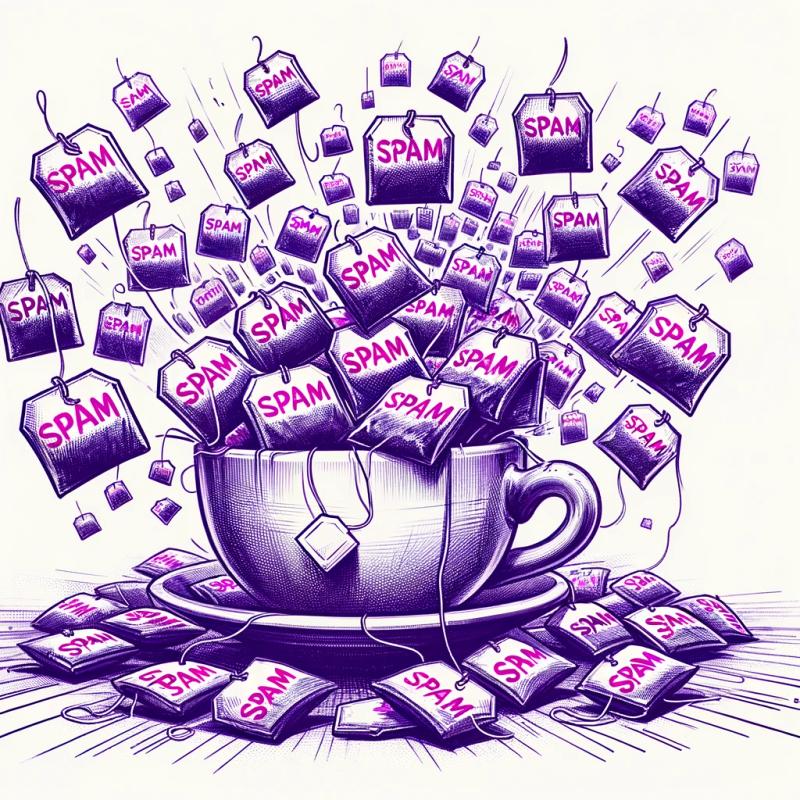
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
ionic-modal-select
Advanced tools
Readme
Modal select for Ionic Framework based on $ionicModal
See all docs and examples on the project site.
We also have a simple Codepen demo.
In order to survive, this project needs:
Any help on this is greatly appreciated. Comment directly those issues or contact me directly at mauro.bianchi at inmagik.com if you are interested in helping with this.
Get the files from github or install from bower:
bower install ionic-modal-select
Include ionic-modal-select.js
or its minified version in your index.html:
<script src="lib/ionic-modal-select/dist/ionic-modal-select.js"></script>
Add the module ionic-modal-select
to your application dependencies:
angular.module('starter', ['ionic', 'ionic-modal-select'])
And you're ready to go.
This directive will transform the element into a modal select: when clicking the element a select dialog will be open, with options presented in a clickable list. Once the user clicks on an option, it will be set on the bound model.
For this to work the following conditions must apply:
The final value bound to your model will be determined as follow:
option-getter
will be set as getterFunction(selectedItem)
option-property
will be set as selectedItem[propertyName]
option | meaning | accepted values | default |
---|---|---|---|
options | List of options to choose from | Array | |
options-expression | The expression indicating how to enumerate a the options collection, of the format variable in expression – where variable is the user defined loop variable and expression is a scope expression giving the collection to enumerate. For example: `album in artist.albums or album in artist.albums | orderBy:'name'`. | expression |
option-getter | Optional method to get the value from the chosen item | function | not set |
option-property | Optional property name to get as model value from the chosen item | string | not set |
modal-class | The class for the modal (set on <ion-modal-view> | string | '' |
selected-class | The class applied to the currently selected option (if any) in the modal list | string | 'option-selected' |
on-select | Callback triggered on object select. Takes two arguments, newValue and oldValue with obvious meaning. | function call with arguments newValue and oldValue | not set |
on-reset | Callback triggered when value is resetted using the relevant ui interface. Takes no arguments. | function call | not set |
modal-title | The title shown on the modal header | string | 'Select an option' |
header-footer-class | The class for header and footer of the modal | string | 'bar-stable' |
cancel-button | Text of the button for closing the modal without changing the value | string | 'Cancel' |
reset-button | Text of the button for unsetting value in the modal dialog | string | 'Reset' |
hide-reset | Hides the button for unsetting value in the modal dialog | string. Set to 'true' for hiding the button | false |
use-collection-repeat | Forces use of collection-repeat or ng-repeat for rendering options in the modal. | string "true", "false" | not set (automatically set according to number of options and short-list-break attribute) |
short-list-break | The maximum number of item in list to be rendered with ng-repeat .(if use-collection-repeat is not set) If the list has a number of options greater than this attribute it will be rendered with ionic collection-repeat directive instead. (see also load-list-message option) | integer | 10 |
load-list-message | Message to be shown when loading a long list of options in the modal | string | 'Loading' |
has-search | Whether to show a search bar to filter options. | set to "true" for showing the search bar | undefined |
search-placeholder | String placeholder in search bar. | string | 'Search' |
sub-header-class | Class to be applied to the subheader containing the search bar (makes sense only if has-search="true ) | string | 'bar-stable' |
cancel-search-button | Text for the button for clearing search text (makes sense only if has-search="true ) | string | 'Clear' |
clear-search-on-select | Tells the directive to not clear the search bar content after user selection. Set to false to prevent clearing the search text. | boolean | true |
search-properties | Array of properties for the search. For example: In your controller $scope.searchProperties = ['property1', 'property2']; and in template attributes search-properties="searchProperties" | Array |
The modal-select
directive must be provided with a set of options to choose from
This can be done in two ways:
options
attribute, that accepts an array of values or objects. The directive will watch for changes in this array and modify its options accordingly.options-expression
