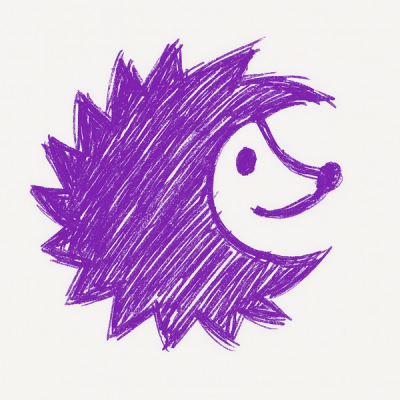
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
json-log-line
Advanced tools
This utility is designed to take JSON and plain objects and convert them into log line strings. It's compatible with any nd JSON logger.
JSON and Plain Object Support: This utility can handle both JSON and plain objects, providing flexibility in the types of data you can convert into log line strings.
Compatible with any nd JSON Logger: This utility is designed to work with any nd JSON logger, making it a versatile tool for your logging needs.
To install this utility, use the following command:
npm install json-log-line
To use this utility, simply pass your JSON or plain object to the logLineFactory
function:
const options = {
/**
* The key to use for the error object. Defaults to `err`.
*/
errorKey: "err",
/**
* include and exclude both take keys with dot notation
*/
exclude: [],
/**
* include always overrides exclude
*/
include: [],
/**
* Format functions for any given key, keys of the object are automatically included and cannot be excluded
* You can use dot notation as the object keys. The keys will print in order.
*/
format: {
some: (obj: any) => {
return obj["some-key"].toString();
},
part: (obj: any) => {
return obj["some-key"].toString();
},
of: (obj: any) => {
return obj["some-key"].toString();
},
log: (obj: any) => {
return obj["some-key"].toString();
},
},
};
export const lineFormatter = logLineFactory(yourObject);
This will return a formatter that can process strings or object output from any nd JSON logger.
example:
import { logLineFactory } from "json-log-line";
const options = {
include: ["nested.field"],
exclude: ["nested"],
format: {
part1: (value) => `[${value}]`,
part2: (value) => `[${value}]`,
"nested.field": (value) => `:${value}:\n`,
},
};
const lineFormatter = logLineFactory(options);
const log = JSON.stringify({
nested: {
other: "something",
field: "FIELD",
},
part1: "hello",
part2: "world",
some: "extra",
data: "here",
});
console.log(lineFormatter(log));
// =>
// [hello] [world] :FIELD:
// {"some":"extra","data":"here"}
Often times you will want to stream nd json logs into a function that formats each log.
Record<keyof parsed log object, () => string>
Format is an object the represents how you want to parse the log object. It will parse in natural order of the object.
A special key that contains the rest of the log object fields which were both included and not formatted by a format function.
string[]
An array of object keys to include. Overrides excludes. All keys are included by default.
string[]
An array of object keys to exclude. The keys can be nested. Can be overridden with a more deeply nested include.
FAQs
A utility for building awesome log lines from JSON
The npm package json-log-line receives a total of 2,047 weekly downloads. As such, json-log-line popularity was classified as popular.
We found that json-log-line demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.