then(function(words) {
words.forEach(function(word) {
streamingUtils.emitJson(word, 1);
});
});
}).then(function() {
process.exit();
}).catch(console.error);
function asyncSplit(line, callback) {
var words = line.split(/\s+/);
setTimeout(function() {
callback(null, words);
}, 500);
}
koorchik (Viktor Turskyi)
FAQs
Hadoop streaming utils for NodeJS
The npm package hadoop-streaming-utils receives a total of 6 weekly downloads. As such, hadoop-streaming-utils popularity was classified as not popular.
We found that hadoop-streaming-utils demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.