interface-datastore
Advanced tools
Comparing version
@@ -0,1 +1,98 @@ | ||
/** | ||
* @packageDocumentation | ||
* | ||
* A Datastore is a key/value database that lets store/retrieve binary blobs using namespaced Keys. | ||
* | ||
* It is used by IPFS to store/retrieve arbitrary metadata needed to run the node - DHT provider records, signed peer records, etc. | ||
* | ||
* ## Backed Implementations | ||
* | ||
* - File System: [`datastore-fs`](https://github.com/ipfs/js-stores/tree/main/packages/datastore-fs) | ||
* - IndexedDB: [`datastore-idb`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-idb) | ||
* - level: [`datastore-level`](https://github.com/ipfs/js-stores/tree/main/packages/datastore-level) (supports any levelup compatible backend) | ||
* - Memory: [`datastore-core/memory`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/memory.ts) | ||
* - S3: [`datastore-s3`](https://github.com/ipfs/js-stores/tree/main/packages/datastore-s3) | ||
* | ||
* ## Wrapper Implementations | ||
* | ||
* - Keytransform: [`datstore-core/src/keytransform`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/keytransform.ts) | ||
* - Mount: [`datastore-core/src/mount`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/mount.ts) | ||
* - Namespace: [`datastore-core/src/namespace`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/namespace.ts) | ||
* - Sharding: [`datastore-core/src/sharding`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/sharding.ts) | ||
* - Tiered: [`datstore-core/src/tiered`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/tiered.ts) | ||
* | ||
* If you want the same functionality as [go-ds-flatfs](https://github.com/ipfs/go-ds-flatfs), use sharding with fs. | ||
* | ||
* @example | ||
* | ||
* ```js | ||
* import FsStore from 'datastore-fs' | ||
* import { ShardingDataStore, shard } from 'datastore-core' | ||
* | ||
* const fs = new FsStore('path/to/store') | ||
* | ||
* // flatfs now works like go-flatfs | ||
* const flatfs = await ShardingStore.createOrOpen(fs, new shard.NextToLast(2)) | ||
* ``` | ||
* | ||
* ### Test suite | ||
* | ||
* Available via the [`interface-datastore-tests`](https://npmjs.com/package/interface-datastore-tests) module | ||
* | ||
* ```js | ||
* import { interfaceDatastoreTests } from 'interface-datastore-tests' | ||
* | ||
* describe('mystore', () => { | ||
* interfaceDatastoreTests({ | ||
* async setup () { | ||
* return instanceOfMyStore | ||
* }, | ||
* async teardown () { | ||
* // cleanup resources | ||
* } | ||
* }) | ||
* }) | ||
* ``` | ||
* | ||
* ### Aborting requests | ||
* | ||
* Most API methods accept an \[AbortSignal]\[] as part of an options object. Implementations may listen for an `abort` event emitted by this object, or test the `signal.aborted` property. When received implementations should tear down any long-lived requests or resources created. | ||
* | ||
* ### Concurrency | ||
* | ||
* The streaming `(put|get|delete)Many` methods are intended to be used with modules such as [it-parallel-batch](https://www.npmjs.com/package/it-parallel-batch) to allow calling code to control levels of parallelisation. The batching method ensures results are returned in the correct order, but interface implementations should be thread safe. | ||
* | ||
* ```js | ||
* import batch from 'it-parallel-batch' | ||
* const source = [{ | ||
* key: .., | ||
* value: .. | ||
* }] | ||
* | ||
* // put values into the datastore concurrently, max 10 at a time | ||
* for await (const { key, data } of batch(store.putMany(source), 10)) { | ||
* console.info(`Put ${key}`) | ||
* } | ||
* ``` | ||
* | ||
* ### Keys | ||
* | ||
* To allow a better abstraction on how to address values, there is a `Key` class which is used as identifier. It's easy to create a key from a `Uint8Array` or a `string`. | ||
* | ||
* ```js | ||
* const a = new Key('a') | ||
* const b = new Key(new Uint8Array([0, 1, 2, 3])) | ||
* ``` | ||
* | ||
