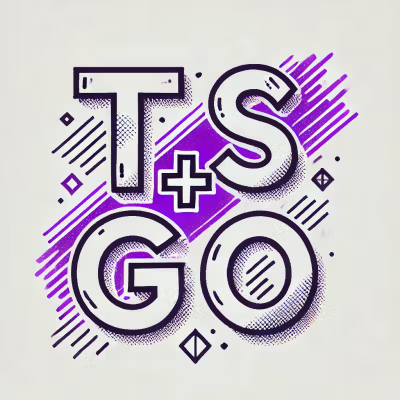
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
innovators
Advanced tools
An unofficial WhatsApp API using [baileys](https://github.com/whiskeysockets/baileys) implemented into [whatsapp-web.js](https://github.com/pedroslopez/whatsapp-web.js).
An unofficial WhatsApp API using baileys implemented into whatsapp-web.js.
You can use all the features here like you use whatsapp-web.js with the help of the main library, baileys.
I cannot guarantee that you can always use this library safely because the threat from WhatsApp to users who use unofficial WhatsApp API services is the possibility of an account ban/blocking for those who engage in such actions, and this ban can be for a significant period. So, use this at your own risk.
To get started, first, you need to install this library using npm/yarn/pnpm.
NPM
npm i innovators
Yarn
yarn add innovators
PNPM
pnpm install innovators
If you have installed the library, you can create a JavaScript file and fill it with the following code.
const { Client } = require("innovators");
const client = new Client({ sessionName: "mysession" });
client.initialize();
client.on("qr", (qr) => {
console.log("QR RECEIVED " + qr)
});
client.on("ready", (msg) => console.log(msg));
client.on("message", (msg) => {
if (msg.body == "#ping") {
msg.reply("pong!");
}
});
A brief explanation: You need to import the client class from the library and initialize it with the available variable (const client = new Client()
). After that, use events to obtain some data from the WhatsApp server connection.
There are several options available in this library.
/** Client options */
interface ClientOptions {
/** Session name of client */
sessionName: string;
/** If true, the bot can reply their message */
self: boolean;
/** Interval that set of store when the message is stored. Default 10s */
intervalStore: number;
}
/** Message send options */
interface MessageOptions {
/** If true, all the text/caption of message will be parsed to get the mentions text */
parseMention?: boolean;
/** Send message with mentions with specific jid */
mentions?: string[];
/** If you want to send message with replied/quoted, fill this option with message id */
quotedMsgId?: string;
/** If the content is MessageMedia, it would be recommended */
mimetype?: Mimetype;
/** If the content is MessageMedia, it would be recommended */
caption?: string;
/** Sticker metadata (if it set, the media will be sent as sticker) */
sticker?: StickerData;
/** Extra options */
extra?: any;
}
For more details, you can refer to index.d.ts.
"use-strict";
const { Client, MessageMedia, Location } = require("innovators");
const client = new Client({ sessionName: "baileys_session", self: true });
client.initialize();
console.log(client.state)
client.on("qr", (qr) => console.log(qr));
client.on("connecting", (msg) => console.log(msg));
client.on("disconnect", (msg) => {
console.log(msg)
client.destroy(true);
process.exit(1);
});
client.on("ready", (msg) => console.log(msg));
client.on("message", async (msg) => {
// if (!msg || !msg.id.fromMe) return;
const groupInfo = msg.isGroupMsg ? await client.getGroupMetadata(msg.from) : null;
console.log(msg);
if (msg.body == "#ping") {
return msg.reply(`pong! @${msg.sender.split("@")[0]}`, { parseMention: true });
} else if (msg.body == "#image") {
const media = await MessageMedia.fromUrl("https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcTH8GWuMpRA101NQjhhM0hY6XNOnoIfUeAPfA&usqp=CAU");
return msg.reply(media, { caption: "this is image!", sticker: { name: "asu", author: "kirek" } });
} else if (msg.body == "#video") {
const media = await MessageMedia.fromFilePath("./video.mp4");
return msg.reply(media, { sticker: { name: "initokyolagii", author: "demon" } });
} else if (msg.body == "#download") {
const media = await msg.downloadMediaMessage();
return msg.reply(media, { caption: "this is" });
} else if (msg.body.startsWith("#sticker")) {
let sticker = {};
const stickerData = msg.body.split(" ").slice(1).join(" ")?.split("|") || [];
if (stickerData.length > 0) {
sticker.name = stickerData[0];
sticker.author = stickerData[1];
}
const media = msg.hasQuotedMsg ? await (await msg.getMessageQuoted()).downloadMediaMessage() : await msg.downloadMediaMessage();
return msg.reply(media, { sticker });
} else if (msg.body == "#getQuoted") {
const message = await msg.getMessageQuoted();
return msg.reply(message.body);
} else if (msg.body == "#groupInfo") {
let text = `*Subject:* ${groupInfo.subject}`;
text += `\n*Owner:* @${groupInfo.owner?.split("@")[0] || "Unknown"}`;
text += `\n*Description:*\n${groupInfo.description}`;
return msg.reply(text, { parseMention: true });
} else if (
msg.body.startsWith("#add") ||
msg.body.startsWith("#kick") ||
msg.body.startsWith("#promote") ||
msg.body.startsWith("#demote")
) {
const isAdmin = groupInfo?.participants?.some((x) => x.jid == msg.sender && x.isAdmin);
const participants = msg.body.split(" ").slice(1).map((x) => `${x}@s.whatsapp.net`);
const command = msg.body.split(" ").shift().slice(1).toLowerCase();
if (isAdmin) {
return groupInfo.changeGroupParticipants(
msg.from,
participants,
command == "kick" ? "remove" : command
)
}
} else if (msg.body.startsWith("#subject") || msg.body.startsWith("#desc")) {
const isAdmin = groupInfo?.participants?.some((x) => x.jid == msg.sender && x.isAdmin);
const content = msg.body.split(" ").slice(1).join(" ");
const command = msg.body.split(" ").shift().slice(1).toLowerCase();
if (isAdmin) {
return groupInfo.updateGroup(
msg.from,
content,
command == "desc" ? "description" : command
)
}
} else if (msg.body.startsWith("#location")) {
const q = msg.body.split(" ").slice(1).join(" ");
if (q) {
const [latitude, longitude, description] = q.split("|");
const location = new Location(latitude, longitude, description);
return msg.reply(location);
}
} else if (msg.body == "#close") {
await client.close();
return process.exit(1);
}
})
/* client.on("group_participants_update", async (update) => {
if (!update) return;
const groupInfo = await client.getGroupMetadata(update.groupId);
if (update.type == "add") {
return client.sendMessage(update.groupId, `Welcome to *${groupInfo.subject}*`);
} else if (update.type == "remove") {
return client.sendMessage(update.groupId, `Goodbye from *${groupInfo.subject}*`);
} else if (update.type == "demote") {
return client.sendMessage(update.groupId, `We loss one admin in *${groupInfo.subject}*`);
} else if (update.type == "promote") {
return client.sendMessage(update.groupId, `New admin in *${groupInfo.subject}*`);
}
}) */
Find Us on Facebook By Serching INNOVATORS SOFT
FAQs
An unofficial WhatsApp API using [baileys](https://github.com/whiskeysockets/baileys) implemented into [whatsapp-web.js](https://github.com/pedroslopez/whatsapp-web.js).
We found that innovators demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.