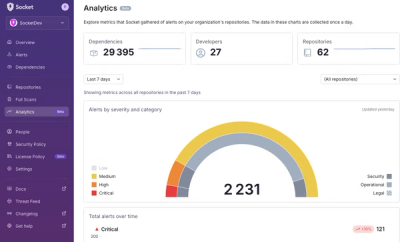
Product
Introducing Historical Analytics – Now in Beta
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.
http-request-factory
Advanced tools
 
Check the API Docs here
A wrapper for the Fetch API to simplify handling of HTTP requests.
Works in:
esm.sh
)--experimental-fetch
flag set before v18).It provides a method-chain interface to setup request and configuration-driven API handling.
The library uses
yarn add http-request-factory
# or
npm i http-request-factory
// node / bundlers
import { HTTPRequestFactory } from 'http-request-factory';
// deno
import {HTTPRequestFactory} from 'https://esm.sh/http-request-factory';
//...
const factory = new HTTPRequestFactory()
.withLogLevel(myenv.LOG_LEVEL);
const data = await factory
.createGETRequest('https://mydomain.com/some-endpoint')
.withQueryParam('color', 'blue')
.withAccept('application/json')
.withHeader('x-my-app-key', myenv.APP_KEY)
.execute();
//data will be the actual response body
It's possible to define a group of endpoints that live at the same base URL by creating an API configuration:
//api-config.ts
import {APIConfig} from 'http-request-factory';
const apis : APIConfig[] = [{
name : 'aws',
baseURL : 'https://aws.mydomain.com/a09dsjas0j9df0asj0fads9jdsj9',
endpoints : {
'get-products' : {
target : '/get-products',
method : 'GET' //optional defaults to GET
}
}
}, {
name : 'my-api'
baseURL : 'https://mydomain.com/api/v2',
meta : {
poweredBy : 'me'
},
endpoints : {
'get-product-info' : {
target : '/product/{{productId}}',
}
}
}];
export default apis;
The APIs can then be attached to the request factory using factory.withAPIConfig()
and requests can be created using factory.createAPIRequest(apiName, endpointName)
It's possible to conditionally configure requests based using factory.when((request:HTTPRequest) => boolean).<any-configuration-method>()
API information is appended to its endpoints' meta dictionary.
When a passed value in configuration methods is a function, its value will be resolved just before executing the fetch request.
//request-factory.ts
import { HTTPRequestFactory } from 'http-request-factory';
import apis from './api-config.ts'
//...
export default new HTTPRequestFactory()
.withLogLevel(myenv.LOG_LEVEL)
.withAPIConfig(...apis)
.when((request) => { //set a condition for the next settings
request.meta.api?.name === 'aws'
})
.withHeaders({
'x-aws-api-key' : myenv.AWS_API_KEY,
'authorization' : () => `Bearer ${sessionModel.awsAccessToken}`
})
.withLogLevel('trace')
.always() //resets the condition
.withHeader('x-powered-by', (request) => request.meta.poweredBy);
Endpoint target paths can contain params in the form of {{paramName}}
that can be substituted using request.withURLParam(paramName, value)
. Useful, for instance, to wrap REST APIs.
//some-controller.ts
import requestFactory from './request-factory.ts';
const awsData = await requestFactory
.createAPIRequest('aws', 'get-products')
.execute();
const myAPIData = await requestFactory
.createAPIRequest('my-api', 'get-product-info')
.withURLParam('productID', 123)
.execute();
The test suite is written in Vitest and requires Deno
FAQs
 
The npm package http-request-factory receives a total of 20 weekly downloads. As such, http-request-factory popularity was classified as not popular.
We found that http-request-factory demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.
Product
Module Reachability filters out unreachable CVEs so you can focus on vulnerabilities that actually matter to your application.
Company News
Socket is bringing best-in-class reachability analysis into the platform — cutting false positives, accelerating triage, and cementing our place as the leader in software supply chain security.