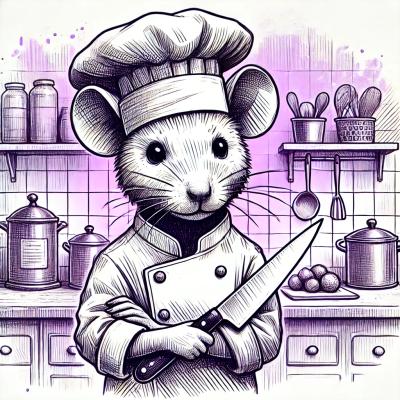
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
The is-subset npm package is a utility that allows you to check if one set of values is a subset of another. This can be particularly useful for validating data structures, filtering data, and performing set operations in JavaScript.
Basic Subset Check
This feature allows you to check if one object is a subset of another. In this example, object `b` is a subset of object `a` because all key-value pairs in `b` exist in `a`.
const isSubset = require('is-subset');
const a = { foo: 'bar', baz: 42 };
const b = { foo: 'bar' };
console.log(isSubset(a, b)); // true
Array Subset Check
This feature allows you to check if one array is a subset of another. In this example, array `b` is a subset of array `a` because all elements in `b` exist in `a`.
const isSubset = require('is-subset');
const a = [1, 2, 3, 4];
const b = [2, 3];
console.log(isSubset(a, b)); // true
Nested Object Subset Check
This feature allows you to check if one nested object is a subset of another. In this example, object `b` is a subset of object `a` because the nested structure and values in `b` exist in `a`.
const isSubset = require('is-subset');
const a = { foo: { bar: 'baz' }, qux: 42 };
const b = { foo: { bar: 'baz' } };
console.log(isSubset(a, b)); // true
Lodash's `isEqual` function can be used to perform deep comparisons between two values to determine if they are equivalent. While it is more general-purpose and can compare any two values for equality, it does not specifically check for subset relationships.
Underscore.js provides a variety of utility functions for JavaScript, including `_.isMatch`, which can be used to check if an object contains certain key-value pairs. This is similar to checking for subsets but is more focused on object properties.
The `deep-equal` package allows for deep comparison between two values to determine if they are equivalent. Like `lodash.isequal`, it is more general-purpose and does not specifically check for subset relationships.
Check if an object is contained within another one.
$ npm install is-subset
import isSubset from 'is-subset/module';
// …or:
var isSubset = require('is-subset');
isSubset(
{a: 1, b: 2},
{a: 1}
);
isSubset(
{a: 1, b: {c: 3, d: 4}, e: 5},
{a: 1, b: {c: 3}}
);
isSubset(
{a: 1, bcd: [1, 2, 3]},
{a: 1, bcd: [1, 2]}
);
…and these are false:
isSubset(
{a: 1},
{a: 2}
);
isSubset(
{a: 1},
{a: 1, b: 2}
);
isSubset(
{a: 1, bcd: [1, 2, 3]},
{a: 1, bcd: [1, 3]}
);
See the specs for more info.
Check if an object is contained within another object.
Returns true
if:
Parameters:
Object
supersetObject
subsetReturn value:
Boolean
FAQs
Check if an object is contained within another one
We found that is-subset demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.