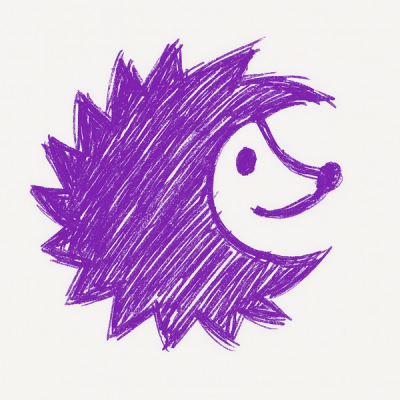
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
immutable-modify
Advanced tools
Slim zero dependencies helper function for modifying redux state or frozen objects
Easily create updated version of your Plain Javascript Object.
Function will create new references to objects or arrays when nested data is changed and leave references otherwise.
That allows react
and react-redux
to fast check your props using reference equality.
yarn add immutable-modify
or
npm install --save immutable-modify
Arguments:
Returns:
(Object): Returns object
import {set} from 'immutable-modify'
const state = {user: {name: 'Skywalker'}}
// same as set(state, ['user', 'name'], 'Dart Vader')
const newState = set(state, 'user.name', 'Dart Vader')
// newState is now {user: {name: 'Dart Vader'}}
newState === state // false
newState.user === state.user // false
Also value
could be function:
import {set} from 'immutable-modify'
const state = {user: {name: 'Skywalker', isJedi: false}, settings: {}}
const newState = set(state, 'user.isJedi', (isJedi) => !isJedi)
// newState is now {user: {name: 'Skywalker', isJedi: true}}
newState === state // false
newState.user === state.user // false
newState.settings === state.settings // true
That updater function should return new reference, otherwise nothing will updated:
import {set} from 'immutable-modify'
const state = {user: {name: 'Skywalker', isJedi: false}, settings: {}}
const newState = set(state, 'user', user => {
user.isJedi = true
return user
})
// newState is still {user: {name: 'Skywalker', isJedi: false}}
newState === state // true
newState.user === state.user // true
newState.settings === state.settings // true
import {push} from 'immutable-modify'
const state = {sequence: [{a: 1}, {a: 2}]}
const newState = push(state, 'sequence', {a: 3})
// newState is now {sequence: [{a: 1}, {a: 2}, {a: 3}]}
newState === state // false
newState.sequence === state.sequence // false
newState.sequence[0] === state.sequence[0] // true
newState.sequence[1] === state.sequence[1] // true
import {merge} from 'immutable-modify'
const state = {product: {name: 'sepulka'}}
const newState = merge(state, 'product', {description: 'Best with sepulator'})
// newState is now {product: {name: 'sepulka', description: 'Best with sepulator'}}
newState === state // false
newState.product === state.product // false
newState.product.name === state.product.name // true
import {remove} from 'immutable-modify'
const state = {product: {name: 'sepulka', isAvailable: true}}
const newState = remove(state, 'product.isAvailable')
// newState is now {product: {name: 'sepulka'}}
newState === state // false
newState.product === state.product // false
yarn test
FAQs
Modify structures in react/redux compatible way
The npm package immutable-modify receives a total of 456 weekly downloads. As such, immutable-modify popularity was classified as not popular.
We found that immutable-modify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.