* The key scheme is inspired by file systems and Google App Engine key model. Keys are meant to be unique across a system. They are typically hierarchical, incorporating more and more specific namespaces. Thus keys can be deemed 'children' or 'ancestors' of other keys: | ||
* | ||
* - `new Key('/Comedy')` | ||
* - `new Key('/Comedy/MontyPython')` | ||
* | ||
* Also, every namespace can be parameterized to embed relevant object information. For example, the Key `name` (most specific namespace) could include the object type: | ||
* | ||
* - `new Key('/Comedy/MontyPython/Actor:JohnCleese')` | ||
* - `new Key('/Comedy/MontyPython/Sketch:CheeseShop')` | ||
* - `new Key('/Comedy/MontyPython/Sketch:CheeseShop/Character:Mousebender')` | ||
*/ | ||
import { Key } from './key.js'; | ||
@@ -8,5 +105,5 @@ import type { Await, AwaitIterable, Store, AbortOptions } from 'interface-store'; | ||
export interface Batch<BatchOptionsExtension = {}> { | ||
put: (key: Key, value: Uint8Array) => void; | ||
delete: (key: Key) => void; | ||
commit: (options?: AbortOptions & BatchOptionsExtension) => Await<void>; | ||
put(key: Key, value: Uint8Array): void; | ||
delete(key: Key): void; | ||
commit(options?: AbortOptions & BatchOptionsExtension): Await<void>; | ||
} | ||
@@ -29,3 +126,3 @@ export interface Datastore<HasOptionsExtension = {}, PutOptionsExtension = {}, PutManyOptionsExtension = {}, GetOptionsExtension = {}, GetManyOptionsExtension = {}, DeleteOptionsExtension = {}, DeleteManyOptionsExtension = {}, QueryOptionsExtension = {}, QueryKeysOptionsExtension = {}, BatchOptionsExtension = {}> extends Store<Key, Uint8Array, Pair, HasOptionsExtension, PutOptionsExtension, PutManyOptionsExtension, GetOptionsExtension, GetManyOptionsExtension, DeleteOptionsExtension, DeleteManyOptionsExtension> { | ||
*/ | ||
batch: () => Batch<BatchOptionsExtension>; | ||
batch(): Batch<BatchOptionsExtension>; | ||
/** | ||
@@ -44,3 +141,3 @@ * Query the datastore. | ||
*/ | ||
query: (query: Query, options?: AbortOptions & QueryOptionsExtension) => AwaitIterable<Pair>; | ||
query(query: Query, options?: AbortOptions & QueryOptionsExtension): AwaitIterable<Pair>; | ||
/** | ||
@@ -59,3 +156,3 @@ * Query the datastore. | ||
*/ | ||
queryKeys: (query: KeyQuery, options?: AbortOptions & QueryKeysOptionsExtension) => AwaitIterable<Key>; | ||
queryKeys(query: KeyQuery, options?: AbortOptions & QueryKeysOptionsExtension): AwaitIterable<Key>; | ||
} | ||
@@ -62,0 +159,0 @@ export interface QueryFilter { |
@@ -6,4 +6,101 @@ /* eslint-disable @typescript-eslint/ban-types */ | ||
// https://github.com/typescript-eslint/typescript-eslint/issues/2063#issuecomment-675156492 | ||
/** | ||
* @packageDocumentation | ||
* | ||
* A Datastore is a key/value database that lets store/retrieve binary blobs using namespaced Keys. | ||
* | ||
* It is used by IPFS to store/retrieve arbitrary metadata needed to run the node - DHT provider records, signed peer records, etc. | ||
* | ||
* ## Backed Implementations | ||
* | ||
* - File System: [`datastore-fs`](https://github.com/ipfs/js-stores/tree/main/packages/datastore-fs) | ||
* - IndexedDB: [`datastore-idb`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-idb) | ||
* - level: [`datastore-level`](https://github.com/ipfs/js-stores/tree/main/packages/datastore-level) (supports any levelup compatible backend) | ||
* - Memory: [`datastore-core/memory`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/memory.ts) | ||
* - S3: [`datastore-s3`](https://github.com/ipfs/js-stores/tree/main/packages/datastore-s3) | ||
* | ||
* ## Wrapper Implementations | ||
* | ||
* - Keytransform: [`datstore-core/src/keytransform`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/keytransform.ts) | ||
* - Mount: [`datastore-core/src/mount`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/mount.ts) | ||
* - Namespace: [`datastore-core/src/namespace`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/namespace.ts) | ||
* - Sharding: [`datastore-core/src/sharding`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/sharding.ts) | ||
