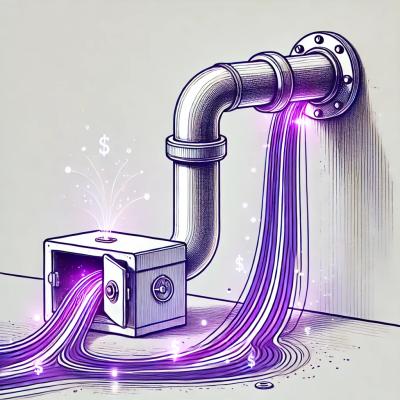
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
http-shutdown
Advanced tools
The http-shutdown npm package extends the standard Node.js HTTP server with a shutdown method. This allows you to gracefully shut down the server, ensuring that all existing connections are served before the server is closed.
Graceful Shutdown
This feature allows you to add a shutdown method to your HTTP server instance. When called, it will not accept new connections and will wait for all existing connections to end before shutting down the server.
const http = require('http');
const enableShutdown = require('http-shutdown');
const server = enableShutdown(http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello World!');
}));
server.listen(3000);
// Shutdown the server after 10 seconds
setTimeout(() => {
server.shutdown(() => {
console.log('Server is gracefully shut down.');
});
}, 10000);
Stoppable is an npm package that also provides a way to gracefully stop an HTTP server. It tracks active connections and allows you to set a timeout for how long the server should wait for active connections to finish before forcibly shutting down. It is similar to http-shutdown but offers a timeout feature.
Terminus is an npm package designed to add graceful shutdown and Kubernetes readiness / liveness checks for any HTTP applications. It extends the server with more advanced features compared to http-shutdown, such as signal handling for shutdown, health checks, and on-shutdown hooks.
Shutdown a Nodejs HTTP server gracefully by doing the following:
Other solutions might just use server.close
which only terminates the listening socket and waits for other sockets to close - which is incomplete since keep-alive sockets can still make requests. Or, they may use ref()/unref()
to simply cause Nodejs to terminate if the sockets are idle - which doesn't help if you have other things to shutdown after the server shutsdown.
http-shutdown
is a complete solution. It uses idle indicators combined with an active socket list to safely, and gracefully, close all sockets. It does not use ref()/unref()
but, instead, actively closes connections as they finish meaning that socket 'close' events still work correctly since the sockets are actually closing - you're not just unref
ing and forgetting about them.
$ npm install http-shutdown
There are currently two ways to use this library. The first is explicit wrapping of the Server
object:
// Create the http server
var server = require('http').createServer(function(req, res) {
res.end('Good job!');
});
// Wrap the server object with additional functionality.
// This should be done immediately after server construction, or before you start listening.
// Additional functionailiy needs to be added for http server events to properly shutdown.
server = require('http-shutdown')(server);
// Listen on a port and start taking requests.
server.listen(3000);
// Sometime later... shutdown the server.
server.shutdown(function(err) {
if (err) {
return console.log('shutdown failed', err.message);
}
console.log('Everything is cleanly shutdown.');
});
The second is implicitly adding prototype functionality to the Server
object:
// .extend adds a .withShutdown prototype method to the Server object
require('http-shutdown').extend();
var server = require('http').createServer(function(req, res) {
res.end('God job!');
}).withShutdown(); // <-- Easy to chain. Returns the Server object
// Sometime later, shutdown the server.
server.shutdown(function(err) {
if (err) {
return console.log('shutdown failed', err.message);
}
console.log('Everything is cleanly shutdown.');
});
$ npm test
FAQs
Gracefully shutdown a running HTTP server.
The npm package http-shutdown receives a total of 909,824 weekly downloads. As such, http-shutdown popularity was classified as popular.
We found that http-shutdown demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.