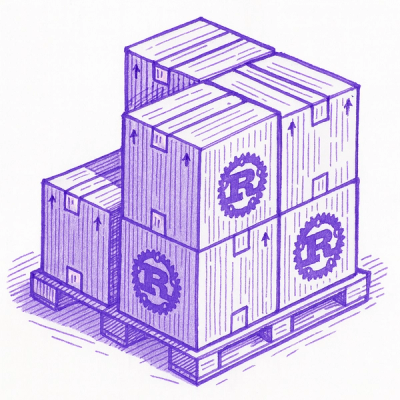
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
json-promise
Advanced tools
Parse and stringify JSON data using promise to gracefully handle success and failures if the data is invalid. See the examples below for usage instructions. This module use bluebird for Promise/A+ support.
npm install json-promise
var json = require('json-promise');
var str = [
'{"menu":{"id":"file","value":"File","popup":'
,'{"menuitem":[{"value":"New","onclick":"CreateNewDoc()"},'
,'{"value":"Open","onclick":"OpenDoc()"},{"value":"Close",'
,'"onclick":"CloseDoc()"}]}}}'
].join('');
json.parse(str)
.then(function onParse(obj) {
// do something with the data object
})
.catch(function onParseError(e) {
// the data is corrupted!
});
var json = require('json-promise');
var obj = {
"menu": {
"id": "file",
"value": "File",
"popup": {
"menuitem": [
{
"value": "New",
"onclick": "CreateNewDoc()"
},
{
"value": "Open",
"onclick": "OpenDoc()"
},
{
"value": "Close",
"onclick": "CloseDoc()"
}
]
}
}
};
json.stringify(obj)
.then(function onStringify(obj) {
// do something with the string
})
.catch(function onStringifyError(e) {
// the data is corrupted!
});
npm test
FAQs
Promise based JSON parser. Handles invalid JSON data gracefully.
The npm package json-promise receives a total of 134,441 weekly downloads. As such, json-promise popularity was classified as popular.
We found that json-promise demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.