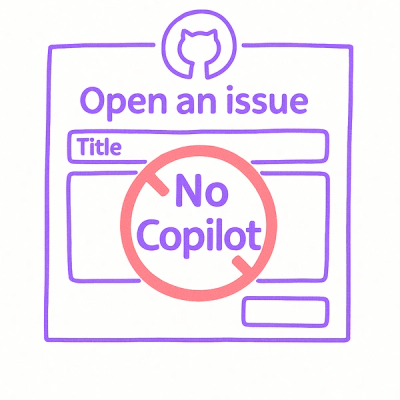
Security News
Open Source Maintainers Demand Ability to Block Copilot-Generated Issues and PRs
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
io-validate
Advanced tools
Runtime data validator.
param-check
.npm install io-validate --save
import v from 'io-validate';
function fn(arg1, arg2) {
v(arg1).isString();
// If you want to print variable name, pass name as the 2nd parameter.
v(arg2, 'arg2').greaterThan(1).lessThan(2);
}
not
operatorimport v from 'io-validate';
function fn(arg) {
v(arg).not.isString();
}
All validators of io-validate support chain calls. A chain call means an "and" expression.
import v from 'io-validate';
function fn(arg) {
v(arg).gt(1).lt(2); // arg > 1 && arg < 2
}
If you want smaller dependence size, just import specific validators.
import v from 'io-validate/minimum';
import isString from 'io-validate/lib/validators/isString';
v.register(isString);
function fn(arg) {
v(arg).isString();
}
import v from 'io-validate';
import isNumber from 'lodash/isNumber';
function isEven(target, name) {
return isNumber(target) && !(target % 2);
}
check.register('isEven', isEven);
function fn(arg) {
v(arg).isEven();
}
import v from 'io-validate'
function isEven (target, name) {
return !(target % 2)
}
function isOdd (target, name) {
return target % 2
}
function next (target) {
return return target + 1
}
v.register('isEven', isEven, next)
v.register('isOdd', isOdd, next)
function fn (arg) {
v(arg).isEven().isOdd()
}
plan is used to generate combinable validators.
By contrast, v('').isString()
executes the validator isString
immediatly, v.plan.isString()
doesn't execute any validator, just return a combinable isString
validator.
import v from 'io-validate';
v('').isString();
v('').or(v.plan.isString, v.plan.isNumber);
Validate that the input value is the same to ('===') the reference value.
v(1).same(1);
v({ a: 1 }).same({ a: 1 }); // Bang!
Validate that the input value is among the reference options.
v(1).among(1, 2, 3, 4);
Validate that the input value is equal to the reference value.
You may pass in a custom equal function instead of the default equal algorithm which is a simplified implemention of deep-equal.
v(1).equal(1);
v(1).eq(1);
v('foobar').equal('Foobar', (left, right) => left.toLowerCase() === right.toLowerCase());
Validate that the input number is equal to or greater than the reference number.
v(1).equalOrGreaterThan(0);
v(1).egt(1);
Validate that the input number is equal to or less than the reference number.
v(1).equalOrLessThan(2);
v(1).elt(1);
Validate that the input number is greater than the reference number.
v(1).greaterThan(0);
v(1).gt(0);
Validate that the input number is less than the reference number.
v(1).lessThan(2);
v(1).lt(2);
Validate that the input number is whthin the reference range(s).
Syntax:
v(1).within('(0, 2)', '[-1, 3.5]');
v(0).within('[0, 1)', '(-1, 0]');
Runtime type checking based on lodash.
v(1).is('number', 'string') // number or string
v(1).isNumber()
var isArguments = require('lodash/isArguments');
var isArray = require('lodash/isArray');
var isArrayBuffer = require('lodash/isArrayBuffer');
var isArrayLike = require('lodash/isArrayLike');
var isArrayLikeObject = require('lodash/isArrayLikeObject');
var isBoolean = require('lodash/isBoolean');
var isBuffer = require('lodash/isBuffer');
var isDate = require('lodash/isDate');
var isElement = require('lodash/isElement');
var isEmpty = require('lodash/isEmpty');
var isError = require('lodash/isError');
var isFunction = require('lodash/isFunction');
var isLength = require('lodash/isLength');
var isMap = require('lodash/isMap');
var isNative = require('lodash/isNative');
var isNil = require('lodash/isNil');
var isNull = require('lodash/isNull');
var isNumber = require('lodash/isNumber');
var isObject = require('lodash/isObject');
var isObjectLike = require('lodash/isObjectLike');
var isPlainObject = require('lodash/isPlainObject');
var isRegExp = require('lodash/isRegExp');
var isSet = require('lodash/isSet');
var isString = require('lodash/isString');
var isSymbol = require('lodash/isSymbol');
var isTypedArray = require('lodash/isTypedArray');
var isUndefined = require('lodash/isUndefined');
var isWeakMap = require('lodash/isWeakMap');
var isWeakSet = require('lodash/isWeakSet');
var isExist = function isExist(o) {
return !(isUndefined(o) || isNull(o));
};
Validate that the input value is an instance of the reference class.
We use the keyword 'instanceof' to implement this validator.
class Foobar {}
const fb = new Foobar();
v(fb).instanceOf(fb);
Validate that the input value is an array of valid values.
v(fb).isArrayOf(fb, (el) => el % 2);
Regular expression validator.
v('foobar42').match(/\w+\d+/);
Common regular expression validators.
v('yusangeng@github.com').matchEmail();
Validate that the input value has an OWN property of the reference name.
has() returns a validatable object that encapsulates the validated property if it exists.
If you want the validatable object that encapsulates the origin input value, use "owner" or "_" operator.
const obj = {
a: {
b: {
c: 'foobar',
},
},
};
v(obj).has('a').has('b').has('c');
v(obj).has('a').owner.has('a').owner.has('c');
v(obj).has('a')._.has('a')._.has('a');
Validate that the input value has a property (maybe NOT own property) of the reference name.
const obj = {
a: {
b: {
c: 'foobar',
},
},
};
v(obj).got('a').got('b').got('c');
v(obj).got('a').got('__proto__').got('constructor');
Equivalent of validator got('length')
.
const arr = [1, 2, 3];
v(arr).length().eq(3);
Validator of "and" expression.
It is often better to express "and" relationships using chain calls, but this API combines functions and plans.
v('Foobar').and(v.plan.isString, (target) => {
return target.toLowerCase() === 'foobar';
});
Validator of "or" expression.
v(1).or(
v.plan.isString,
v.plan.and(v.plan.isNumber(), (target) => target % 2)
);
Single parameter version of validator and()
.
v('Foobar').meet(v.plan.isString);
FAQs
Runtime data validator.
The npm package io-validate receives a total of 2 weekly downloads. As such, io-validate popularity was classified as not popular.
We found that io-validate demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
Research
Security News
Malicious Koishi plugin silently exfiltrates messages with hex strings to a hardcoded QQ account, exposing secrets in chatbots across platforms.
Research
Security News
Malicious PyPI checkers validate stolen emails against TikTok and Instagram APIs, enabling targeted account attacks and dark web credential sales.