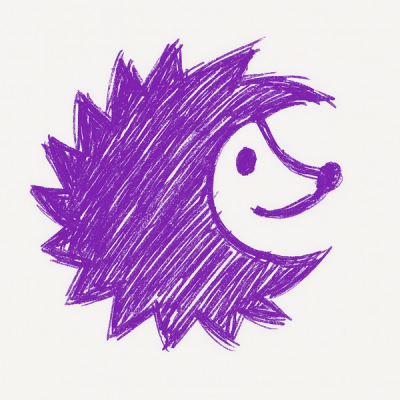
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
hapi-maily-widgets
Advanced tools
Email MJML widgets used by hapi-maily and other email services
A collection of email widgets for use with MJML
Install Widgets
yarn add hapi-maily-widgets
Usage
import React from 'react';
import { renderToStaticMarkup } from 'react-dom/server';
import { Footer, renderMJML } from 'header';
const emailComponent = renderMJML(<Footer />);
const staticEmail = renderToStaticMarkup(emailComponent);
console.log(staticEmail); // Static HTML email
Preview available widgets in react storybook, each story item
needs to be served through renderMJML
in order to convert
mjml into valid HTML markup.
You are able to customize the look of the mail components by passing a
theme property to the renderMJML
method.
The structure of the theme should be as follows
const theme = {
colors: {
primary: '#E91E63'
// you can use additional colors
// below, so long as it is
// string: 'HEX'
},
headerImage: {
src: 'url to hosted image',
alt: 'alt text describing image'
}
}
Then you can simply call the renderMJML method with your components.
import React from 'react';
import { renderToStaticMarkup } from 'react-dom/server';
import { Button, renderMJML } from 'header';
const theme = {
colors: {
primary: '#03A9F4',
secondary: '#009688',
paul: '#8BC34A'
}
};
const buttonComponent = (
<Button
url="http://google.com"
buttonType="paul"
>
Hi Im Paul!
</Button>
)
const emailComponent = renderMJML(buttonComponent, theme);
const staticEmail = renderToStaticMarkup(emailComponent);
console.log(staticEmail); // Static Themed HTML email
yarn start
Generates the email widgets in storybook mode. Open http://localhost:9009 to view it in the browser.
yarn lint
Runs the linting command, should be done before pull requests are made. This application follows the airbnb linting styleguide with minor changes.
FAQs
Email MJML widgets used by hapi-maily and other email services
The npm package hapi-maily-widgets receives a total of 24 weekly downloads. As such, hapi-maily-widgets popularity was classified as not popular.
We found that hapi-maily-widgets demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.