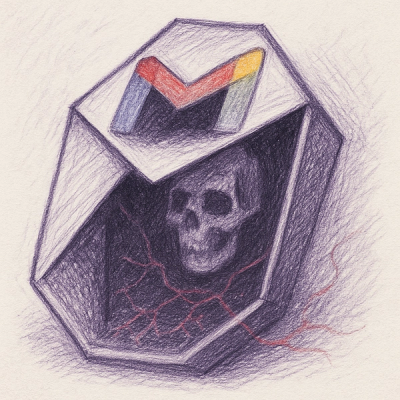
Research
Using Trusted Protocols Against You: Gmail as a C2 Mechanism
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.
htmldl
is a lightweight and customizable utility for exporting DOM nodes as images (PNG, JPEG, or SVG) in React applications. It is built on top of the html-to-image
library, providing an easy-to-use API to generate high-quality images from any HTML content.
Install the package using npm or bun:
npm install htmldl
or
bun add htmldl
Below is an example of how you can use htmldl
in your React project:
import { useCallback, useRef } from "react";
import { downloadNodeAsImage } from "htmldl";
function App() {
const printRef = useRef<HTMLDivElement>(null);
const handleDownload = useCallback(async () => {
if (!printRef.current) return;
await downloadNodeAsImage(printRef.current, {
fileName: "example-download",
format: "jpeg",
quality: 0.9,
scale: 2,
backgroundColor: "#ffffff",
onDownloadComplete: () => alert("Download complete!"),
onError: (error) => console.error("Download failed:", error),
});
}, []);
return (
<main>
<div>
<div ref={printRef}>
<h1>htmldl Example</h1>
<p>
Export this content as an image by clicking the download button
below.
</p>
</div>
</div>
<button onClick={handleDownload}>Download as Image</button>
</main>
);
}
export default App;
downloadNodeAsImage(node: HTMLElement, options?: ExportOptions): Promise<void>
Converts a DOM node to an image and triggers a download.
node
(required): The DOM element you want to capture.options
(optional): An object to customize the export process.Option | Type | Default | Description |
---|---|---|---|
fileName | string | "download" | Name of the downloaded file. |
format | "png" | "jpeg" | "svg" | "png" | Format of the downloaded image. |
quality | number | 1 | JPEG quality (0 to 1). |
scale | number | 1 | Scale factor for resolution. |
backgroundColor | string | undefined | Background color for the image (e.g., "#ffffff" ). |
onDownloadComplete | () => void | undefined | Callback executed after the download completes. |
onError | (error: Error) => void | undefined | Custom error handler, invoked when an error occurs. |
await downloadNodeAsImage(printRef.current, {
fileName: "custom-image",
format: "svg",
scale: 3,
backgroundColor: "#ff0000",
onDownloadComplete: () => console.log("Export complete!"),
onError: (err) => console.error("Export failed:", err),
});
htmldl
uses the following utility methods from html-to-image
to generate images:
toPng
: Converts a node to a PNG image.toJpeg
: Converts a node to a JPEG image.toSvg
: Converts a node to an SVG image.The triggerDownload
function automatically creates a download link for the generated image.
16.8+
(requires hooks support).We welcome contributions! If you encounter any issues or have ideas for improvements, feel free to open an issue or submit a pull request.
Start using htmldl
today and make exporting your DOM content as images a breeze! 🎉
FAQs
A utility to capture HTML DOM nodes as PNG images and download them.
The npm package htmldl receives a total of 7 weekly downloads. As such, htmldl popularity was classified as not popular.
We found that htmldl demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.
Product
We redesigned Socket's first logged-in page to display rich and insightful visualizations about your repositories protected against supply chain threats.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.