Comparing version 0.2.7 to 0.2.8
@@ -223,10 +223,10 @@ (function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s})({1:[function(require,module,exports){ | ||
//----------------------------------- | ||
init: function(keyFields, callback, options) { | ||
init: function(keyFields, callback, options) { | ||
keyFields = keyFields instanceof Array ? keyFields : [keyFields]; | ||
this._map = {}; | ||
this._list = []; | ||
this.callback = callback; | ||
this._map = {}; | ||
this._list = []; | ||
this.callback = callback; | ||
this.keyFields = keyFields; | ||
this.keyFields = keyFields; | ||
@@ -239,6 +239,6 @@ this.isHashArray = true; | ||
if (callback) { | ||
callback('construct'); | ||
} | ||
}, | ||
if (callback) { | ||
callback('construct'); | ||
} | ||
}, | ||
//----------------------------------- | ||
@@ -249,34 +249,34 @@ // add() | ||
var needsDupCheck = false; | ||
for (var key in this.keyFields) { | ||
key = this.keyFields[key]; | ||
var inst = this.objectAt(obj, key); | ||
if (inst) { | ||
if (this._map[inst]) { | ||
for (var key in this.keyFields) { | ||
key = this.keyFields[key]; | ||
var inst = this.objectAt(obj, key); | ||
if (inst) { | ||
if (this._map[inst]) { | ||
if (this.options.ignoreDuplicates) | ||
return; | ||
if (this._map[inst].indexOf(obj) != -1) { | ||
// Cannot add the same item twice | ||
if (this._map[inst].indexOf(obj) != -1) { | ||
// Cannot add the same item twice | ||
needsDupCheck = true; | ||
continue; | ||
} | ||
this._map[inst].push(obj); | ||
} | ||
continue; | ||
} | ||
this._map[inst].push(obj); | ||
} | ||
else this._map[inst] = [obj]; | ||
} | ||
} | ||
} | ||
} | ||
if (!needsDupCheck || this._list.indexOf(obj) == -1) | ||
this._list.push(obj); | ||
this._list.push(obj); | ||
}, | ||
add: function() { | ||
for (var i = 0; i < arguments.length; i++) { | ||
this.addOne(arguments[i]); | ||
} | ||
add: function() { | ||
for (var i = 0; i < arguments.length; i++) { | ||
this.addOne(arguments[i]); | ||
} | ||
if (this.callback) { | ||
this.callback('add', Array.prototype.slice.call(arguments, 0)); | ||
} | ||
if (this.callback) { | ||
this.callback('add', Array.prototype.slice.call(arguments, 0)); | ||
} | ||
return this; | ||
}, | ||
}, | ||
addAll: function (arr) { | ||
@@ -292,13 +292,13 @@ if (arr.length < 100) | ||
}, | ||
addMap: function(key, obj) { | ||
this._map[key] = obj; | ||
if (this.callback) { | ||
this.callback('addMap', { | ||
key: key, | ||
obj: obj | ||
}); | ||
} | ||
addMap: function(key, obj) { | ||
this._map[key] = obj; | ||
if (this.callback) { | ||
this.callback('addMap', { | ||
key: key, | ||
obj: obj | ||
}); | ||
} | ||
return this; | ||
}, | ||
}, | ||
//----------------------------------- | ||
@@ -347,5 +347,5 @@ // Intersection, union, etc. | ||
//----------------------------------- | ||
get: function(key) { | ||
return (!(this._map[key] instanceof Array) || this._map[key].length != 1) ? this._map[key] : this._map[key][0]; | ||
}, | ||
get: function(key) { | ||
return (!(this._map[key] instanceof Array) || this._map[key].length != 1) ? this._map[key] : this._map[key][0]; | ||
}, | ||
getAll: function(keys) { | ||
@@ -400,5 +400,5 @@ keys = keys instanceof Array ? keys : [keys]; | ||
//----------------------------------- | ||
has: function(key) { | ||
return this._map.hasOwnProperty(key); | ||
}, | ||
has: function(key) { | ||
return this._map.hasOwnProperty(key); | ||
}, | ||
collides: function (item) { | ||
@@ -411,93 +411,93 @@ for (var k in this.keyFields) | ||
}, | ||
hasMultiple: function(key) { | ||
return this._map[key] instanceof Array; | ||
}, | ||
hasMultiple: function(key) { | ||
return this._map[key] instanceof Array; | ||
}, | ||
//----------------------------------- | ||
// Removal | ||
//----------------------------------- | ||
removeByKey: function() { | ||
var removed = []; | ||
for (var i = 0; i < arguments.length; i++) { | ||
var key = arguments[i]; | ||
var items = this._map[key].concat(); | ||
if (items) { | ||
removed = removed.concat(items); | ||
for (var j in items) { | ||
var item = items[j]; | ||
for (var ix in this.keyFields) { | ||
var key2 = this.objectAt(item, this.keyFields[ix]); | ||
if (key2 && this._map[key2]) { | ||
var ix = this._map[key2].indexOf(item); | ||
if (ix != -1) { | ||
this._map[key2].splice(ix, 1); | ||
} | ||
removeByKey: function() { | ||
var removed = []; | ||
for (var i = 0; i < arguments.length; i++) { | ||
var key = arguments[i]; | ||
var items = this._map[key].concat(); | ||
if (items) { | ||
removed = removed.concat(items); | ||
for (var j in items) { | ||
var item = items[j]; | ||
for (var ix in this.keyFields) { | ||
var key2 = this.objectAt(item, this.keyFields[ix]); | ||
if (key2 && this._map[key2]) { | ||
var ix = this._map[key2].indexOf(item); | ||
if (ix != -1) { | ||