* - Tiered: [`datstore-core/src/tiered`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/tiered.ts) | ||
* | ||
* If you want the same functionality as [go-ds-flatfs](https://github.com/ipfs/go-ds-flatfs), use sharding with fs. | ||
* | ||
* @example | ||
* | ||
* ```js | ||
* import FsStore from 'datastore-fs' | ||
* import { ShardingDataStore, shard } from 'datastore-core' | ||
* | ||
* const fs = new FsStore('path/to/store') | ||
* | ||
* // flatfs now works like go-flatfs | ||
* const flatfs = await ShardingStore.createOrOpen(fs, new shard.NextToLast(2)) | ||
* ``` | ||
* | ||
* ### Test suite | ||
* | ||
* Available via the [`interface-datastore-tests`](https://npmjs.com/package/interface-datastore-tests) module | ||
* | ||
* ```js | ||
* import { interfaceDatastoreTests } from 'interface-datastore-tests' | ||
* | ||
* describe('mystore', () => { | ||
* interfaceDatastoreTests({ | ||
* async setup () { | ||
* return instanceOfMyStore | ||
* }, | ||
* async teardown () { | ||
* // cleanup resources | ||
* } | ||
* }) | ||
* }) | ||
* ``` | ||
* | ||
* ### Aborting requests | ||
* | ||
* Most API methods accept an \[AbortSignal]\[] as part of an options object. Implementations may listen for an `abort` event emitted by this object, or test the `signal.aborted` property. When received implementations should tear down any long-lived requests or resources created. | ||
* | ||
* ### Concurrency | ||
* | ||
* The streaming `(put|get|delete)Many` methods are intended to be used with modules such as [it-parallel-batch](https://www.npmjs.com/package/it-parallel-batch) to allow calling code to control levels of parallelisation. The batching method ensures results are returned in the correct order, but interface implementations should be thread safe. | ||
* | ||
* ```js | ||
* import batch from 'it-parallel-batch' | ||
* const source = [{ | ||
* key: .., | ||
* value: .. | ||
* }] | ||
* | ||
* // put values into the datastore concurrently, max 10 at a time | ||
* for await (const { key, data } of batch(store.putMany(source), 10)) { | ||
* console.info(`Put ${key}`) | ||
* } | ||
* ``` | ||
* | ||
* ### Keys | ||
* | ||
* To allow a better abstraction on how to address values, there is a `Key` class which is used as identifier. It's easy to create a key from a `Uint8Array` or a `string`. | ||
* | ||
* ```js | ||
* const a = new Key('a') | ||
* const b = new Key(new Uint8Array([0, 1, 2, 3])) | ||
* ``` | ||
* | ||
* The key scheme is inspired by file systems and Google App Engine key model. Keys are meant to be unique across a system. They are typically hierarchical, incorporating more and more specific namespaces. Thus keys can be deemed 'children' or 'ancestors' of other keys: | ||
* | ||
* - `new Key('/Comedy')` | ||
* - `new Key('/Comedy/MontyPython')` | ||
* | ||
* Also, every namespace can be parameterized to embed relevant object information. For example, the Key `name` (most specific namespace) could include the object type: | ||
* | ||
* - `new Key('/Comedy/MontyPython/Actor:JohnCleese')` | ||
* - `new Key('/Comedy/MontyPython/Sketch:CheeseShop')` | ||
* - `new Key('/Comedy/MontyPython/Sketch:CheeseShop/Character:Mousebender')` | ||
*/ | ||
import { Key } from './key.js'; | ||
export { Key }; | ||
//# sourceMappingURL=index.js.map |
{ | ||
"name": "interface-datastore", | ||
"version": "8.2.5", | ||
"version": "8.2.6", | ||
"description": "datastore interface", | ||
@@ -57,2 +57,3 @@ "license": "Apache-2.0 OR MIT", | ||
"parserOptions": { | ||
"project": true, | ||
"sourceType": "module" | ||
@@ -165,4 +166,4 @@ } | ||
"devDependencies": { | ||
"aegir": "^40.0.8" | ||
"aegir": "^41.1.9" | ||
} | ||
} |
139
README.md
@@ -1,3 +0,1 @@ | ||
# interface-datastore <!-- omit in toc --> | ||
[](https://ipfs.tech) | ||
@@ -10,18 +8,4 @@ [](https://discuss.ipfs.tech) | ||
## Table of contents <!-- omit in toc --> | ||
# Install | ||
- [Install](#install) | ||
- [Browser `<script>` tag](#browser-script-tag) | ||
- [Implementations](#implementations) | ||
- [Test suite](#test-suite) | ||
