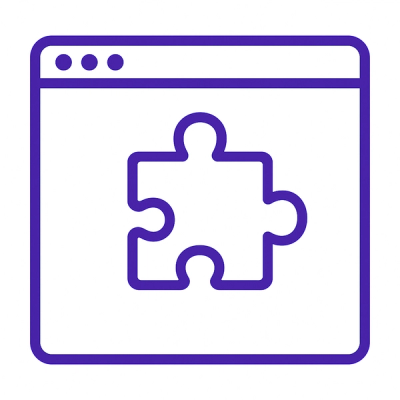
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
json-parse-safe
Advanced tools
Parse your json safely and stop writing try {} catch {}
var JSONParse = require('json-parse-safe')
JSONParse(text[, reviver])
{value, error}
var JSONParse = require('json-parse-safe');
var str = '{"cat": "Peter", "age": 1, "colors": ["white", "cyan", "black"]}';
var obj = JSONParse(str);
// obj.value should be
{
cat: 'Peter',
age: 1,
colors: ['white', 'cyan', 'black']
}
getting the exception
var bad = '{"cat": "Peter" "age": 1}';
var obj = JSONParse(str);
// obj.value should be 'undefined'
// obj.error should be an Error
console.log(obj.error);
console.log(obj.error.message);
console.log(obj.error.stack);
to run test
npm test
to run jshint
npm run jshint
to run code style
npm run code-style
to run check code coverage
npm run check-coverage
to open the code coverage report
npm run open-coverage
FAQs
Parse your json safely and stop writing try {} catch {}
The npm package json-parse-safe receives a total of 5,315 weekly downloads. As such, json-parse-safe popularity was classified as popular.
We found that json-parse-safe demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.