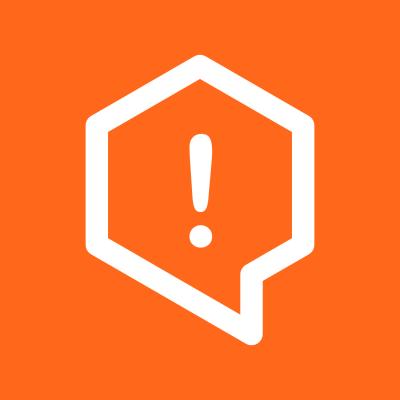
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
jira-client
Advanced tools
The jira-client npm package is a Node.js wrapper for the JIRA REST API. It allows developers to interact with JIRA programmatically, enabling them to automate tasks, retrieve data, and manage JIRA issues, projects, and other resources.
Create Issue
This feature allows you to create a new issue in JIRA. The code sample demonstrates how to configure the jira-client and create a new issue with specified fields such as project key, summary, description, and issue type.
const JiraClient = require('jira-client');
const jira = new JiraClient({
protocol: 'https',
host: 'your-jira-host.atlassian.net',
username: 'your-username',
password: 'your-password',
apiVersion: '2',
strictSSL: true
});
jira.addNewIssue({
fields: {
project: { key: 'TEST' },
summary: 'New issue from jira-client',
description: 'Creating an issue via jira-client',
issuetype: { name: 'Task' }
}
}).then(issue => {
console.log(`Created issue: ${issue.key}`);
}).catch(err => {
console.error(err);
});
Get Issue
This feature allows you to retrieve details of a specific issue by its key. The code sample shows how to configure the jira-client and fetch an issue using its key.
const JiraClient = require('jira-client');
const jira = new JiraClient({
protocol: 'https',
host: 'your-jira-host.atlassian.net',
username: 'your-username',
password: 'your-password',
apiVersion: '2',
strictSSL: true
});
jira.findIssue('TEST-1').then(issue => {
console.log(`Found issue: ${issue.key}`);
console.log(issue);
}).catch(err => {
console.error(err);
});
Update Issue
This feature allows you to update an existing issue in JIRA. The code sample demonstrates how to configure the jira-client and update the summary and description of an issue using its key.
const JiraClient = require('jira-client');
const jira = new JiraClient({
protocol: 'https',
host: 'your-jira-host.atlassian.net',
username: 'your-username',
password: 'your-password',
apiVersion: '2',
strictSSL: true
});
jira.updateIssue('TEST-1', {
fields: {
summary: 'Updated summary',
description: 'Updated description'
}
}).then(issue => {
console.log(`Updated issue: ${issue.key}`);
}).catch(err => {
console.error(err);
});
Search Issues
This feature allows you to search for issues in JIRA using JQL (JIRA Query Language). The code sample shows how to configure the jira-client and perform a search for issues in a specific project with a specific status.
const JiraClient = require('jira-client');
const jira = new JiraClient({
protocol: 'https',
host: 'your-jira-host.atlassian.net',
username: 'your-username',
password: 'your-password',
apiVersion: '2',
strictSSL: true
});
jira.searchJira('project = TEST AND status = "To Do"').then(result => {
console.log('Search results:', result.issues);
}).catch(err => {
console.error(err);
});
The jira-connector package is another Node.js wrapper for the JIRA REST API. It provides similar functionalities to jira-client, such as creating, updating, and searching for issues. However, jira-connector offers a more modular approach, allowing developers to include only the parts of the API they need.
The atlassian-jira package is a comprehensive library for interacting with JIRA's REST API. It supports a wide range of JIRA functionalities, including issue management, project management, and user management. Compared to jira-client, atlassian-jira offers more extensive documentation and examples, making it easier for developers to get started.
A node.js module, which provides an object oriented wrapper for the Jira Rest API.
Install with the node package manager npm:
$ npm install jira-client
// With ES5
var JiraApi = require('jira-client');
// With ES6
import JiraApi from 'jira-client';
// Initialize
var jira = new JiraApi({
protocol: 'https',
host: 'jira.somehost.com',
username: 'username',
password: 'password',
apiVersion: '2',
strictSSL: true
});
// ES5
// We are using an ES5 Polyfill for Promise support. Please note that if you don't explicitly
// apply a catch exceptions will get swallowed. Read up on ES6 Promises for further details.
jira.findIssue(issueNumber)
.then(function(issue) {
console.log('Status: ' + issue.fields.status.name);
})
.catch(function(err) {
console.error(err);
});
// ES6
jira.findIssue(issueNumber)
.then(issue => {
console.log(`Status: ${issue.fields.status.name}`);
})
.catch(err => {
console.error(err);
});
// ES7
async function logIssueName() {
try {
const issue = await jira.findIssue(issueNumber);
console.log(`Status: ${issue.fields.status.name}`);
} catch (err) {
console.error(err);
}
}
Can't find what you need in the readme? Check out our documentation here: https://jira-node.github.io/
FAQs
Wrapper for the JIRA API
The npm package jira-client receives a total of 127,749 weekly downloads. As such, jira-client popularity was classified as popular.
We found that jira-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.