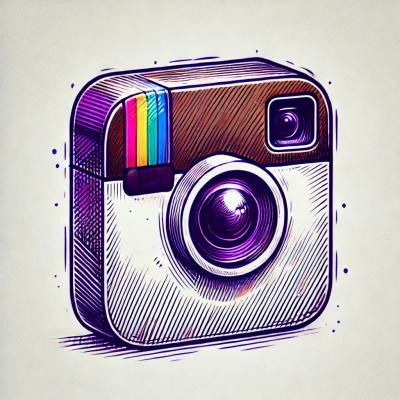
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
inherit-js
Advanced tools
Supply Chain Security
Vulnerability
Quality
Maintenance
License
Unpopular package
QualityThis package is not very popular.
Found 1 instance in 1 package
modified from https://raw.github.com/dfilatov/jquery-plugins/master/src/jquery.inherit/jquery.inherit.js
if you want to support IE6-8,use the difference between this repo and the repo above.
Plugin provides "class" and inheritance implementation. It brings some syntax sugar for "class" declarations, constructors, "super" calls and static members.
node: npm install inherit-js
bower: bower install inherit-js
var inherit = require('inherit');//seajs way.if you use requireJS,modify it.
var MyClass = $.inherit({
[name: 'ClassName'],
[base: ParentClass],
proto: prototypeMembers,
[statics: staticMembers]
});
// base "class"
var A = inherit({
// set the name,will generate a better constructor with name.
// this will be friendly for debug when you see the
// instance's constructor is a named Class instead of an anonymous Function
name: 'A',
/** @lends A.prototype */
proto: {
__constructor : function(property) { // constructor
this.property = property;
},
getProperty : function() {
return this.property + ' of instanceA';
},
getType : function() {
return 'A';
},
getStaticProperty : function() {
return this.__self.staticMember; // access to static
}
},
/** @lends A */
statics:{
staticProperty : 'staticA',
staticMethod : function() {
return this.staticProperty;
}
}
});
// inherited "class" from A
var B = $.inherit({
name:'B',
base: A,
/** @lends B.prototype */
proto: {
getProperty : function() { // overriding
return this.property + ' of instanceB';
},
getType : function() { // overriding + "super" call
return this.__base() + 'B';
}
},
/** @lends B */
statics: {
staticMethod : function() { // static overriding + "super" call
return this.__base() + ' of staticB';
}
}
});
var instanceOfA = new A('property');
var instanceOfB = new B('property');
instanceOfB instanceof B;//true
instanceOfB instanceof A;//true
instanceOfB.getProperty(); // returns 'property of instanceB'
instanceOfB.getType(); // returns 'AB'
B.staticMethod(); // returns 'staticA of staticB'
FAQs
inheritance for javascript
The npm package inherit-js receives a total of 0 weekly downloads. As such, inherit-js popularity was classified as not popular.
We found that inherit-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.