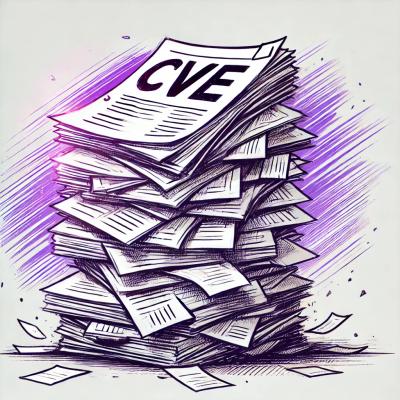
Security News
CISA Rebuffs Funding Concerns as CVE Foundation Draws Criticism
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Simple Binary Heap implementation. Supports pop, push, peek, heapify and mutateTop operations.
Heap is a data structure that holds a collection of comparable objects and allows to extract min(max) object in a time logarithmic of the number of items in the heap.
npm i --save heappy
import Heap from 'heappy';
// default parameters
const minHeap = new Heap([5, 19, 33, 4]);
minHeap.push(5);
minHeap.push(19);
minHeap.push(33);
minHeap.push(4);
minHeap.push(3);
minHeap.pop(); // 3
minHeap.pop(); // 4
minHeap.peek(); // 5
minHeap.mutateTop((item) => item + 10); // 15
minHeap.getSize(); // 3
// array passed as the first parameter will be heapified
const maxHeap = new Heap([5, 19, 33, 4], (e1, e2) => e1 - e2);
maxHeap.pop(); // 33
new Heap(items?: any[], compare?: (item, nextItem) => number);
new Heap([...items])
if you need to avoid mutation.heap.getSize(): number;
Returns the size of the heap.
heap.push(item: any);
Adds an item to the heap. Preserves the heap condition.
heap.pop(): any;
Returns the item at the top of the heap and removes it from the heap. Preserves the heap condition.
heap.peek(): any;
Returns the item at the top of the heap. Keeps the item in the heap.
heap.mutateTop(mutator: (item: any) => any): any;
Applies the mutator to the top item from the heap and returns mutated item. Preserves heap condition.
Note: same opeartion could be done by pop
ing an item, creating a new item and push
ing it back in the heap. However, it would require an unnecessary extra log(N)
-operation (sink new top item after popping + float new item after pushing).
FAQs
Simple Heap implementation with mutateTop method
The npm package heappy receives a total of 0 weekly downloads. As such, heappy popularity was classified as not popular.
We found that heappy demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.
Product
Module Reachability filters out unreachable CVEs so you can focus on vulnerabilities that actually matter to your application.