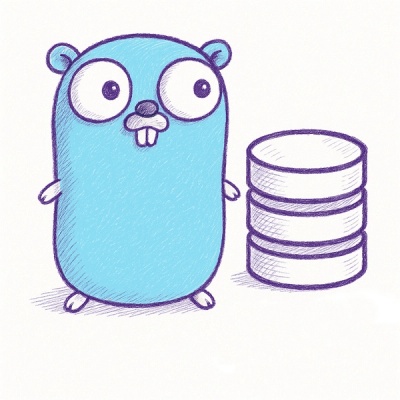
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
ignore-loader
Advanced tools
The ignore-loader npm package is a webpack loader that allows you to ignore specific files or modules during the build process. This can be useful for excluding files that are not needed in the final bundle, such as test files, large assets, or platform-specific code.
Ignore specific files
This feature allows you to ignore files that match a specific pattern. In this example, all files ending with .test.js will be ignored during the build process.
module.exports = {
module: {
rules: [
{
test: /\.test.js$/,
loader: 'ignore-loader'
}
]
}
};
Ignore specific modules
This feature allows you to ignore specific modules. In this example, any module that matches the pattern 'some-large-module' will be ignored during the build process.
module.exports = {
module: {
rules: [
{
test: /some-large-module/,
loader: 'ignore-loader'
}
]
}
};
The null-loader package is similar to ignore-loader in that it allows you to ignore specific files or modules. However, instead of ignoring them, it replaces the content with an empty module. This can be useful if you want to ensure that the ignored files do not affect the build process in any way.
The file-loader package is used to emit files as separate assets in the build process. While it is not used to ignore files, it can be used in conjunction with ignore-loader to manage which files are included or excluded from the final bundle.
The url-loader package is similar to file-loader but can inline files as base64 URIs if they are below a certain size. This can be useful for managing small assets. Like file-loader, it can be used alongside ignore-loader to control the inclusion of files in the build.
To ignore certain files when building webpack application.
$ npm install --save-dev ignore-loader
.css
)webpack.config.js
module.exports = {
// other configurations
module: {
loaders: [
{ test: /\.css$/, loader: 'ignore-loader' }
]
}
};
FAQs
Webpack loader to ignore certain package on build.
The npm package ignore-loader receives a total of 207,447 weekly downloads. As such, ignore-loader popularity was classified as popular.
We found that ignore-loader demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.