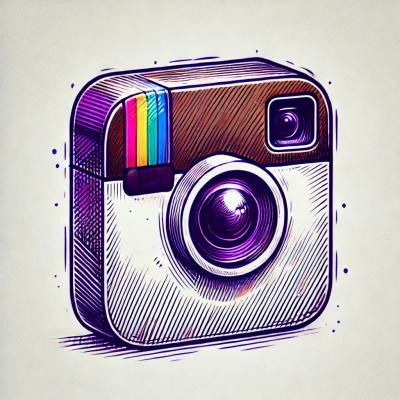
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
iteragain
Advanced tools
Another Javascript library for iterating. Available on npm.js and jsr.io.
Pure JavaScript, Iterable/Iterator/Generator-function utilities. No dependencies and shipped with types as is.
Iterators and Iterables in Javascript has basically no supporting methods or functions. This package provides easy to use, lazy evaluation methods to save on memory and unnecessary processing. Support with using the already existing next()
method and usage in "for of" loops, this makes using Iterators as easy as using an Array.
This package can be used like in Python with standalone functions like map, filter, etc. Or be used with method chaining by calling iter which returns an instance of the ExtendedIterator class. Either is supported for however you want to handle iterators.
You can see the full list of modules and the documentation on everything here.
View the full list of changes here.
// This is an example of using Array higher-order methods.
// And this particular one will iterate over `someArray` 3 times.
const result1 = someArray.map(iteratee).filter(predicate).reduce(reducer);
// This example of using `iter` returns the same result, but it will only iterate over `someArray` once.
const result2 = iter(someArray).map(iteratee).filter(predicate).reduce(reducer).toArray();
equal(result1, result2); // Asserts that the results are the same.
iter
and some standalone functions// Import from the root index
import { iter } from 'iteragain';
// Or from the file itself (tree shakeable):
import iter from 'iteragain/iter';
let nums = iter([1, 2, 3, 4, 5])
.map(n => n * n)
.filter(n => n % 2 === 0)
.toArray();
// [4, 16]
// Or use the standalone functions instead of `iter`:
import { map, filter, toArray } from 'iteragain';
nums = toArray(
filter(
map([1, 2, 3, 4, 5], n => n * n),
n => n % 2 === 0,
),
); // [4, 16]
range
standalone functionimport range from 'iteragain/range';
range(10).toArray(); // [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
range(10, 0).toArray(); // [10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
range(0, -10).toArray(); // [0, -1, -2, -3, -4, -5, -6, -7, -8, -9]
range(0, 10, 2).toArray(); // [0, 2, 4, 6, 8]
let r = range(3);
const nums = [...r, ...r]; // [0, 1, 2, 0, 1, 2], can be reused after a full iteration.
r = range(0, 10, 2);
// The following methods do not iterate/access the internal iterator:
r.length; // 10, (a readonly property)
r.includes(4); // true
r.nth(-1); // 8 (the last element)
r.nth(1); // 2 (the second element)
r.nth(10); // undefined (the 10th element doesn't exist)
r.index(4); // 2 (the index of the value 4)
import iter from 'iteragain/iter';
const obj = { a: 1, b: { c: 2, d: { e: 3 }, f: 4 } };
const keys = iter(obj)
.map(([key, _value, _parent]) => key)
.toArray();
// ['a', 'c', 'e', 'd', 'b', 'f'] Post-order depth first search traversal of `obj`.
import iter from 'iteragain/iter';
const it = iter(() => someFunction(1, 2, 3), null);
// Will call `someFunction` with the arguments [1, 2, 3] for each iteration until it returns `null`:
const results = it.toArray();
The arrayLike
uses a Proxy that wraps a seekable iterator. This allows access to elements from the iterator. This can be useful when you may need to easily search behind the current element, then look ahead, or just access elements by index.
import { arrayLike, range, iter, toArray } from 'iteragain';
const arr = arrayLike(iter(range(100)).map(n => n * n)); // Cached elements: []
arr.length; // Returns 0
arr[4]; // Returns 16. Cached elements: [0, 1, 4, 9, 16]
arr.length; // returns 5
arr[9]; // Returns 81. Cached elements: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
arr.length; // Returns 10
// Negative indices are supported, because internally it's just a seekable.
// This will seek starting from the end of the internal cache.
arr[-1]; // Returns 81. Cached elements: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
arr.length; // Returns 10
// All other array methods are supported, as it's of type `readonly number[]`.
// Including using it as an iterable:
for (const n of arr) {
console.log(n);
if (n > 20) break;
}
// Exhausts the iterator wrapped inside of `arr`. `collected` is just a regular array.
const collected = toArray(arr);
iterplus, iterare, lodash, rxjs, ixjs and the Python itertools and more-itertools modules. See benchmark section for performance against some of these.
Starting benchmark suite: index.bm.ts
for of loop x 2,831 ops/sec, ±10 ops/sec or ±0.35% (98 runs sampled)
iteragain x 1,853 ops/sec, ±7 ops/sec or ±0.36% (98 runs sampled)
iteragain standalones x 1,740 ops/sec, ±27 ops/sec or ±1.57% (97 runs sampled)
iterare x 1,754 ops/sec, ±31 ops/sec or ±1.76% (96 runs sampled)
rxjs x 1,319 ops/sec, ±7 ops/sec or ±0.50% (96 runs sampled)
ixjs x 873 ops/sec, ±15 ops/sec or ±1.70% (95 runs sampled)
4.1.6 - 2025-02-10
join
method no longer returns undefined with an empty inputFAQs
Javascript Iterable/Iterator/Generator-function utilities.
The npm package iteragain receives a total of 0 weekly downloads. As such, iteragain popularity was classified as not popular.
We found that iteragain demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.