hermetrics
Advanced tools
Comparing version 1.1.11 to 1.2.11
@@ -6,18 +6,18 @@ import Jaro from './jaro'; | ||
/** | ||
* Jaro Winkler Similarity | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param p | ||
*/ | ||
* Jaro Winkler Similarity | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param p | ||
*/ | ||
similarity(source: string, target: string, { insertionCost, deletionCost, substitutionCost, lambdaCost, roCost }?: JaroCostOptions): number; | ||
/** | ||
* Jaro Winkler distance | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param p | ||
*/ | ||
* Jaro Winkler distance | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param p | ||
*/ | ||
distance(source: string, target: string, { insertionCost, deletionCost, substitutionCost, lambdaCost, roCost }?: JaroCostOptions): number; | ||
} | ||
export default JaroWinkler; |
@@ -8,16 +8,16 @@ "use strict"; | ||
class JaroWinkler extends jaro_1.default { | ||
constructor(name = 'Jaro_Winkler') { | ||
constructor(name = 'JaroWinkler') { | ||
super(name); | ||
} | ||
/** | ||
* Jaro Winkler Similarity | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param p | ||
*/ | ||
* Jaro Winkler Similarity | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param p | ||
*/ | ||
similarity(source, target, { insertionCost, deletionCost, substitutionCost, lambdaCost, roCost } = {}) { | ||
const p = roCost !== null && roCost !== void 0 ? roCost : 0.1; | ||
if (!(0 <= p && p <= 0.25)) { | ||
new Error("The p parameter must be between 0 and 0.25"); | ||
if (!(p >= 0 && p <= 0.25)) { | ||
throw new Error('The p parameter must be between 0 and 0.25'); | ||
} | ||
@@ -27,3 +27,3 @@ let l = 0; | ||
for (let i = 0; i < maxL; i++) { | ||
if (source[i] != target[i]) | ||
if (source[i] !== target[i]) | ||
break; | ||
@@ -36,8 +36,8 @@ l++; | ||
/** | ||
* Jaro Winkler distance | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param p | ||
*/ | ||
* Jaro Winkler distance | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param p | ||
*/ | ||
distance(source, target, { insertionCost, deletionCost, substitutionCost, lambdaCost, roCost } = {}) { | ||
@@ -44,0 +44,0 @@ return 1 - this.similarity(source, target, { insertionCost, deletionCost, substitutionCost, lambdaCost, roCost }); |
@@ -20,8 +20,8 @@ "use strict"; | ||
const targetLength = target.length; | ||
if (sourceLength == 0 && targetLength == 0) { | ||
if (sourceLength === 0 && targetLength === 0) { | ||
return 1; | ||
} | ||
const matchDistance = Math.max(sourceLength, targetLength) / 2 - 1; | ||
let sourceMatches = new Array(sourceLength); | ||
let targetMatches = new Array(targetLength); | ||
const sourceMatches = new Array(sourceLength); | ||
const targetMatches = new Array(targetLength); | ||
let matches = 0; | ||
@@ -45,3 +45,3 @@ let transpositions = 0; | ||
} | ||
if (matches == 0) | ||
if (matches === 0) | ||
return 0; | ||
@@ -54,3 +54,3 @@ let k = 0; | ||
k++; | ||
if (source[i] != target[k]) | ||
if (source[i] !== target[k]) | ||
transpositions++; | ||
@@ -57,0 +57,0 @@ k++; |
@@ -6,4 +6,4 @@ import Metric from './metric'; | ||
distance(source: string, target: string, { deletionCost, insertionCost, substitutionCost }?: LevenshteinCostOptions): number; | ||
maxDistance(source: string, target: string, { deletionCost, insertionCost, substitutionCost }?: LevenshteinCostOptions): number; | ||
maxDistance(source: string, target: string, { deletionCost, insertionCost, substitutionCost, cost }?: LevenshteinCostOptions): number; | ||
} | ||
export default Levenshtein; |
@@ -37,8 +37,8 @@ "use strict"; | ||
} | ||
maxDistance(source, target, { deletionCost, insertionCost, substitutionCost } = {}) { | ||
maxDistance(source, target, { deletionCost, insertionCost, substitutionCost, cost = 1 } = {}) { | ||
const sourceLength = source.length; | ||
const targetLength = target.length; | ||
const delCost = deletionCost !== null && deletionCost !== void 0 ? deletionCost : 1; | ||
const insCost = insertionCost !== null && insertionCost !== void 0 ? insertionCost : 1; | ||
const subCost = substitutionCost !== null && substitutionCost !== void 0 ? substitutionCost : 1; | ||