attribute, that accepts an expression similar to what you would use with ionic collection-repeat
directive, of the format variable in expression
– where variable is the user defined loop variable and expression is a scope expression giving the collection to enumerate. For example: album in artist.albums or album in artist.albums | orderBy:'name'
. This allows you to apply ordering or filtering without acting on the original array.This directive expects to find a single inner element of class "option" that is used to define the template of the options that can be selected. Options will be rendered as items into a list in the modal (The content of each option, rendered with your template, is wrapped in an element of class 'item item-text wrap' and the original ".option" element is removed).
For example:
<button class="button button-positive" modal-select ng-model="someModel" options="selectables" modal-title="Select a number">
Select it
<div class="option">
{{option}}
</div>
</button>
Will be rendered in the modal as :
<div class="item item-text-wrap">
{{option}}
</div>
From version 1.1.0 you can include a search bar into the modal for filtering options by simply adding the attribute has-search="true"
to your modal-select
element.
Filtering is implemented with the angular filter
filter, which searches recursively in all properties of the objects passed in as options. This means that you cannot search on "computed properties" right now. For example if you are using a custom setter you will be only able to search the original properties of the options.
### Examples
This example shows a modal for choosing a number between 1 and 5.
In your controller:
$scope.selectables = [1,2,3,4,5];
In your template:
<button class="button button-positive" modal-select ng-model="someModel" options="selectables" modal-title="Select a number">
Select it
<div class="option">
{{option}}
</div>
</button>
To include a search bar in the previous example, just add has-search="true"
:
<button class="button button-positive" modal-select ng-model="someModel" options="selectables" modal-title="Select a number" has-search="true">
Select it
<div class="option">
{{option}}
</div>
</button>
In the following example we use some objects as options.
In your controller:
$scope.selectables = [
{ name: "Mauro", role : "navigator"},
{ name: "Silvia", role : "chef"},
{ name: "Merlino", role : "canaglia"}
];
We'll explore different possibilities we have with this options.
If we do not set option-getter
or option-property
attributes, the model is assigned to the full option object when an option is selected.
<button class="button button-positive" modal-select ng-model="someModel" options="selectables" modal-title="Select a character">
Select it
<div class="option">
{{option.name}} ({{option.role}})
</div>
</button>
If option-property
attribute is set to a string, the bound model assigned that property of the option object when an option is selected. For example if we set option-getter="name"
, we get back the 'name' property of our options.
<button class="button button-positive" modal-select ng-model="someModel" options="selectables" modal-title="Select a character" option-property="name">
Select it
<div class="option">
{{option.name}} ({{option.role}})
</div>
</button>
If a function call is passed via option-getter
attribute, the bound model assignment is done by calling this function with the selected option as the only argument (named 'option'). For example if we do this in our controller:
$scope.getOption = function(option){
return option.name + ":" + option.role;
};
<button class="button button-positive" modal-select ng-model="someModel" options="selectables" modal-title="Select a character" option-getter="getOption(option)">
Select it
<div class="option">
{{option.name}} ({{option.role}})
</div>
</button>
Specify in the array the properties' name for search $scope.search_properties = ['propertie_1', 'propertie_2', '...'];
:
$scope.search_properties = ['name'];
<button class="button button-positive" modal-select ng-model="someModel" options="selectables" modal-title="Select a character" option-getter="getOption(option)" search-properties="search_properties">
Select it
<div class="option">
{{option.name}} ({{option.role}})
</div>
</button>
We get back the phrase "Mauro:navigator", "Silvia:chef" or "Merlino:canaglia" if we click the previous defined options.
This software package is available for free with a MIT license, but if you find it useful and want support its development consider buying a copy on the Ionic Marketplace for just a few bucks.
FAQs
Modal select for ionic
The npm package ionic-modal-select receives a total of 8 weekly downloads. As such, ionic-modal-select popularity was classified as not popular.
We found that ionic-modal-select demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.