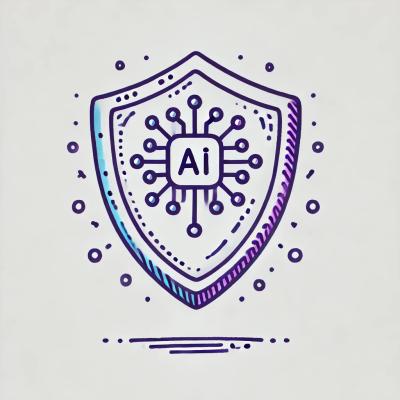
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
i18next-xhr-backend
Advanced tools
The i18next-xhr-backend package is a backend layer for the i18next internationalization framework, which allows you to load translations from a server using XMLHttpRequest (XHR). This is particularly useful for dynamic loading of translations in web applications.
Loading translations from a server
This feature allows you to load translation files from a server. The `loadPath` option specifies the path to the translation files, which can include placeholders for the language (`{{lng}}`) and namespace (`{{ns}}`).
const i18next = require('i18next');
const XHR = require('i18next-xhr-backend');
i18next
.use(XHR)
.init({
backend: {
loadPath: '/locales/{{lng}}/{{ns}}.json'
}
}, function(err, t) {
if (err) return console.error(err);
console.log('i18next is ready...');
});
Customizing XHR settings
This feature allows you to customize the XHR settings, such as using the Fetch API instead of the default XMLHttpRequest. The `ajax` function can be overridden to provide custom behavior for loading translation files.
const i18next = require('i18next');
const XHR = require('i18next-xhr-backend');
i18next
.use(XHR)
.init({
backend: {
loadPath: '/locales/{{lng}}/{{ns}}.json',
ajax: function (url, options, callback, data) {
// Custom AJAX request
fetch(url, options)
.then(response => response.json())
.then(data => callback(data, { status: '200' }))
.catch(error => callback(null, { status: '500' }));
}
}
});
Caching translations
This feature allows you to cache translations in localStorage to reduce the number of requests to the server. The `cache` option can be configured to enable caching and set a key prefix for the cached translations.
const i18next = require('i18next');
const XHR = require('i18next-xhr-backend');
const Cache = require('i18next-localstorage-cache');
i18next
.use(XHR)
.use(Cache)
.init({
backend: {
loadPath: '/locales/{{lng}}/{{ns}}.json'
},
cache: {
enabled: true,
prefix: 'i18next_res_' // Key prefix for localStorage
}
});
The i18next-http-backend package is another backend layer for i18next that uses the Fetch API to load translations from a server. It is similar to i18next-xhr-backend but uses modern Fetch API instead of XMLHttpRequest, providing better support for modern browsers and environments.
The i18next-localstorage-backend package allows you to load translations from localStorage. This is useful for offline applications or scenarios where you want to avoid making network requests for translations. It differs from i18next-xhr-backend by focusing on localStorage as the source of translations.
The i18next-fs-backend package is designed for Node.js environments and allows you to load translations from the file system. This is useful for server-side rendering or Node.js applications. It differs from i18next-xhr-backend by focusing on file system access rather than network requests.
This is a simple i18next backend to be used in the browser. It will load resources from a backend server using the xhr API.
Source can be loaded via npm, bower or downloaded from this repo.
# npm package
$ npm install i18next-xhr-backend
# bower
$ bower install i18next-xhr-backend
Wiring up:
import i18next from 'i18next';
import XHR from 'i18next-xhr-backend';
i18next.use(XHR).init(i18nextOptions);
window.i18nextXHRBackend
{
// path where resources get loaded from, or a function
// returning a path:
// function(lngs, namespaces) { return customPath; }
// the returned path will interpolate lng, ns if provided like giving a static path
loadPath: '/locales/{{lng}}/{{ns}}.json',
// path to post missing resources
addPath: 'locales/add/{{lng}}/{{ns}}',
// your backend server supports multiloading
// /locales/resources.json?lng=de+en&ns=ns1+ns2
// Adapter is needed to enable MultiLoading https://github.com/i18next/i18next-multiload-backend-adapter
// Returned JSON structure in this case is
// {
// lang : {
// namespaceA: {},
// namespaceB: {},
// ...etc
// }
// }
allowMultiLoading: false, // set loadPath: '/locales/resources.json?lng={{lng}}&ns={{ns}}' to adapt to multiLoading
// parse data after it has been fetched
// in example use https://www.npmjs.com/package/json5
// here it removes the letter a from the json (bad idea)
parse: function(data) { return data.replace(/a/g, ''); },
//parse data before it has been sent by addPath
parsePayload: function(namespace, key, fallbackValue) { return { key } },
// allow cross domain requests
crossDomain: false,
// allow credentials on cross domain requests
withCredentials: false,
// overrideMimeType sets request.overrideMimeType("application/json")
overrideMimeType: false,
// custom request headers sets request.setRequestHeader(key, value)
customHeaders: {
authorization: 'foo',
// ...
},
// define a custom xhr function
// can be used to support XDomainRequest in IE 8 and 9
//
// 'url' will be passed the value of 'loadPath'
// 'options' will be this entire options object
// 'callback' is a function that takes two parameters, 'data' and 'xhr'.
// 'data' should be the key:value translation pairs for the
// requested language and namespace, or null in case of an error.
// 'xhr' should be a status object, e.g. { status: 200 }
// 'data' will be a key:value object used when saving missing translations
ajax: function (url, options, callback, data) {},
// adds parameters to resource URL. 'example.com' -> 'example.com?v=1.3.5'
queryStringParams: { v: '1.3.5' }
}
Options can be passed in:
preferred - by setting options.backend in i18next.init:
import i18next from 'i18next';
import XHR from 'i18next-xhr-backend';
i18next.use(XHR).init({
backend: options,
});
on construction:
import XHR from 'i18next-xhr-backend';
const xhr = new XHR(null, options);
via calling init:
import XHR from 'i18next-xhr-backend';
const xhr = new XHR();
xhr.init(null, options);
Install from @types
(for TypeScript v2 and later):
npm install --save-dev @types/i18next-xhr-backend
Install from typings
:
typings install --save --global dt~i18next-xhr-backend
3.2.2
FAQs
backend layer for i18next using browsers xhr
The npm package i18next-xhr-backend receives a total of 72,347 weekly downloads. As such, i18next-xhr-backend popularity was classified as popular.
We found that i18next-xhr-backend demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.