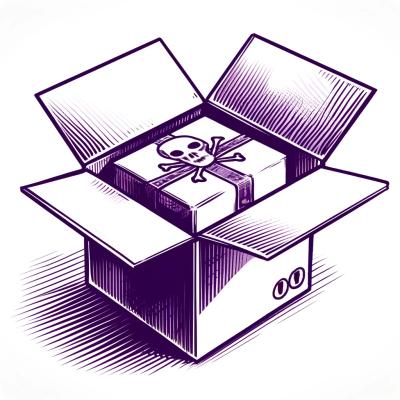
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
is-function
Advanced tools
The is-function npm package is a simple utility used for checking if a given value is a function. It is particularly useful in JavaScript environments where determining the type of an object can sometimes be less straightforward due to the dynamic nature of the language. This package provides a reliable way to ensure that a variable holds a function before attempting to invoke it, thus preventing runtime errors in code.
Function Type Checking
This feature allows developers to check if a variable is a function. It supports various types of functions including regular functions, async functions, and arrow functions. It returns true if the variable is a function and false otherwise. This is particularly useful for validating callbacks or any variable that is expected to be a function before invoking it.
const isFunction = require('is-function');
console.log(isFunction(function() {})); // true
console.log(isFunction(async () => {})); // true
console.log(isFunction(() => {})); // true
console.log(isFunction(class MyClass {})); // false
console.log(isFunction({})); // false
lodash.isfunction is a part of the Lodash library, which is a broader utility library for JavaScript. While is-function focuses solely on checking if a value is a function, lodash.isfunction offers this functionality within the context of a larger set of utility functions. Lodash's isFunction method may be preferred in projects that already use Lodash for other utilities.
kind-of is a more general type checking library that can determine the type of a given value, including whether it is a function. Unlike is-function, which is specialized for function type checking, kind-of can be used to check a wide variety of types such as strings, numbers, objects, etc. This makes kind-of a more versatile choice if your project requires extensive type checking beyond just functions.
Is that thing a function? Use this module to find out.
Return true
if fn
is a function, otherwise false
.
Because certain old browsers misreport the type of RegExp
objects as functions.
I stole this from https://github.com/ljharb/object-keys
MIT
FAQs
is that thing a function? Use this module to find out
The npm package is-function receives a total of 3,189,712 weekly downloads. As such, is-function popularity was classified as popular.
We found that is-function demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.