const delCost = deletionCost !== null && deletionCost !== void 0 ? deletionCost : cost; | ||
const insCost = insertionCost !== null && insertionCost !== void 0 ? insertionCost : cost; | ||
const subCost = substitutionCost !== null && substitutionCost !== void 0 ? substitutionCost : cost; | ||
const maxDel = Math.max(sourceLength - targetLength, 0); | ||
@@ -45,0 +45,0 @@ const maxIns = Math.max(targetLength - sourceLength, 0); |
@@ -19,3 +19,8 @@ import LevenshteinCostOptions from '../interfaces/levenshtein-opts.interface'; | ||
normalizedDistance(source: string, target: string, { deletionCost, insertionCost, substitutionCost }?: LevenshteinCostOptions): number; | ||
/** | ||
* similarity | ||
*/ | ||
similarity(source: string, target: string, costs?: LevenshteinCostOptions): number; | ||
get name(): string; | ||
} | ||
export default Metric; |
@@ -44,3 +44,12 @@ "use strict"; | ||
} | ||
/** | ||
* similarity | ||
*/ | ||
similarity(source, target, costs = {}) { | ||
return 1 - this.normalizedDistance(source, target, costs); | ||
} | ||
get name() { | ||
return this._name; | ||
} | ||
} | ||
exports.default = Metric; |
@@ -5,2 +5,7 @@ import Levenshtein from './hermetrics/levenshtein'; | ||
import JaroWinkler from './hermetrics/jaro_winkler'; | ||
export { Levenshtein, Metric, Jaro, JaroWinkler }; | ||
import MetricComparator from './hermetrics/metric-comparator'; | ||
import Jaccard from './hermetrics/jaccard'; | ||
import Hamming from './hermetrics/hamming'; | ||
import DamerauLevenshtein from './hermetrics/damerau_levenshtein'; | ||
import Dice from './hermetrics/dice'; | ||
export { Levenshtein, Metric, Jaro, JaroWinkler, MetricComparator, Jaccard, Hamming, DamerauLevenshtein, Dice }; |
@@ -14,1 +14,11 @@ "use strict"; | ||
exports.JaroWinkler = jaro_winkler_1.default; | ||
const metric_comparator_1 = __importDefault(require("./hermetrics/metric-comparator")); | ||
exports.MetricComparator = metric_comparator_1.default; | ||
const jaccard_1 = __importDefault(require("./hermetrics/jaccard")); | ||
exports.Jaccard = jaccard_1.default; | ||
const hamming_1 = __importDefault(require("./hermetrics/hamming")); | ||
exports.Hamming = hamming_1.default; | ||
const damerau_levenshtein_1 = __importDefault(require("./hermetrics/damerau_levenshtein")); | ||
exports.DamerauLevenshtein = damerau_levenshtein_1.default; | ||
const dice_1 = __importDefault(require("./hermetrics/dice")); | ||
exports.Dice = dice_1.default; |
@@ -5,2 +5,4 @@ export default interface LevenshteinCostOptions { | ||
substitutionCost?: number; | ||
transpositionCost?: number; | ||
cost?: number; | ||
} |
{ | ||
"name": "hermetrics", | ||
"version": "1.1.11", | ||
"version": "1.2.11", | ||
"description": "Javascript version of hermetrics.py", | ||
@@ -11,2 +11,5 @@ "main": "dist/index.js", | ||
}, | ||
"publishConfig": { | ||
"registery": "https://npm.pkg.github.com/" | ||
}, | ||
"keywords": [ | ||
@@ -16,3 +19,11 @@ "hermetrics", | ||
"similarity metric", | ||
"distance metric" | ||
"distance metric", | ||
"levenshtein", | ||
"jaro", | ||
"jaro-winkler", | ||
"hamming", | ||
"osa", | ||
"damerau-levenshtein", | ||
"jaccard", | ||
"dice" | ||
], | ||
@@ -54,5 +65,5 @@ "scripts": { | ||
"hooks": { | ||
"pre-push": "npm test" | ||
"pre-push": "npm test && npm run lint --fix" | ||
} | ||
} | ||
} |
@@ -20,8 +20,8 @@ 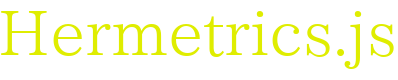 | ||
* [Jaro-Winkler](#jaro-winkler) | ||
* Hamming (work in progress) | ||
* [Jaccard](#jaccard) | ||
* [Metric comparator](#mc) | ||
* [Hamming](#hamming) | ||
* [Damerau-Levenshtein](#dl) | ||
* [Dice](#dice) | ||
* OSA (work in progress) | ||
* Damerau-Levenshtein (work in progress) | ||
* Jaccard (work in progress) | ||
* Dice (work in progress) | ||
* Metric comparator (work in progress) | ||
@@ -58,4 +58,4 @@ # Installation <a name="installation"></a> | ||
deletionCost: 3, | ||
substitutionCost: 2, | ||
deletionCost: 5 | ||
insertionCost: 5, | ||
substitutionCost: 2 | ||
}; | ||
@@ -75,3 +75,3 @@ | ||
A metric has three main methods distance, normalizeDistance and similarity. In general the distance method computes the absolute distance between two strings, whereas normalizeDistance can be used to scale the distance to a particular range, usually (0,1), and the similarity method being normally defined as (1-normalizeDistance). | ||
