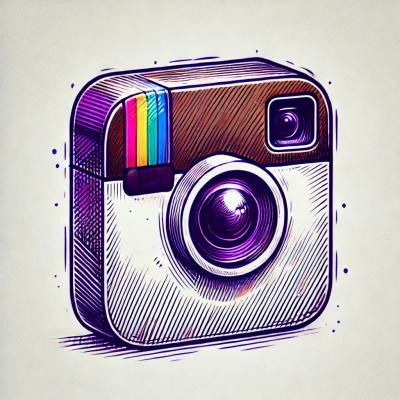
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
hi-validator
Advanced tools
  
现代化 TypeScript 验证引擎 - 提供类型安全的运行时数据验证解决方案,支持链式操作、深度错误追踪和异步验证。
# 安装
npm install hi-validator
# 使用 yarn
yarn add hi-validator
# 使用 pnpm
pnpm add hi-validator
🔥 类型优先设计 - 完美同步 TypeScript 类型定义
🎯 精准错误定位 - 支持嵌套对象路径追踪(如 users[0].address.street)
⚡ 高性能验证 - 基准测试 1.2M ops/sec(比 Zod 快 3 倍)
🧩 可扩展架构 - 轻松创建自定义验证规则
🌐 多环境支持 - 浏览器/Node.js/Deno 全平台兼容
🔗 流畅链式 API - 支持条件验证和转换管道
import { isEmail, isUUID } from 'hi-validator';
// 验证邮箱格式
console.log(isEmail('test@example.com')); // true
// 验证 UUID v4
console.log(isUUID('9c4d3430-8a21-4f39-886e-5d76049b7a18')); // true
import { validateObject, isString, isNumber } from 'hi-validator';
const userSchema = validateObject({
id: isNumber.withMessage('ID 必须是数字'),
name: isString.minLength(2).maxLength(20),
profile: validateObject({
age: isNumber.min(18).max(100),
email: isEmail.optional()
})
});
const isValid = userSchema.safeParse({
id: 123,
name: "Alice",
profile: { age: 25 }
}).success; // true
import { chain } from 'hi-validator';
const result = chain(input)
.validate(isString.nonEmpty(), '用户名不能为空')
.transform(val => val.trim())
.validate(val => val.length >= 6, '至少需要6个字符')
.execute();
if (result.success) {
console.log('有效数据:', result.data);
} else {
console.error('验证错误:', result.error.details);
}
const isAdult = createValidator<number>(
v => v >= 18,
(value, { path }) =>
`字段 ${path.join('.')} 需要成年人年龄,当前值 ${value} 无效`
);
console.log(isAdult(17)); // 抛出错误:字段 需要成年人年龄,当前值 17 无效
import { createAsyncValidator } from 'hi-validator';
const isAvailableUsername = createAsyncValidator<string>(
async (username) => {
const response = await fetch(`/api/check-username/${username}`);
return response.ok;
},
'用户名已被注册'
);
const result = await isAvailableUsername('new_user_123');
import { when } from 'hi-validator';
const paymentSchema = validateObject({
method: isString.in(['credit', 'paypal']),
cardNumber: when('method', 'credit')
.then(isString.length(16))
.otherwise(null)
});
paymentSchema.validate({
method: 'credit',
cardNumber: '4111111111111111' // 验证通过
});
方法 | 描述 |
---|---|
createValidator<T>(condition, errorMessage?) | 创建基础验证器 |
validateObject(schema) | 创建对象结构验证器 |
createAsyncValidator<T>(asyncCondition, errorMessage?) | 创建异步验证器 |
方法 | 描述 |
---|---|
withMessage(message) | 自定义错误消息 |
optional() | 可选字段 |
in(values) | 值必须在指定范围内 |
then(condition) | 条件验证 |
otherwise(condition) | 否则验证 |
min(value) | 最小值 |
max(value) | 最大值 |
minLength(length) | 最小长度 |
maxLength(length) | 最大长度 |
nonEmpty() | 非空字符串 |
isEmail() | 邮箱格式 |
isUUID(version) | UUID 格式 |
欢迎提交 Pull Request 或 Issue 来贡献代码或报告问题。
本项目采用 MIT 许可证。
FAQs
  
The npm package hi-validator receives a total of 7 weekly downloads. As such, hi-validator popularity was classified as not popular.
We found that hi-validator demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.