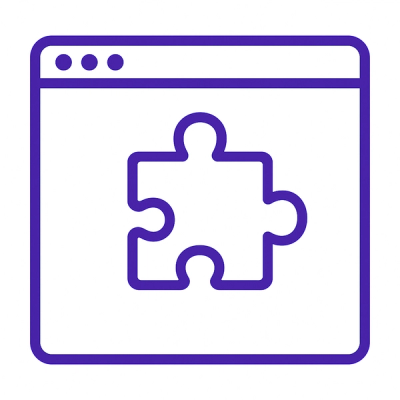
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
headless-currency-input
Advanced tools
Headless Currency Input is a component to format currency input in an elegant way.
A customizable and feature-rich React component for handling currency input. It supports multiple currencies, formatting, and provides a seamless user experience for handling currency values.
👋 Hello there! Follow me @danestves or visit danestves.com for more cool projects like this one.
npm install headless-currency-input
# or
yarn add headless-currency-input
# or
pnpm add headless-currency-input
# or
bun add headless-currency-input
import React from 'react';
import { CurrencyInput } from 'headless-currency-input';
const App = () => {
const [values, setValue] = React.useState(245698189);
return (
<CurrencyInput
value={value}
onValueChange={(values) => {
console.log(values);
/**
* Will output:
*
* {
* formattedValue: "$ 2,456,981.89",
* value: '2456981.89',
* floatValue: 2456981.89,
* }
*/
}}
currency="USD"
locale="en-US"
/>
);
};
currency
default: USD
The currency to use. Must be a valid currency code based on ISO 4217.
locale
default: en
The locale to use. Must be a valid locale based on ISO 639-1.
text | input
default: input
If value is input
, it renders an input element where formatting happens as you type characters. If value is text, it renders formatted text
in a span tag.
import { CurrencyInput } from 'headless-currency-input';
<CurrencyInput displayType="input" value={110} />;
<CurrencyInput displayType="text" value={110} />;
[!NOTE] More info https://s-yadav.github.io/react-number-format/docs/props#displaytype-text--input
elm => void
default: null
Method to get reference of input, span (based on displayType prop) or the customInput's reference.
import { CurrencyInput } from 'headless-currency-input';
import { useRef } from 'react';
export default function App() {
let ref = useRef();
return <CurrencyInput getInputRef={ref} />;
}
[!NOTE] More info https://s-yadav.github.io/react-number-format/docs/props#getinputref-elm--void
(values) => boolean
default: undefined
A checker function to validate the input value. If this function returns false, the onChange method will not get triggered and the input value will not change.
import { CurrencyInput } from 'headless-currency-input';
const MAX_LIMIT = 1000;
<CurrencyInput
value={11}
isAllowed={(values) => {
const { floatValue } = values;
return floatValue < MAX_LIMIT;
}}
/>;
[!NOTE] More info https://s-yadav.github.io/react-number-format/docs/props#isallowed-values--boolean
(values, sourceInfo) => {}
default: undefined
This handler provides access to any values changes in the input field and is triggered only when a prop changes or the user input changes. It provides two arguments namely the valueObject as the first and the sourceInfo as the second. The valueObject parameter contains the formattedValue
, value
and the floatValue
of the given input field. The sourceInfo contains the event
Object and a source
key which indicates whether the triggered change is due to an event or a prop change. This is particularly useful in identify whether the change is user driven or is an uncontrolled change due to any prop value being updated.
import { CurrencyInput } from 'headless-currency-input';
<CurrencyInput
value={1234}
onValueChange={(values, sourceInfo) => {
console.log(values, sourceInfo);
}}
/>;
[!NOTE] More info https://s-yadav.github.io/react-number-format/docs/props#onvaluechange-values-sourceinfo--
(formattedValue, customProps) => React Element
default: undefined
A renderText method useful if you want to render formattedValue in different element other than span. It also returns the custom props that are added to the component which can allow passing down props to the rendered element.
import { CurrencyInput } from 'headless-currency-input';
<CurrencyInput
value={1231231}
displayType="text"
renderText={(value) => <b>{value}</b>}
/>;
[!NOTE] More info https://s-yadav.github.io/react-number-format/docs/props#rendertext-formattedvalue-customprops--react-element
[!TIP] Other than this it accepts all the props which can be given to a input or span based on displayType you selected.
FAQs
Headless Currency Input is a component to format currency input in an elegant way.
The npm package headless-currency-input receives a total of 44 weekly downloads. As such, headless-currency-input popularity was classified as not popular.
We found that headless-currency-input demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.