A metric has three main methods: distance, normalizeDistance and similarity. In general the distance method computes the absolute distance between two strings, whereas normalizeDistance can be used to scale the distance to a particular range, usually (0,1), and the similarity method being normally defined as (1-normalizeDistance). | ||
@@ -82,4 +82,52 @@ The normalization of the distance can be customized overriding the auxiliary methods for its computation. Those methods are maxDistance, minDistance and normalize. | ||
Metric is a base class that can receive as arguments an metric name, and contains six specific functions to be used as methods for the metric being implemented. | ||
Metric is a base class that contains six specific functions to be used as methods for the metric being implemented. | ||
If you want customize any function, for now, there are two available ways: | ||
You could modify the class `prototype` | ||
``` javascript | ||
const { Levenshtein } = require('hermetrics'); | ||
function foo() { | ||
console.log('foo'); | ||
} | ||
Levenshtein.prototype.distance = foo; | ||
// or | ||
Levenshtein.prototype.distance = function() { | ||
console.log('foo'); | ||
} | ||
const levenshtein = new Levenshtein(); | ||
levenshtein.distance() // foo | ||
``` | ||
You could extend the `Metric` base class | ||
```javascript | ||
const { Metric } = require('hermetrics'); | ||
class MyAwesomeMetric extends Metric { | ||
constructor(name = 'MyAwesomeMetric') { | ||
super(name); | ||
} | ||
distance() { | ||
console.log('bar'); | ||
} | ||
} | ||
const myAwesomeMetric = new MyAwesomeMetric(); | ||
myAwesomeMetric.distance(); //bar | ||
``` | ||
## Default methods <a name="custom"></a> | ||
@@ -104,3 +152,2 @@ | ||
## Levenshtein metric <a name="levenshtein"></a> | ||
@@ -115,2 +162,22 @@ Levenshtein distance is usually known as "the" edit distance. It is defined as the minimum number of edit operations (deletion, insertion and substitution) to transform the source string into the target string. The algorithm for distance computation is implemented using the dynamic programming approach with the full matrix construction, althought there are optimizations for time and space complexity those are not implemented here. | ||
## Jaccard <a name="jaccard"></a> | ||
The Jaccard index considers the strings as a bag-of-characters set and computes the cardinality of the intersection over the cardinality of the union. The distance function for Jaccard index is already normalized. | ||
## Metric comparador <a name="mc"></a> | ||
This is a class useful to compare the result of various metrics when applied on the same strings. For example, to see the difference between OSA and Damerau-Levenshtein you can pass those two metrics on a list to a MetricComparator instance and compute the similarity between the same two strings. | ||
By default the MetricComparator class use the 8 metrics implemented on the library, so you can compare all of them on the same two strings. Currently the similarity is the only measure implemented on the class. | ||
## Hamming <a name="hamming"></a> | ||
The Hamming distance count the positions where two strings differ. Normally the Hamming distance is only defined for strings of equal size but in this implementation strings of different size can be compared counting the difference in size as part of the distance. | ||
## Damerau-Levenshtein <a name="dl"></a> | ||
The Damerau-Levenshtein distance is like OSA but without the restriction on the number of transpositions for the same substring. | ||
## Dice (Sorenson-Dice) <a name="dice"></a> | ||
Is related to Jaccard index in the following manner: | ||
 | ||
## Contributors | ||
@@ -117,0 +184,0 @@ |
import Jaro from './jaro' | ||
import JaroCostOptions from './../interfaces/jaro-opts.interface' | ||
class JaroWinkler extends Jaro | ||
{ | ||
constructor(name: string = 'Jaro_Winkler') | ||
{ | ||
super(name); | ||
} | ||
/** | ||
class JaroWinkler extends Jaro { | ||
constructor (name: string = 'JaroWinkler') { | ||