this._map[key2].splice(ix, 1); | ||
} | ||
if (this._map[key2].length == 0) | ||
delete this._map[key2]; | ||
} | ||
} | ||
if (this._map[key2].length == 0) | ||
delete this._map[key2]; | ||
} | ||
} | ||
this._list.splice(this._list.indexOf(item), 1); | ||
} | ||
} | ||
delete this._map[key]; | ||
} | ||
this._list.splice(this._list.indexOf(item), 1); | ||
} | ||
} | ||
delete this._map[key]; | ||
} | ||
if (this.callback) { | ||
this.callback('removeByKey', removed); | ||
} | ||
if (this.callback) { | ||
this.callback('removeByKey', removed); | ||
} | ||
return this; | ||
}, | ||
remove: function() { | ||
for (var i = 0; i < arguments.length; i++) { | ||
var item = arguments[i]; | ||
for (var ix in this.keyFields) { | ||
var key = this.objectAt(item, this.keyFields[ix]); | ||
if (key) { | ||
var ix = this._map[key].indexOf(item); | ||
if (ix != -1) | ||
this._map[key].splice(ix, 1); | ||
}, | ||
remove: function() { | ||
for (var i = 0; i < arguments.length; i++) { | ||
var item = arguments[i]; | ||
for (var ix in this.keyFields) { | ||
var key = this.objectAt(item, this.keyFields[ix]); | ||
if (key) { | ||
var ix = this._map[key].indexOf(item); | ||
if (ix != -1) | ||
this._map[key].splice(ix, 1); | ||
if (this._map[key].length == 0) | ||
delete this._map[key]; | ||
} | ||
} | ||
if (this._map[key].length == 0) | ||
delete this._map[key]; | ||
} | ||
} | ||
this._list.splice(this._list.indexOf(item), 1); | ||
} | ||
this._list.splice(this._list.indexOf(item), 1); | ||
} | ||
if (this.callback) { | ||
this.callback('remove', arguments); | ||
} | ||
if (this.callback) { | ||
this.callback('remove', arguments); | ||
} | ||
return this; | ||
}, | ||
removeAll: function() { | ||
var old = this._list.concat(); | ||
this._map = {}; | ||
this._list = []; | ||
}, | ||
removeAll: function() { | ||
var old = this._list.concat(); | ||
this._map = {}; | ||
this._list = []; | ||
if (this.callback) { | ||
this.callback('remove', old); | ||
} | ||
if (this.callback) { | ||
this.callback('remove', old); | ||
} | ||
return this; | ||
}, | ||
}, | ||
//----------------------------------- | ||
// Utility | ||
//----------------------------------- | ||
objectAt: function(obj, path) { | ||
if (typeof path === 'string') { | ||
return obj[path]; | ||
} | ||
objectAt: function(obj, path) { | ||
if (typeof path === 'string') { | ||
return obj[path]; | ||
} | ||
var dup = path.concat(); | ||
// else assume array. | ||
while (dup.length && obj) { | ||
obj = obj[dup.shift()]; | ||
} | ||
var dup = path.concat(); | ||
// else assume array. | ||
while (dup.length && obj) { | ||
obj = obj[dup.shift()]; | ||
} | ||
return obj; | ||
}, | ||
return obj; | ||
}, | ||
//----------------------------------- | ||
@@ -530,8 +530,8 @@ // Iteration | ||
//----------------------------------- | ||
clone: function(callback, ignoreItems) { | ||
var n = new HashArray(this.keyFields.concat(), callback ? callback : this.callback); | ||
clone: function(callback, ignoreItems) { | ||
var n = new HashArray(this.keyFields.concat(), callback ? callback : this.callback); | ||
if (!ignoreItems) | ||
n.add.apply(n, this.all.concat()); | ||
return n; | ||
}, | ||
return n; | ||
}, | ||
//----------------------------------- | ||
@@ -595,11 +595,11 @@ // Mathematical | ||
Object.defineProperty(HashArray.prototype, 'all', { | ||
get: function () { | ||
return this._list; | ||
} | ||
get: function () { | ||
return this._list; | ||
} | ||
}); | ||
Object.defineProperty(HashArray.prototype, 'map', { | ||
get: function () { | ||
return this._map; | ||
} | ||
get: function () { | ||
return this._map; | ||
} | ||
}); | ||
@@ -613,3 +613,3 @@ | ||
if (typeof window !== 'undefined') | ||
window.HashArray = HashArray; | ||
window.HashArray = HashArray; | ||
},{"jclass":1}]},{},[2]); |
@@ -6,3 +6,3 @@ { | ||
"description": "A data structure that combines a hash and an array for fast dictionary lookup and traversal by complex keys.", | ||
"version": "0.2.7", | ||
"version": "0.2.8", | ||
"main": "index.js", | ||
@@ -28,2 +28,2 @@ "url": "https://github.com/joshjung/hash-array", | ||
] | ||
} | ||
} |
@@ -52,2 +52,19 @@ 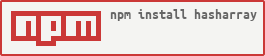 | ||
**`callback`** | ||
A callback function can be specified to monitor changes to the HashArray as they occur: | ||
var ha = new HashArray('someKey', function(type, whatChanged) { | ||
// type will be 'add', 'addMap', 'remove', 'removeByKey', or 'construct' | ||
// whatChanged will be the items that were changed | ||
}); | ||
**`options`** | ||
{ | ||
ignoreDuplicates: false; // When true, any attempt to add items that collide | ||
// with any items in the HashArray will be ignored. | ||
// default is false. | ||
} | ||
Insertion | ||
@@ -54,0 +71,0 @@ --------- |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
31324
396