- [Aborting requests](#aborting-requests) | ||
- [Concurrency](#concurrency) | ||
- [Keys](#keys) | ||
- [API](#api) | ||
- [API Docs](#api-docs) | ||
- [License](#license) | ||
- [Contribute](#contribute) | ||
## Install | ||
```console | ||
@@ -31,3 +15,3 @@ $ npm i interface-datastore | ||
### Browser `<script>` tag | ||
## Browser `<script>` tag | ||
@@ -40,98 +24,7 @@ Loading this module through a script tag will make it's exports available as `InterfaceDatastore` in the global namespace. | ||
## Implementations | ||
# API Docs | ||
- Backed Implementations | ||
- File System: [`datastore-fs`](https://github.com/ipfs/js-stores/tree/main/packages/datastore-fs) | ||
- IndexedDB: [`datastore-idb`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-idb) | ||
- level: [`datastore-level`](https://github.com/ipfs/js-stores/tree/main/packages/datastore-level) (supports any levelup compatible backend) | ||
- Memory: [`datastore-core/memory`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/memory.ts) | ||
- S3: [`datastore-s3`](https://github.com/ipfs/js-stores/tree/main/packages/datastore-s3) | ||
- Wrapper Implementations | ||
- Keytransform: [`datstore-core/src/keytransform`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/keytransform.ts) | ||
- Mount: [`datastore-core/src/mount`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/mount.ts) | ||
- Namespace: [`datastore-core/src/namespace`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/namespace.ts) | ||
- Sharding: [`datastore-core/src/sharding`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/sharding.ts) | ||
- Tiered: [`datstore-core/src/tiered`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/tiered.ts) | ||
If you want the same functionality as [go-ds-flatfs](https://github.com/ipfs/go-ds-flatfs), use sharding with fs. | ||
```js | ||
import FsStore from 'datastore-fs' | ||
import { ShardingDataStore, shard } from 'datastore-core' | ||
const fs = new FsStore('path/to/store') | ||
// flatfs now works like go-flatfs | ||
const flatfs = await ShardingStore.createOrOpen(fs, new shard.NextToLast(2)) | ||
``` | ||
### Test suite | ||
Available via the [`interface-datastore-tests`](https://npmjs.com/package/interface-datastore-tests) module | ||
```js | ||
import { interfaceDatastoreTests } from 'interface-datastore-tests' | ||
describe('mystore', () => { | ||
interfaceDatastoreTests({ | ||
async setup () { | ||
return instanceOfMyStore | ||
}, | ||
async teardown () { | ||
// cleanup resources | ||
} | ||
}) | ||
}) | ||
``` | ||
### Aborting requests | ||
Most API methods accept an \[AbortSignal]\[] as part of an options object. Implementations may listen for an `abort` event emitted by this object, or test the `signal.aborted` property. When received implementations should tear down any long-lived requests or resources created. | ||
### Concurrency | ||
The streaming `(put|get|delete)Many` methods are intended to be used with modules such as [it-parallel-batch](https://www.npmjs.com/package/it-parallel-batch) to allow calling code to control levels of parallelisation. The batching method ensures results are returned in the correct order, but interface implementations should be thread safe. | ||
```js | ||
import batch from 'it-parallel-batch' | ||
const source = [{ | ||
key: .., | ||
value: .. | ||
}] | ||
// put values into the datastore concurrently, max 10 at a time | ||
for await (const { key, data } of batch(store.putMany(source), 10)) { | ||
console.info(`Put ${key}`) | ||
} | ||
``` | ||
### Keys | ||
To allow a better abstraction on how to address values, there is a `Key` class which is used as identifier. It's easy to create a key from a `Uint8Array` or a `string`. | ||
```js | ||
const a = new Key('a') | ||
const b = new Key(new Uint8Array([0, 1, 2, 3])) | ||
``` | ||
The key scheme is inspired by file systems and Google App Engine key model. Keys are meant to be unique across a system. They are typically hierarchical, incorporating more and more specific namespaces. Thus keys can be deemed 'children' or 'ancestors' of other keys: | ||
