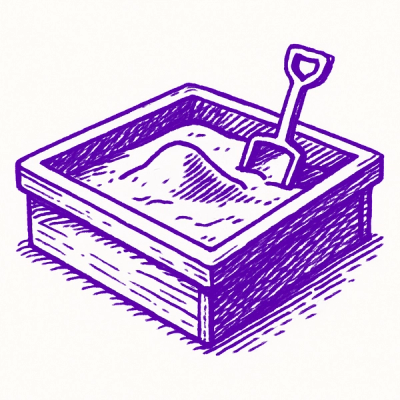
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
iltorb is a Node.js package offering native bindings for the brotli compression library.
This module uses prebuild
to download a pre-compiled binary for your platform, if it exists. Otherwise, it will use node-gyp
to build the module.
npm install iltorb
The following is required to build from source or when a pre-compiled binary does not exist.
Omitting the callback argument will result in the compress and decompress methods to return a Promise.
const compress = require('iltorb').compress;
// callback style
compress(input, function(err, output) {
// ...
});
// promise style
compress(input)
.then(output => /* ... */)
.catch(err => /* ... */);
// async/await style
try {
const output = await compress(input);
} catch(err) {
// ...
}
const decompress = require('iltorb').decompress;
// callback style
decompress(input, function(err, output) {
// ...
});
// promise style
decompress(input)
.then(output => /* ... */)
.catch(err => /* ... */);
// async/await style
try {
const output = await decompress(input);
} catch(err) {
// ...
}
const compressSync = require('iltorb').compressSync;
try {
var output = compressSync(input);
} catch(err) {
// ...
}
const decompressSync = require('iltorb').decompressSync;
try {
var output = decompressSync(input);
} catch(err) {
// ...
}
const compressStream = require('iltorb').compressStream;
const fs = require('fs');
fs.createReadStream('path/to/input')
.pipe(compressStream())
.pipe(fs.createWriteStream('path/to/output'));
Call this method to flush pending data. Don't call this frivolously, premature flushes negatively impact the effectiveness of the compression algorithm.
const decompressStream = require('iltorb').decompressStream;
const fs = require('fs');
fs.createReadStream('path/to/input')
.pipe(decompressStream())
.pipe(fs.createWriteStream('path/to/output'));
The compress
, compressSync
and compressStream
methods may accept an optional brotliEncodeParams
object to define some or all of brotli's compression parameters:
const brotliEncodeParams = {
mode: 0,
quality: 11,
lgwin: 22,
lgblock: 0,
disable_literal_context_modeling: false,
size_hint: 0, // automatically set for `compress` and `compressSync`
large_window: false,
npostfix: 0,
ndirect: 0
};
I am unable to install iltorb
because the host (GitHub) that serves the binaries is blocked by my firewall.
a) By default, if the binaries could not be downloaded for any reason, the install script will attempt to compile the binaries locally on your machine. This requires having all of the build requirements fulfilled.
b) You can override the binary.host
value found in package.json
with the following methods:
npm_config_iltorb_binary_host=https://domain.tld/path
--iltorb_binary_host=https://domain.tld/path
Note: Both of these would result in downloading the binary from https://domain.tld/path/vX.X.X/iltorb-vX.X.X-node-vXX-arch.tar.gz
[2.4.5] - 2020-02-01
iltorb
is now deprecated in favor of zlib
.
zlib
module has support for brotli compression/decompression APIs starting with Node.js v10.16.0.FAQs
Brotli compression/decompression with native bindings
The npm package iltorb receives a total of 90,348 weekly downloads. As such, iltorb popularity was classified as popular.
We found that iltorb demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.