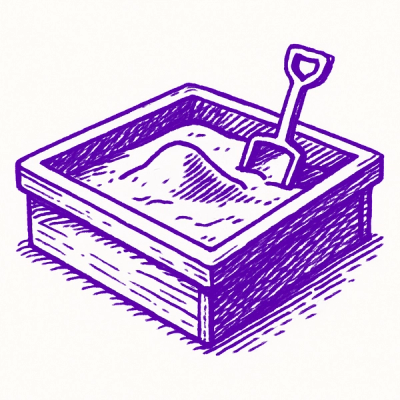
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
The js-md4 package is a JavaScript implementation of the MD4 message digest algorithm. This package allows for the hashing of strings using the MD4 algorithm, which is a cryptographic hashing function. Although MD4 is considered to be broken and vulnerable for cryptographic purposes, this package can still be used for legacy systems or non-security-critical applications where MD4 hashing is required.
MD4 Hashing of Strings
This feature allows users to hash strings using the MD4 algorithm. The code sample demonstrates how to hash the string 'string to hash' and log the result to the console.
"use strict";
const md4 = require('js-md4');
console.log(md4('string to hash'));
The md5 package is a JavaScript implementation of the MD5 message digest algorithm. Similar to js-md4, it allows for the hashing of strings but using the MD5 algorithm instead. MD5 is more widely used than MD4 and is considered to be more secure, although it also has known vulnerabilities and is not recommended for cryptographic security purposes.
The sha1 package provides a JavaScript implementation of the SHA-1 hashing algorithm. Unlike js-md4, which uses the MD4 algorithm, sha1 uses SHA-1, which is a more secure hashing algorithm but also has been found to have vulnerabilities. This package is used for hashing strings in a similar manner to js-md4 but with a different underlying algorithm.
Crypto-js is a collection of cryptography algorithms implemented in JavaScript. It offers a wide range of cryptographic functions including MD4, MD5, SHA-1, SHA-256, and more. Compared to js-md4, crypto-js provides a more comprehensive suite of cryptographic tools, making it a versatile choice for projects requiring various cryptographic functionalities.
A simple MD4 hash function for JavaScript supports UTF-8 encoding.
You can also install js-md4 by using Bower.
bower install js-md4
For node.js, you can use this command to install:
npm install js-md4
buffer
method is deprecated. This maybe confuse with Buffer in node.js. Please use arrayBuffer
instead.
You could use like this:
md4('Message to hash');
var hash = md4.create();
hash.update('Message to hash');
hash.hex();
var hash2 = md4.update('Message to hash');
hash2.update('Message2 to hash');
hash2.array();
If you use node.js, you should require the module first:
var md4 = require('js-md4');
It supports AMD:
require(['your/path/md4.js'], function (md4) {
// ...
});
md4(''); // 31d6cfe0d16ae931b73c59d7e0c089c0
md4('The quick brown fox jumps over the lazy dog'); // 1bee69a46ba811185c194762abaeae90
md4('The quick brown fox jumps over the lazy dog.'); // 2812c6c7136898c51f6f6739ad08750e
// It also supports UTF-8 encoding
md4('中文'); // 223088bf7bd45a16436b15360c5fc5a0
// It also supports byte `Array`, `Uint8Array`, `ArrayBuffer`
md4([]); // 31d6cfe0d16ae931b73c59d7e0c089c0
md4(new Uint8Array([])); // 31d6cfe0d16ae931b73c59d7e0c089c0
// Different output
md4(''); // 31d6cfe0d16ae931b73c59d7e0c089c0
md4.hex(''); // 31d6cfe0d16ae931b73c59d7e0c089c0
md4.array(''); // [49, 214, 207, 224, 209, 106, 233, 49, 183, 60, 89, 215, 224, 192, 137, 192]
md4.digest(''); // [49, 214, 207, 224, 209, 106, 233, 49, 183, 60, 89, 215, 224, 192, 137, 192]
md4.arrayBuffer(''); // ArrayBuffer
The project is released under the MIT license.
The project's website is located at https://github.com/emn178/js-md4
Author: Chen, Yi-Cyuan (emn178@gmail.com)
v0.3.2 / 2017-01-24
FAQs
A simple MD4 hash function for JavaScript supports UTF-8 encoding.
The npm package js-md4 receives a total of 2,570,820 weekly downloads. As such, js-md4 popularity was classified as popular.
We found that js-md4 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.