- `new Key('/Comedy')` | ||
- `new Key('/Comedy/MontyPython')` | ||
Also, every namespace can be parameterized to embed relevant object information. For example, the Key `name` (most specific namespace) could include the object type: | ||
- `new Key('/Comedy/MontyPython/Actor:JohnCleese')` | ||
- `new Key('/Comedy/MontyPython/Sketch:CheeseShop')` | ||
- `new Key('/Comedy/MontyPython/Sketch:CheeseShop/Character:Mousebender')` | ||
## API | ||
<https://ipfs.github.io/interface-datastore/> | ||
## API Docs | ||
- <https://ipfs.github.io/js-stores/modules/interface_datastore.html> | ||
## License | ||
# License | ||
@@ -143,3 +36,3 @@ Licensed under either of | ||
## Contribute | ||
# Contribute | ||
@@ -155,23 +48,1 @@ Contributions welcome! Please check out [the issues](https://github.com/ipfs/js-stores/issues). | ||
[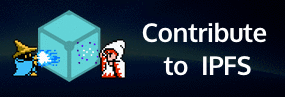](https://github.com/ipfs/community/blob/master/CONTRIBUTING.md) | ||
[Key]: #Keys | ||
[Object]: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object | ||
[Uint8Array]: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Uint8Array | ||
[AbortSignal]: https://developer.mozilla.org/en-US/docs/Web/API/AbortSignal | ||
[AsyncIterator]: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Symbol/asyncIterator | ||
[AsyncIterable]: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Iteration_protocols | ||
[String]: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String | ||
[Array]: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array | ||
[Function]: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Function | ||
[Number]: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Number | ||
[Boolean]: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Boolean |
110
src/index.ts
@@ -7,2 +7,100 @@ /* eslint-disable @typescript-eslint/ban-types */ | ||
/** | ||
* @packageDocumentation | ||
* | ||
* A Datastore is a key/value database that lets store/retrieve binary blobs using namespaced Keys. | ||
* | ||
* It is used by IPFS to store/retrieve arbitrary metadata needed to run the node - DHT provider records, signed peer records, etc. | ||
* | ||
* ## Backed Implementations | ||
* | ||
* - File System: [`datastore-fs`](https://github.com/ipfs/js-stores/tree/main/packages/datastore-fs) | ||
* - IndexedDB: [`datastore-idb`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-idb) | ||
* - level: [`datastore-level`](https://github.com/ipfs/js-stores/tree/main/packages/datastore-level) (supports any levelup compatible backend) | ||
* - Memory: [`datastore-core/memory`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/memory.ts) | ||
* - S3: [`datastore-s3`](https://github.com/ipfs/js-stores/tree/main/packages/datastore-s3) | ||
* | ||
* ## Wrapper Implementations | ||
* | ||
* - Keytransform: [`datstore-core/src/keytransform`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/keytransform.ts) | ||
* - Mount: [`datastore-core/src/mount`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/mount.ts) | ||
* - Namespace: [`datastore-core/src/namespace`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/namespace.ts) | ||
* - Sharding: [`datastore-core/src/sharding`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/sharding.ts) | ||
* - Tiered: [`datstore-core/src/tiered`](https://github.com/ipfs/js-stores/blob/main/packages/datastore-core/src/tiered.ts) | ||
* | ||
* If you want the same functionality as [go-ds-flatfs](https://github.com/ipfs/go-ds-flatfs), use sharding with fs. | ||
* | ||
* @example | ||
* | ||
* ```js | ||
* import FsStore from 'datastore-fs' | ||
* import { ShardingDataStore, shard } from 'datastore-core' | ||
* | ||
* const fs = new FsStore('path/to/store') | ||
* | ||
* // flatfs now works like go-flatfs | ||
* const flatfs = await ShardingStore.createOrOpen(fs, new shard.NextToLast(2)) | ||
* ``` | ||
* | ||
* ### Test suite | ||
* | ||
* Available via the [`interface-datastore-tests`](https://npmjs.com/package/interface-datastore-tests) module | ||