super(name) | ||
} | ||
/** | ||
* Jaro Winkler Similarity | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param p | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param p | ||
*/ | ||
public similarity(source: string, target: string, {insertionCost, deletionCost, substitutionCost, lambdaCost, roCost}: JaroCostOptions = {}): number | ||
{ | ||
const p: number = roCost ?? 0.1; | ||
if (!( 0 <= p && p <= 0.25 )) | ||
{ | ||
new Error("The p parameter must be between 0 and 0.25"); | ||
} | ||
public similarity (source: string, target: string, { insertionCost, deletionCost, substitutionCost, lambdaCost, roCost }: JaroCostOptions = {}): number { | ||
const p: number = roCost ?? 0.1 | ||
if (!(p >= 0 && p <= 0.25)) { | ||
throw new Error('The p parameter must be between 0 and 0.25') | ||
} | ||
let l: number = 0; | ||
const maxL : number = lambdaCost ?? 4; | ||
let l: number = 0 | ||
const maxL: number = lambdaCost ?? 4 | ||
for( let i = 0; i < maxL; i++) | ||
{ | ||
if(source[i] != target[i]) break; | ||
l++; | ||
} | ||
for (let i = 0; i < maxL; i++) { | ||
if (source[i] !== target[i]) break | ||
l++ | ||
} | ||
const j: number = super.similarity(source, target, {insertionCost, deletionCost, substitutionCost}); | ||
return j + l*p*(1 - j); | ||
const j: number = super.similarity(source, target, { insertionCost, deletionCost, substitutionCost }) | ||
return j + l * p * (1 - j) | ||
} | ||
} | ||
/** | ||
/** | ||
* Jaro Winkler distance | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param p | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param p | ||
*/ | ||
public distance(source: string, target: string, {insertionCost, deletionCost, substitutionCost, lambdaCost, roCost}: JaroCostOptions = {}): number | ||
{ | ||
return 1 - this.similarity(source, target, {insertionCost, deletionCost, substitutionCost, lambdaCost, roCost}) | ||
} | ||
public distance (source: string, target: string, { insertionCost, deletionCost, substitutionCost, lambdaCost, roCost }: JaroCostOptions = {}): number { | ||
return 1 - this.similarity(source, target, { insertionCost, deletionCost, substitutionCost, lambdaCost, roCost }) | ||
} | ||
} | ||
export default JaroWinkler; | ||
export default JaroWinkler |
import Metric from './metric' | ||
import JaroCostOptions from './../interfaces/jaro-opts.interface' | ||
class Jaro extends Metric | ||
{ | ||
constructor (name: string = 'Jaro') | ||
{ | ||
class Jaro extends Metric { | ||
constructor (name: string = 'Jaro') { | ||
super(name) | ||
} | ||
/** | ||
* Jaro Similarity | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param source | ||
* @param target | ||
* @param cost | ||
*/ | ||
public similarity(source: string, target: string, { deletionCost, insertionCost, substitutionCost }: JaroCostOptions = {}) : number | ||
{ | ||
const sourceLength: number = source.length; | ||
const targetLength: number = target.length; | ||
public similarity (source: string, target: string, { deletionCost, insertionCost, substitutionCost }: JaroCostOptions = {}): number { | ||
const sourceLength: number = source.length | ||
const targetLength: number = target.length | ||
if (sourceLength == 0 && targetLength == 0) | ||
{ | ||
return 1; | ||
if (sourceLength === 0 && targetLength === 0) { | ||
return 1 | ||
} | ||
const matchDistance: number = Math.max(sourceLength, targetLength) / 2 - 1; | ||
let sourceMatches: Array<boolean> = new Array(sourceLength); | ||
let targetMatches: Array<boolean> = new Array(targetLength); | ||
const matchDistance: number = Math.max(sourceLength, targetLength) / 2 - 1 | ||
const sourceMatches: boolean[] = new Array(sourceLength) | ||
const targetMatches: boolean[] = new Array(targetLength) | ||
let matches: number = 0; | ||
let transpositions: number = 0; | ||
let start: number = 0; | ||
let end: number = 0; | ||
let matches: number = 0 | ||
let transpositions: number = 0 | ||
let start: number = 0 | ||
let end: number = 0 | ||
for (let i = 0; i < sourceLength; i++) | ||
{ | ||
start = Math.max(0, i - matchDistance); | ||
end = Math.min(i+matchDistance+1, targetLength); | ||
for (let i = 0; i < sourceLength; i++) { | ||
