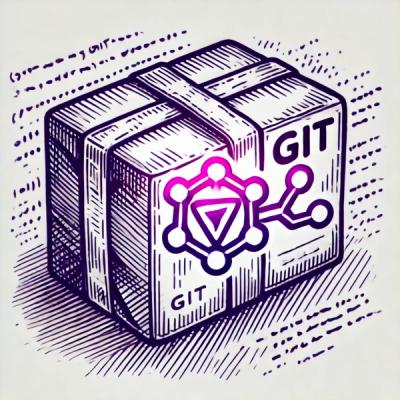
Security News
How to Mitigate the Risks of Using Open Source Packages with Git Dependencies
Git dependencies in open source packages can introduce significant risks, including lack of version control, stability issues, dependency drift, and difficulty in auditing, making them potential targets for supply chain attacks.