* | ||
* ```js | ||
* import { interfaceDatastoreTests } from 'interface-datastore-tests' | ||
* | ||
* describe('mystore', () => { | ||
* interfaceDatastoreTests({ | ||
* async setup () { | ||
* return instanceOfMyStore | ||
* }, | ||
* async teardown () { | ||
* // cleanup resources | ||
* } | ||
* }) | ||
* }) | ||
* ``` | ||
* | ||
* ### Aborting requests | ||
* | ||
* Most API methods accept an \[AbortSignal]\[] as part of an options object. Implementations may listen for an `abort` event emitted by this object, or test the `signal.aborted` property. When received implementations should tear down any long-lived requests or resources created. | ||
* | ||
* ### Concurrency | ||
* | ||
* The streaming `(put|get|delete)Many` methods are intended to be used with modules such as [it-parallel-batch](https://www.npmjs.com/package/it-parallel-batch) to allow calling code to control levels of parallelisation. The batching method ensures results are returned in the correct order, but interface implementations should be thread safe. | ||
* | ||
* ```js | ||
* import batch from 'it-parallel-batch' | ||
* const source = [{ | ||
* key: .., | ||
* value: .. | ||
* }] | ||
* | ||
* // put values into the datastore concurrently, max 10 at a time | ||
* for await (const { key, data } of batch(store.putMany(source), 10)) { | ||
* console.info(`Put ${key}`) | ||
* } | ||
* ``` | ||
* | ||
* ### Keys | ||
* | ||
* To allow a better abstraction on how to address values, there is a `Key` class which is used as identifier. It's easy to create a key from a `Uint8Array` or a `string`. | ||
* | ||
* ```js | ||
* const a = new Key('a') | ||
* const b = new Key(new Uint8Array([0, 1, 2, 3])) | ||
* ``` | ||
* | ||
* The key scheme is inspired by file systems and Google App Engine key model. Keys are meant to be unique across a system. They are typically hierarchical, incorporating more and more specific namespaces. Thus keys can be deemed 'children' or 'ancestors' of other keys: | ||
* | ||
* - `new Key('/Comedy')` | ||
* - `new Key('/Comedy/MontyPython')` | ||
* | ||
* Also, every namespace can be parameterized to embed relevant object information. For example, the Key `name` (most specific namespace) could include the object type: | ||
* | ||
* - `new Key('/Comedy/MontyPython/Actor:JohnCleese')` | ||
* - `new Key('/Comedy/MontyPython/Sketch:CheeseShop')` | ||
* - `new Key('/Comedy/MontyPython/Sketch:CheeseShop/Character:Mousebender')` | ||
*/ | ||
import { Key } from './key.js' | ||
@@ -22,5 +120,5 @@ import type { | ||
export interface Batch<BatchOptionsExtension = {}> { | ||
put: (key: Key, value: Uint8Array) => void | ||
delete: (key: Key) => void | ||
commit: (options?: AbortOptions & BatchOptionsExtension) => Await<void> | ||
put(key: Key, value: Uint8Array): void | ||
delete(key: Key): void | ||
commit(options?: AbortOptions & BatchOptionsExtension): Await<void> | ||
} | ||
@@ -53,3 +151,3 @@ | ||
*/ | ||
batch: () => Batch<BatchOptionsExtension> | ||
batch(): Batch<BatchOptionsExtension> | ||
@@ -69,3 +167,3 @@ /** | ||
*/ | ||
query: (query: Query, options?: AbortOptions & QueryOptionsExtension) => AwaitIterable<Pair> | ||
query(query: Query, options?: AbortOptions & QueryOptionsExtension): AwaitIterable<Pair> | ||
@@ -85,3 +183,3 @@ /** | ||
*/ | ||
queryKeys: (query: KeyQuery, options?: AbortOptions & QueryKeysOptionsExtension) => AwaitIterable<Key> | ||
queryKeys(query: KeyQuery, options?: AbortOptions & QueryKeysOptionsExtension): AwaitIterable<Key> | ||
} | ||
@@ -88,0 +186,0 @@ |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Unidentified License
License(Experimental) Something that seems like a license was found, but its contents could not be matched with a known license.
Found 1 instance in 1 package
Unidentified License
License(Experimental) Something that seems like a license was found, but its contents could not be matched with a known license.
Found 1 instance in 1 package
84277
10.73%1612
22.31%44
-74.57%