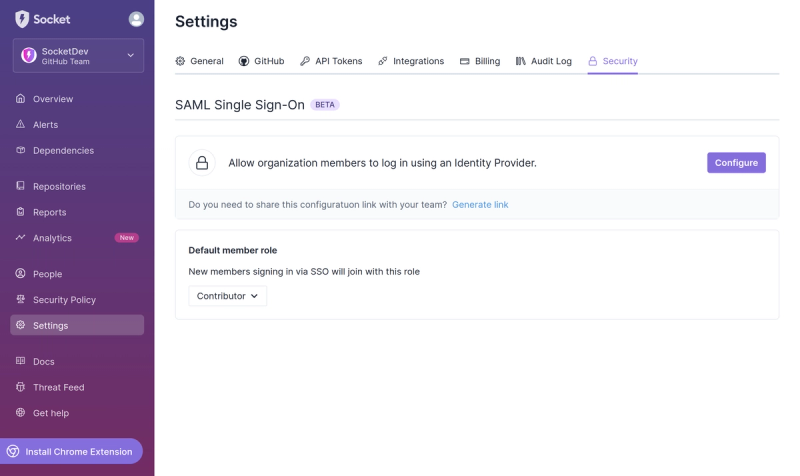
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
js-utilities-libs
Advanced tools
js-utilities-libs is a JavaScript library that provides a collection of utility functions for common programming tasks. It includes functions for string manipulation, array operations, date and time handling, mathematical calculations, object manipulation
Readme
js-utilities-libs is a JavaScript library that provides a collection of utility functions for common programming tasks. It includes functions for string manipulation, array operations, date and time handling, mathematical calculations, object manipulation, data validation, file and path operations, network utilities, data formatting, and error handling. Each function is designed to be simple, efficient, and versatile, making it easy to use in a wide range of projects.
npm install js-utilities-libs
import { trim, toCamelCase, toSnakeCase, contains } from "js-utilities-libs";
console.log(trim(" example ")); // Output: 'example'
console.log(toCamelCase("hello_world")); // Output: 'helloWorld'
console.log(toSnakeCase("helloWorld")); // Output: 'hello_world'
console.log(contains("example", "amp")); // Output: false
import {
sortArray,
filterEvenNumbers,
mapToUppercase,
sumArray,
} from "js-utilities-libs";
console.log(sortArray([3, 1, 5, 2, 4])); // Output: [1, 2, 3, 4, 5]
console.log(filterEvenNumbers([1, 2, 3, 4, 5])); // Output: [2, 4]
console.log(mapToUppercase(["hello", "world"])); // Output: ['HELLO', 'WORLD']
console.log(sumArray([1, 2, 3, 4, 5])); // Output: 15
import { formatDate, dateDiffInDays } from "js-utilities-libs";
const today = new Date();
const tomorrow = new Date(today);
tomorrow.setDate(today.getDate() + 1);
console.log(formatDate(today)); // Output: 'YYYY-MM-DD'
console.log(dateDiffInDays(today, tomorrow)); // Output: 1
import { getRandomNumber, calculateAverage } from "js-utilities-libs";
console.log(getRandomNumber(1, 10)); // Output: Random number between 1 and 10
console.log(calculateAverage([1, 2, 3, 4, 5])); // Output: 3
import { mergeObjects, cloneObject, hasProperty } from "js-utilities-libs";
const obj1 = { a: 1 };
const obj2 = { b: 2 };
console.log(mergeObjects(obj1, obj2)); // Output: { a: 1, b: 2 }
console.log(cloneObject(obj1)); // Output: { a: 1 }
console.log(hasProperty(obj1, "a")); // Output: true
import { validateEmail, validateURL } from "js-utilities-libs";
console.log(validateEmail("example@example.com")); // Output: true
console.log(validateURL("https://example.com")); // Output: true
import { readFile, writeFile } from "js-utilities-libs";
readFile("example.txt")
.then((data) => {
console.log(data); // Output: Contents of the file
})
.catch((err) => {
console.error(err);
});
writeFile("example.txt", "Hello, world!")
.then(() => {
console.log("File written successfully!");
})
.catch((err) => {
console.error(err);
});
import { fetchData } from "js-utilities-libs";
fetchData("https://jsonplaceholder.typicode.com/posts/1")
.then((data) => {
console.log(data); // Output: JSON data from the API
})
.catch((err) => {
console.error(err);
});
import { objectToJson, jsonToObject } from "js-utilities-libs";
const obj = { key: "value" };
const jsonString = objectToJson(obj);
console.log(jsonString); // Output: '{"key":"value"}'
console.log(jsonToObject(jsonString)); // Output: { key: 'value' }
import { logError, formatErrorMessage } from "js-utilities-libs";
try {
// Code that may throw an error
throw new Error("An error occurred");
} catch (error) {
logError(error); // Output: [timestamp] Error: An error occurred
console.log(formatErrorMessage(error)); // Output: An error occurred: An error occurred
}
js-utilities-libs is released under the ISC License.
1.0.3
Name : Deepak Sharma Email : uddibhardwaj08@gmail.com
FAQs
js-utilities-libs is a JavaScript library that provides a collection of utility functions for common programming tasks. It includes functions for string manipulation, array operations, date and time handling, mathematical calculations, object manipulation
The npm package js-utilities-libs receives a total of 1 weekly downloads. As such, js-utilities-libs popularity was classified as not popular.
We found that js-utilities-libs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.