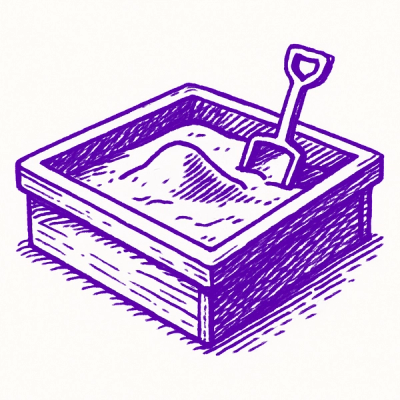
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
html-to-document-core
Advanced tools
Core engine that parses HTML into an intermediate DocumentElement tree and exposes a plugin registry so external adapters can convert that tree into DOCX, PDF, XLSX, Markdown and more.
Core engine for converting HTML to document formats.
This package provides the core parsing and conversion infrastructure. Adapters for specific output formats (e.g., DOCX, PDF) can be plugged in at runtime.
# Install the core engine
npm install html-to-document-core html-to-document-adapter-docx
# Or install the all-in-one wrapper (includes core + default adapters)
npm install html-to-document
For full documentation and usage examples, visit:
https://www.npmjs.com/package/html-to-document
import { init, Converter } from 'html-to-document-core';
import { DocxAdapter } from 'html-to-document-adapter-docx';
// Initialize with optional tags, middleware, and adapters
const converter = init({
adapters: {
register: [{ format: 'docx', adapter: DocxAdapter }],
},
tags: {
defaultStyles: [
{ key: 'p', styles: { marginBottom: '1px', marginTop: '1px' } },
],
},
});
// Parse HTML into an intermediate format
const elements = await converter.parse('<p>Hello, world!</p>');
// Convert parsed elements using a registered adapter (e.g., 'docx')
const outputBuffer = await converter.convert(elements, 'docx');
Or with the wrapper package:
import { init, DocxAdapter } from 'html-to-document';
// wrapper automatically includes core + DOCX adapter
const converter = init({
adapters: {
register: [{ format: 'docx', adapter: DocxAdapter }],
},
tags: {
defaultStyles: [
{ key: 'p', styles: { marginBottom: '1px', marginTop: '1px' } },
],
},
});
const buffer = await converter.convert('<p>Example</p>', 'docx');
You can install any adapter without the wrapper. For example, to add the DOCX adapter:
npm install html-to-document-adapter-docx
After installing, register it when initializing the core:
import { init } from 'html-to-document-core';
import { DocxAdapter } from 'html-to-document-adapter-docx';
const converter = init({
adapters: {
register: [{ format: 'docx', adapter: DocxAdapter }],
},
});
// Now you can convert:
const elements = await converter.parse('<p>Hello</p>');
const docxBuffer = await converter.convert(elements, 'docx');
init(options?: InitOptions): Converter
options
: configuration for tags, middleware, adapters, and DOM parser.Converter
instance.Converter
parse(html: string): Promise<DocumentElement[]>
convert(elements: DocumentElement[] | string, format: string): Promise<Buffer | Blob>
useMiddleware(mw: Middleware): void
registerConverter(format: string, adapter: IDocumentConverter): void
serialize(elements: DocumentElement[]): string
# At repo root
npm install
npm run build
# To test core only
cd packages/core
npm test
# Lint and format
npm run lint
npm run format
ISC
FAQs
Core engine that parses HTML into an intermediate DocumentElement tree and exposes a plugin registry so external adapters can convert that tree into DOCX, PDF, XLSX, Markdown and more.
The npm package html-to-document-core receives a total of 35 weekly downloads. As such, html-to-document-core popularity was classified as not popular.
We found that html-to-document-core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.