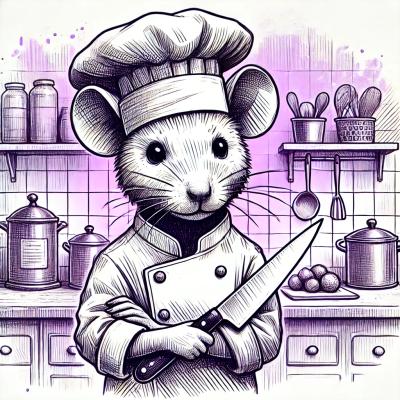
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Hammer.js is a popular JavaScript library for handling touch gestures. It provides a simple way to recognize gestures made by touch, mouse, and pointer events, enabling developers to easily implement interactive features such as swiping, pinching, rotating, and pressing on web pages.
Swipe Gesture
This code sample demonstrates how to detect left and right swipe gestures on an element. It initializes Hammer.js on a target element and listens for 'swipeleft' and 'swiperight' events.
var myElement = document.getElementById('myElement');
var mc = new Hammer(myElement);
mc.on('swipeleft swiperight', function(ev) {
console.log(ev.type + " gesture detected.");
});
Pinch Gesture
This example shows how to enable and detect pinch gestures. It sets up pinch gesture recognition on an element and logs the scale of the pinch gesture.
var myElement = document.getElementById('myElement');
var mc = new Hammer(myElement);
mc.get('pinch').set({ enable: true });
mc.on('pinch', function(ev) {
console.log("Pinch gesture detected with scale: " + ev.scale);
});
Rotate Gesture
This code snippet enables rotation gesture detection on an element. When a rotate gesture is detected, it logs the rotation angle.
var myElement = document.getElementById('myElement');
var mc = new Hammer(myElement);
mc.get('rotate').set({ enable: true });
mc.on('rotate', function(ev) {
console.log("Rotate gesture detected with angle: " + ev.rotation);
});
Interact.js is a JavaScript library for drag and drop, resizing, and multi-touch gestures with inertia and snapping. It's similar to Hammer.js in providing touch gesture support but also offers additional functionalities like drag-and-drop and element resizing.
TouchSwipe is a jQuery plugin for handling touch events like swipe, tap, pinch, and more. It's similar to Hammer.js in terms of providing touch gesture recognition but is specifically designed for use with jQuery.
Visit hammerjs.github.io for detailed documentation.
// get a reference to an element
var stage = document.getElementById('stage');
// create a manager for that element
var mc = new Hammer.Manager(stage);
// create a recognizer
var Rotate = new Hammer.Rotate();
// add the recognizer
mc.add(Rotate);
// subscribe to events
mc.on('rotate', function(e) {
// do something cool
var rotation = Math.round(e.rotation);
stage.style.transform = 'rotate('+rotation+'deg)';
});
An advanced demo is available here: http://codepen.io/runspired/full/ZQBGWd/
Read the contributing guidelines.
For PRs.
You can get the pre-build versions from the Hammer.js website, or do this by yourself running
npm install -g grunt-cli && npm install && grunt build
2.0.8, 2016-04-22
FAQs
A javascript library for multi-touch gestures
The npm package hammerjs receives a total of 430,674 weekly downloads. As such, hammerjs popularity was classified as popular.
We found that hammerjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.