start = Math.max(0, i - matchDistance) | ||
end = Math.min(i + matchDistance + 1, targetLength) | ||
for (let j = start; j < end; j++ ) | ||
{ | ||
if (targetMatches[j]) continue; | ||
if (source[i] === target[j]) | ||
{ | ||
sourceMatches[i] = true; | ||
targetMatches[j] = true; | ||
matches++; | ||
break; | ||
} | ||
for (let j = start; j < end; j++) { | ||
if (targetMatches[j]) continue | ||
if (source[i] === target[j]) { | ||
sourceMatches[i] = true | ||
targetMatches[j] = true | ||
matches++ | ||
break | ||
} | ||
} | ||
} | ||
if (matches == 0) return 0; | ||
if (matches === 0) return 0 | ||
let k: number = 0; | ||
let k: number = 0 | ||
for (let i = 0; i < sourceLength; i++) | ||
{ | ||
if (!sourceMatches[i]) continue; | ||
while (!targetMatches[k]) k++; | ||
if (source[i] != target[k]) transpositions++; | ||
k++; | ||
for (let i = 0; i < sourceLength; i++) { | ||
if (!sourceMatches[i]) continue | ||
while (!targetMatches[k]) k++ | ||
if (source[i] !== target[k]) transpositions++ | ||
k++ | ||
} | ||
return ((matches / sourceLength) + (matches/targetLength) + ((matches - transpositions / 2) / matches)) / 3; | ||
return ((matches / sourceLength) + (matches / targetLength) + ((matches - transpositions / 2) / matches)) / 3 | ||
} | ||
/** | ||
* Jaro distance | ||
* @param source | ||
* @param target | ||
* @param cost | ||
* @param source | ||
* @param target | ||
* @param cost | ||
*/ | ||
public distance (source: string, target: string, { deletionCost, insertionCost, substitutionCost }:JaroCostOptions = {} ): number | ||
{ | ||
return 1 - this.similarity(source, target, {deletionCost, insertionCost, substitutionCost}); | ||
public distance (source: string, target: string, { deletionCost, insertionCost, substitutionCost }: JaroCostOptions = {}): number { | ||
return 1 - this.similarity(source, target, { deletionCost, insertionCost, substitutionCost }) | ||
} | ||
} | ||
export default Jaro |
@@ -10,3 +10,2 @@ import Metric from './metric' | ||
public distance (source: string, target: string, { deletionCost, insertionCost, substitutionCost }: LevenshteinCostOptions = {}): number { | ||
const sourceLength: number = source.length | ||
@@ -45,8 +44,8 @@ const targetLength: number = target.length | ||
public maxDistance (source: string, target: string, { deletionCost, insertionCost, substitutionCost }: LevenshteinCostOptions = {}): number { | ||
public maxDistance (source: string, target: string, { deletionCost, insertionCost, substitutionCost, cost = 1 }: LevenshteinCostOptions = {}): number { | ||
const sourceLength: number = source.length | ||
const targetLength: number = target.length | ||
const delCost: number = deletionCost ?? 1 | ||
const insCost: number = insertionCost ?? 1 | ||
const subCost: number = substitutionCost ?? 1 | ||
const delCost: number = deletionCost ?? cost | ||
const insCost: number = insertionCost ?? cost | ||
const subCost: number = substitutionCost ?? cost | ||
@@ -53,0 +52,0 @@ const maxDel: number = Math.max(sourceLength - targetLength, 0) |
@@ -52,4 +52,15 @@ import LevenshteinCostOptions from '../interfaces/levenshtein-opts.interface' | ||
} | ||
/** | ||
* similarity | ||
*/ | ||
public similarity (source: string, target: string, costs: LevenshteinCostOptions = {}): number { | ||
return 1 - this.normalizedDistance(source, target, costs) | ||
} | ||
get name (): string { | ||
return this._name | ||
} | ||
} | ||
export default Metric |
@@ -5,3 +5,7 @@ import Levenshtein from './hermetrics/levenshtein' | ||
import JaroWinkler from './hermetrics/jaro_winkler' | ||
import MetricComparator from './hermetrics/metric-comparator' | ||
import Jaccard from './hermetrics/jaccard' | ||
import Hamming from './hermetrics/hamming' | ||
import DamerauLevenshtein from './hermetrics/damerau_levenshtein' | ||
import Dice from './hermetrics/dice' | ||
export { | ||
@@ -11,3 +15,8 @@ Levenshtein, | ||
Jaro, | ||
JaroWinkler | ||
JaroWinkler, | ||
MetricComparator, | ||
Jaccard, | ||
Hamming, | ||
DamerauLevenshtein, | ||
Dice | ||
} |
@@ -5,2 +5,4 @@ export default interface LevenshteinCostOptions { | ||
substitutionCost?: number | ||
transpositionCost?: number | ||
cost?: number | ||
} |
77024
64
1677
180